User Input with Variables in C#
Introduction
In this tutorial, we will explore how to work with user input and variables in C#. Understanding how to accept and manipulate user input is a fundamental skill in programming, as it allows us to create interactive applications. We’ll cover the basics of reading user input, storing it in variables, and performing simple operations. Whether you’re a beginner or just brushing up on C#, this guide will help you understand the key concepts to enhance your coding skills. Let’s dive into C# user input handling and variables!
Objectives
In this tutorial, we aim to provide you with a comprehensive understanding of handling user input and variables in C#. By the end, you will have learned how to accept, store, and manipulate input from users effectively. Our focus is on helping you build practical skills through a step-by-step approach. This tutorial is designed with clear objectives to ensure you can not only understand the concepts but also practice and apply them in real-world scenarios.
- Understand User Input and Variables in C#
- Gain a solid understanding of how user input is captured and stored in variables in C# applications. You will also learn about the different types of variables and how they work in C# programming.
- Learn to Capture User Input
- Learn how to use methods like Console.ReadLine() to read input from users and how to store this input in variables for further processing.
- Practice Using Variables with User Input
- Practice handling different data types such as strings, integers, and floats when working with user input. You’ll gain hands-on experience in writing code to perform operations on these variables.
- Apply Input Handling in Real-World Scenarios
- Apply the concepts you’ve learned to create interactive C# programs that can perform tasks based on user input, such as calculations, decision-making, or text manipulation.
By mastering these objectives, you’ll be equipped to write interactive and dynamic C# applications.
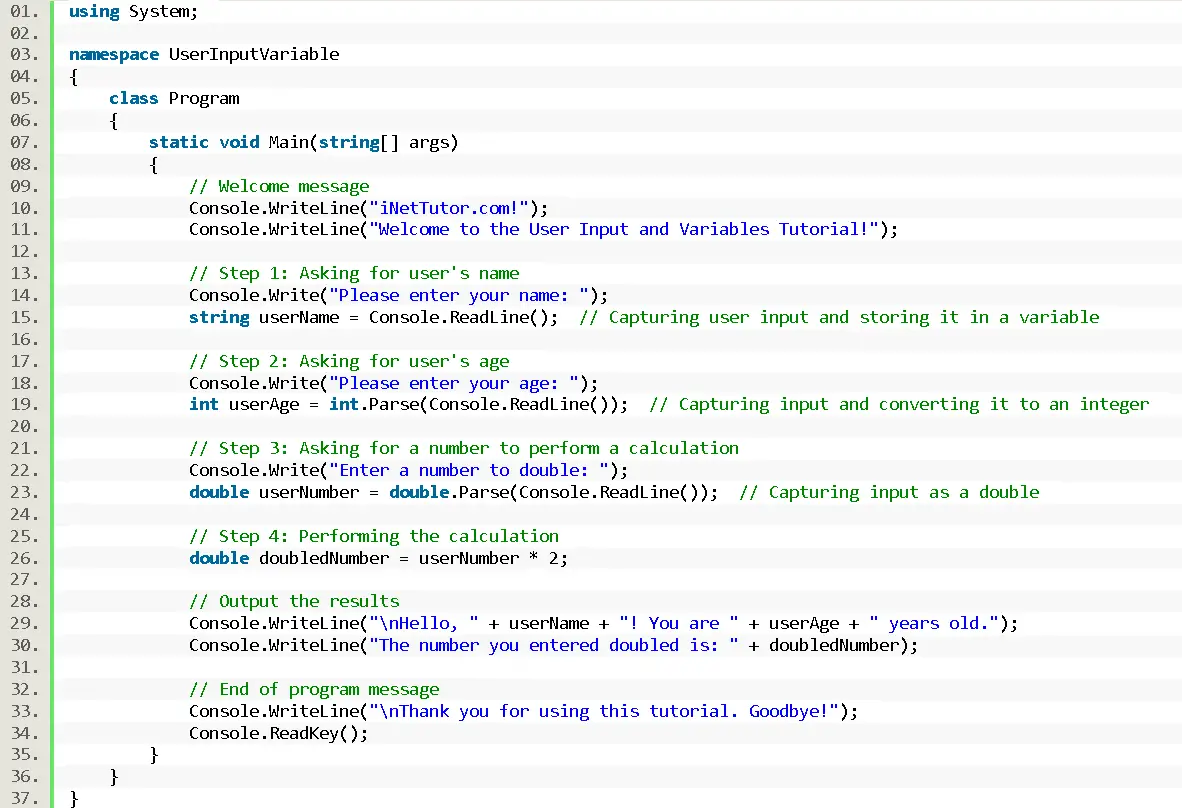
Source code example
using System; namespace UserInputVariable { class Program { static void Main(string[] args) { // Welcome message Console.WriteLine("iNetTutor.com!"); Console.WriteLine("Welcome to the User Input and Variables Tutorial!"); // Step 1: Asking for user's name Console.Write("Please enter your name: "); string userName = Console.ReadLine(); // Capturing user input and storing it in a variable // Step 2: Asking for user's age Console.Write("Please enter your age: "); int userAge = int.Parse(Console.ReadLine()); // Capturing input and converting it to an integer // Step 3: Asking for a number to perform a calculation Console.Write("Enter a number to double: "); double userNumber = double.Parse(Console.ReadLine()); // Capturing input as a double // Step 4: Performing the calculation double doubledNumber = userNumber * 2; // Output the results Console.WriteLine("\nHello, " + userName + "! You are " + userAge + " years old."); Console.WriteLine("The number you entered doubled is: " + doubledNumber); // End of program message Console.WriteLine("\nThank you for using this tutorial. Goodbye!"); Console.ReadKey(); } } }
Explanation
The provided C# code demonstrates the fundamentals of user input, variables, and basic calculations. Here’s a breakdown of each section:
- Using System:
This line tells the compiler to include the System namespace, which contains essential classes like Console for interacting with the user through the console window.
