Structures in C#
Introduction
In C#, structures (structs) are value types that allow you to group related variables under a single unit. They are useful when you need a lightweight alternative to classes for storing small data objects. Unlike classes, structs are stored on the stack, making them more memory-efficient in certain scenarios.
This guide will walk you through the basics of C# structs, their advantages, and when to use them. Whether you’re a beginner or an experienced developer, understanding structs can help you write optimized and well-structured code.
Objectives
When diving into the world of C# programming, understanding structures is essential for writing efficient and optimized code. Structures, or structs, are a fundamental concept that allows developers to create custom value types, offering performance benefits in specific scenarios. To master this topic, we’ve outlined four key objectives: understand, learn, practice, and apply. These objectives will guide you through grasping the core concepts, exploring their functionality, honing your skills through hands-on exercises, and ultimately applying structures in real-world programming scenarios. By the end of this journey, you’ll have a solid foundation in using structures effectively in your C# projects.
- Understand: Gain a clear understanding of what structures are, how they differ from classes, and their role in C# programming.
- Learn: Explore the syntax, features, and best practices for defining and using structures in C#.
- Practice: Reinforce your knowledge by working on practical examples and exercises to build confidence in using structures.
- Apply: Learn how to implement structures in real-world applications, ensuring optimal performance and memory efficiency.
Source code example
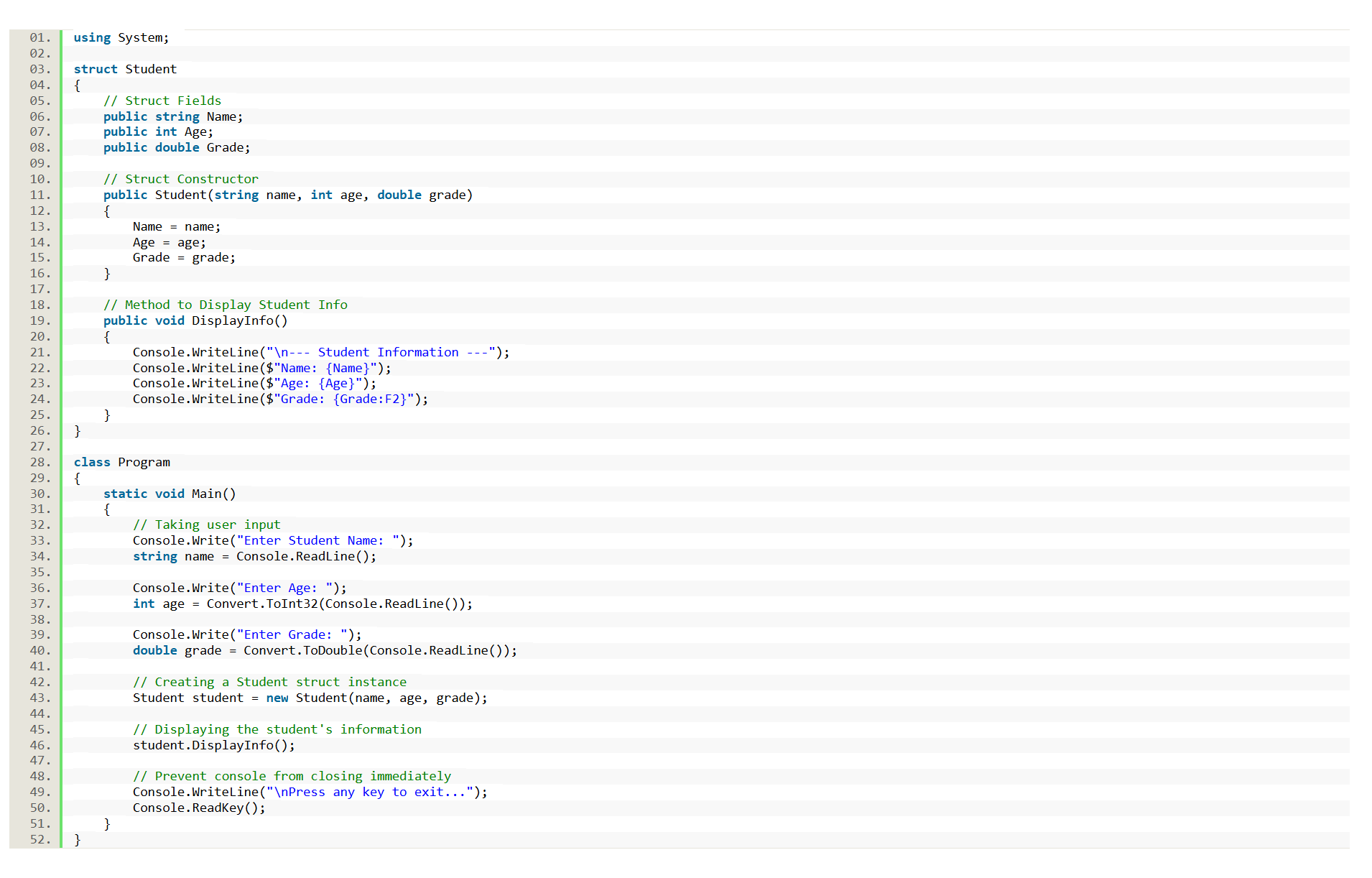
Here’s a beginner-friendly C# struct example that includes user interaction. This simple program defines a Student struct, takes input from the user, and displays the details.
