Strings in C#
Introduction
Table of Contents
What is a String?
In programming, a string is a sequence of characters used to represent text. Whether it’s a single word, a sentence, or an entire document, strings are essential for handling and manipulating textual data. In C#, a string is an object of the System.String class, which provides a wide range of methods and properties to work with text efficiently.
Importance of Strings in Programming
Strings are one of the most fundamental data types in programming. They are used in almost every application, from simple console outputs to complex web applications. Here’s why strings are so important:
- User Interaction: Strings are used to display messages, take user input, and communicate with users.
- Data Processing: They are crucial for parsing, formatting, and manipulating data, such as reading files, processing JSON, or handling URLs.
- Text Manipulation: Strings enable operations like searching, replacing, splitting, and concatenating text, which are essential for tasks like data validation or text analysis.
How Strings are Represented in C#
In C#, strings are represented as a sequence of Unicode characters. Each character in a string is stored as a 16-bit Unicode value, allowing C# to support a wide range of characters from different languages and symbols.
For example:
string greeting = “Hello, World!”;
Here, the variable greeting holds the string “Hello, World!”, which is a sequence of characters. Internally, C# treats this string as an immutable object, meaning once a string is created, it cannot be changed. Any operation that appears to modify a string actually creates a new string object.
Strings in C# are also reference types, meaning they are stored in the heap memory, and variables hold references to these objects. This design allows for efficient memory management and powerful string manipulation capabilities.
Objectives
Strings are a cornerstone of programming, and mastering them is essential for building robust and efficient applications. In this lesson, we will focus on four key objectives to help you gain a solid understanding of strings in C#. By the end of this session, you will understand the fundamentals of strings, learn how to use them effectively, practice through hands-on examples, and apply your knowledge to solve real-world problems. Let’s dive into these objectives in detail.
- Understand: Grasp the Fundamentals of Strings
- Gain a clear understanding of what strings are and how they are represented in C#.
- Learn about string immutability and why it matters in programming.
- Explore the role of strings in handling and manipulating textual data.
- Learn: Explore String Operations and Methods