- Namespace UserInputVariable:
This line defines a namespace named UserInputVariable. Namespaces help organize code by grouping related elements together. It prevents conflicts with similarly named elements in other parts of your project.
- Class Program:
This line defines a class named Program. Classes are blueprints for creating objects that encapsulate data (variables) and functionality (methods). In this example, the Program class holds the main logic of the code.
- Static void Main(string[] args):
- static: This keyword indicates that the Main method can be called directly without creating an instance of the Program class.
- void: This keyword specifies that the Main method doesn’t return any value after its execution.
- string[] args: This parameter is an array of strings that can be used to pass arguments from the command line when running the program (not used in this specific code). This is the entry point of the program, where execution begins.
- Welcome Message:
- Console.WriteLine(“iNetTutor.com!”); and Console.WriteLine(“Welcome to the User Input and Variables Tutorial!”); These lines use the Console.WriteLine method to display a welcome message and the tutorial title on the console window.
- Step 1: Asking for User’s Name:
- Console.Write(“Please enter your name: “); This line displays a prompt asking the user to enter their name using Console.Write. The Write method prints the message without adding a newline character at the end.
- string userName = Console.ReadLine(); This line captures the user’s input for their name using Console.ReadLine. It reads the entire line entered by the user (including spaces) and stores it in the userName variable of type string. This variable will hold the user’s name.
- Step 2: Asking for User’s Age:
- Console.Write(“Please enter your age: “); This line displays a prompt asking for the user’s age.
- int userAge = int.Parse(Console.ReadLine()); This line retrieves the user’s input for their age using Console.ReadLine, which reads it as a string. However, age should be an integer (whole number). So, int.Parse(Console.ReadLine()) converts the string input to an integer and stores it in the userAge variable of type int. If the user enters non-numeric characters, this conversion might fail and throw an exception (covered in more advanced courses).
- Step 3: Asking for a Number to Perform a Calculation:
- Console.Write(“Enter a number to double: “); This line displays a prompt asking the user to enter a number to be doubled.
- double userNumber = double.Parse(Console.ReadLine()); This line captures the user’s input as a string and converts it to a double (floating-point number) using double.Parse(Console.ReadLine()). The result is stored in the userNumber variable of type double.
- Step 4: Performing the Calculation:
- double doubledNumber = userNumber * 2; This line calculates the double of the user’s entered number using multiplication and stores the result in the doubledNumber variable.
- Output the Results:
- Console.WriteLine(“\nHello, ” + userName + “! You are ” + userAge + ” years old.”); This line uses string concatenation (+) to create a personalized greeting message that combines the user’s name and age. The \n at the beginning adds a newline character for better formatting.
- Console.WriteLine(“The number you entered doubled is: ” + doubledNumber); This line displays the result of the calculation, showing the doubled value of the user’s input.