using System; struct Student { // Struct Fields public string Name; public int Age; public double Grade; // Struct Constructor public Student(string name, int age, double grade) { Name = name; Age = age; Grade = grade; } // Method to Display Student Info public void DisplayInfo() { Console.WriteLine("\n--- Student Information ---"); Console.WriteLine($"Name: {Name}"); Console.WriteLine($"Age: {Age}"); Console.WriteLine($"Grade: {Grade:F2}"); } } class Program { static void Main() { // Taking user input Console.Write("Enter Student Name: "); string name = Console.ReadLine(); Console.Write("Enter Age: "); int age = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter Grade: "); double grade = Convert.ToDouble(Console.ReadLine()); // Creating a Student struct instance Student student = new Student(name, age, grade); // Displaying the student's information student.DisplayInfo(); // Prevent console from closing immediately Console.WriteLine("\nPress any key to exit..."); Console.ReadKey(); } }
Explanation
This C# program demonstrates the use of a structure (struct) to store and display information about a Student. It includes user interaction, allowing the user to input details about a student, and then displays the information in a structured format.
Key Components of the Code
- Structure Definition (Student):
- The Student structure is defined with three fields:
- Name (string): Stores the student’s name.
- Age (int): Stores the student’s age.
- Grade (double): Stores the student’s grade.
- A constructor is defined to initialize the fields when a Student instance is created.
- A method DisplayInfo() is included to print the student’s details in a readable format.
- The Student structure is defined with three fields:
- User Interaction:
- The program prompts the user to input the student’s name, age, and grade.
- These inputs are stored in variables (name, age, and grade).
- Creating a Struct Instance:
- A Student instance is created using the constructor, passing the user-provided values (name, age, and grade) as arguments.
- Displaying Information:
- The DisplayInfo() method is called on the Student instance to print the student’s details.
- Preventing Console Closure:
- The program waits for the user to press any key before closing the console window, ensuring the output is visible.
How It Works
- The program starts by asking the user to enter the student’s name, age, and grade.
- These inputs are stored in variables and passed to the Student constructor to create a new instance.
- The DisplayInfo() method is called to print the student’s details in a formatted way.
- The program waits for a key press before exiting, allowing the user to view the output.
Why This Code is Useful
- It introduces the concept of structures in C# and demonstrates how to define and use them.
- It includes user interaction, making it practical and engaging for beginners.
- It shows how to create a constructor and methods within a structure.
- It provides a clear example of how to organize and display data effectively.
Example Output
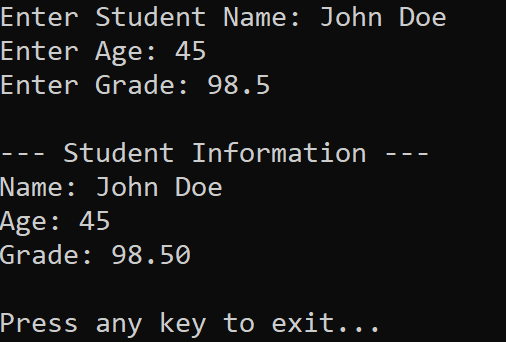
Why Use a Struct Instead of a Class?
Lightweight – Structs are stored on the stack, making them faster in scenarios where large numbers of small data objects are used.
Value Type – Unlike classes, structs don’t require garbage collection since they are automatically removed when they go out of scope.
Encapsulation – The struct neatly groups related properties and behavior together.
Summary
This lesson introduces the concept of structures (struct) in C#, which are value types used to group related data members under a single name. Using a practical example of a Student structure, the lesson demonstrates how to define a structure with fields (such as Name, Age, and Grade), create a constructor to initialize these fields, and add methods (like DisplayInfo()) to display the data. The program also includes user interaction, allowing the user to input student details and view the formatted output. By the end of the lesson, beginners will understand how to create, use, and apply structures in C# for organizing and managing data efficiently.
Quiz
- What is the primary characteristic of a struct in C#?
A. It is a reference type stored on the heap
B. It is a value type stored on the stack
C. It supports inheritance like a class
D. It requires explicit memory management - Which of the following statements best explains the difference between a struct and a class in C#?
A. Structs are used for larger, more complex objects, while classes are for small, simple data types.
B. Structs and classes function the same way, but structs allow multiple inheritance.
C. Structs are value types stored on the stack, while classes are reference types stored on the heap.
D. Structs support dynamic memory allocation, while classes do not. - Which of the following is the correct way to declare and initialize a struct in C#?
A. Student s = new Student(); s.Name = “John”;
B. Student s = new Student(“John”);
C. Student s = Student();
D. Student s = struct Student { Name = “John” }; - Why would you use a struct instead of a class in performance-critical applications?
A. Structs are reference types, which makes them faster.
B. Structs are allocated on the stack, avoiding the overhead of garbage collection.
C. Structs allow dynamic memory allocation, which improves performance.
D. Structs consume more memory, making them ideal for high-performance computing. - Which scenario best justifies the use of a struct over a class in C#?
A. You need to store large amounts of data and frequently modify it.
B. You need to create a lightweight data type that represents a simple object with a few fields.
C. You need to implement object-oriented features like polymorphism and inheritance.
D. You want to share a single instance of an object across multiple parts of your program.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.