- End of Program Message:
- Console.WriteLine(“\nThank you for using this tutorial. Goodbye!”); This line displays a farewell message for the user.
- Console.ReadKey(); This line pauses the console window until the user presses any key. This prevents the window from closing immediately after the program finishes and allows the user to review the output.
Output
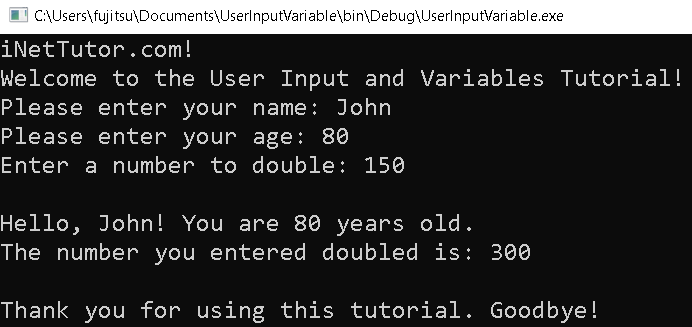
Summary
In this lesson, we explored how to handle user input and work with variables in C#. We learned to capture input from the user using Console.ReadLine(), store it in variables of different data types such as string, int, and double, and convert string input to numeric types using int.Parse() and double.Parse() for calculations. We also demonstrated basic arithmetic by doubling a user-inputted number and displaying personalized messages. This foundational understanding of user input and variables is essential for creating interactive C# applications.
Exercises and Assessment
The provided C# code demonstrates basic input/output operations, variable usage, and simple calculations. To enhance the code’s functionality, readability, and robustness, we can introduce exercises that focus on error handling, data validation, and code organization.
Exercises
Exercise 1: Error Handling
Objective: To handle potential exceptions that might occur during user input and conversion.
Task:
- Modify the code to use a try-catch block to handle the potential FormatException that might arise when parsing the user’s input for age and number.
- Provide informative error messages to the user if an exception occurs.
Exercise 2: Data Validation
Objective: To validate user input to ensure it’s within a reasonable range.
Task:
- Add code to check if the user’s age is greater than 0 and less than 120.
- If the age is outside this range, display an error message and prompt the user to enter a valid age.
Exercise 3: Code Organization
Objective: To improve code readability and maintainability through better organization.
Task:
- Create separate methods for each distinct task: getting user input, validating input, performing calculations, and displaying output.
- Use meaningful variable names and comments to explain the purpose of different code sections.
Assessment
The assessment will evaluate the student’s ability to:
- Implement error handling using try-catch blocks.
- Validate user input effectively.
- Organize code into well-structured methods.
- Use meaningful variable names and comments.
- Produce code that is readable, maintainable, and robust.
Lab Exam
The lab exam will require students to:
- Write C# code to solve a similar problem, incorporating the concepts learned in the exercises.
- Demonstrate their understanding of error handling, data validation, and code organization.
- Explain their code and reasoning behind their approach.
Example Lab Exam Problem:
Write a C# program that calculates the area of a rectangle based on user-provided length and width. Implement error handling to catch invalid input (e.g., negative values). Validate the input to ensure both length and width are positive. Organize your code into separate methods for input, validation, calculation, and output.
Quiz
1. What method is used to read user input in C#?
A) Console.Write()
B) Console.ReadLine()
C) Console.Read()
D) Console.WriteLine()
2. Which of the following best describes what the int.Parse() method does in C#?
A) Converts a string to an integer.
B) Reads an integer from the console.
C) Displays an integer as output.
D) Calculates the sum of two integers.
3. Given the following code, what will be the output if the user inputs the number 4?
Console.Write("Enter a number: "); int num = int.Parse(Console.ReadLine()); Console.WriteLine(num * 3);
A) 3
B) 7
C) 12
D) 9
4. In the code below, which part is responsible for preventing invalid inputs when a user enters non-numeric data for age?
int userAge; while (!int.TryParse(Console.ReadLine(), out userAge)) { Console.WriteLine("Invalid input. Please enter a valid number for age."); }
A) int userAge;
B) while (!int.TryParse(…))
C) Console.ReadLine()
D) out userAge
5. Which of the following is the most efficient way to handle multiple incorrect user inputs in a C# program?
A) Use a try-catch block for every input.
B) Use if-else conditions to check input validity.
C) Use a while loop with TryParse() for continuous validation.
D) Stop the program if an invalid input is detected.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.