- Discover the various ways to declare, initialize, and manipulate strings in C#.
- Learn about common string operations such as concatenation, interpolation, and comparison.
- Understand how to use built-in string methods like Substring(), Replace(), Trim(), and more.
- Practice: Hands-On Coding Exercises
- Practice writing code to perform basic and advanced string manipulations.
- Work through examples like reversing a string, counting words, and checking for palindromes.
- Experiment with StringBuilder for efficient string handling in scenarios involving heavy text manipulation.
- Apply: Solve Real-World Problems
- Apply your knowledge to solve practical problems, such as formatting user input, parsing data, or processing text files.
- Build a small project or feature that leverages string manipulation, like a simple text analyzer or a password validator.
- Develop the confidence to use strings effectively in your own applications.
Source code example
Here’s a beginner-friendly source code for your lesson on Strings in C#. This code covers the basics of strings, including declaration, initialization, common operations, and some practical examples. Each section is explained with comments to make it easy to follow.
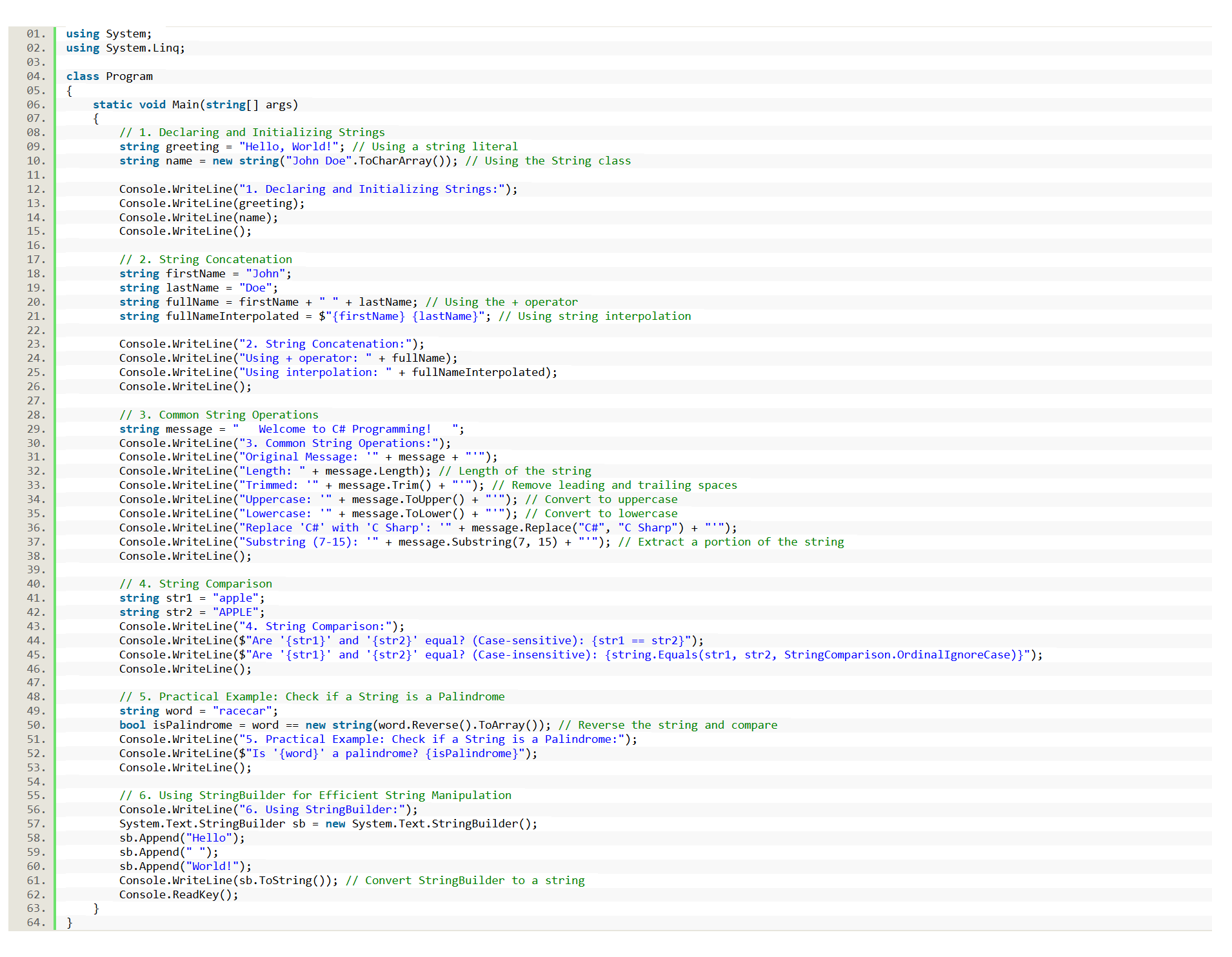
Explanation
- Declaring and Initializing Strings:
- Shows how to create strings using string literals and the String class.
- String Concatenation:
- Demonstrates two ways to combine strings: using the + operator and string interpolation.
- Common String Operations:
- Introduces methods like Trim(), ToUpper(), ToLower(), Replace(), and Substring().
- String Comparison:
- Compares strings using == and String.Equals() with case-sensitive and case-insensitive options.
- Practical Example:
- Checks if a string is a palindrome by reversing it and comparing it to the original.
- StringBuilder:
- Introduces StringBuilder for efficient string manipulation, especially when dealing with large or frequently changing text.
Example Output
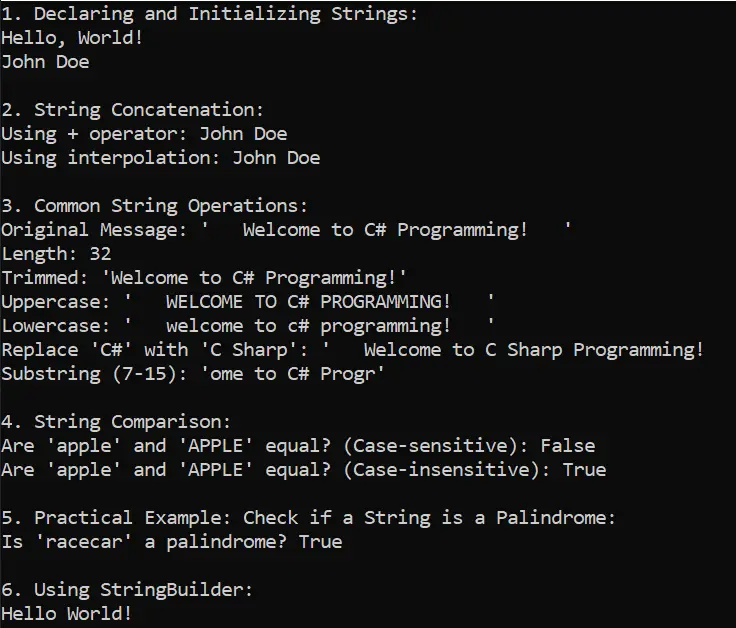
Summary
In this lesson, we explored the fundamentals of strings in C#, starting with their definition as sequences of characters used to represent text. We learned how to declare and initialize strings, perform common operations like concatenation, trimming, and replacing, and compared strings using both case-sensitive and case-insensitive methods. Practical examples, such as checking for palindromes, helped solidify our understanding, while an introduction to StringBuilder highlighted efficient ways to handle large or dynamic text. By the end of the lesson, we gained the skills to manipulate, analyze, and apply strings effectively in real-world programming scenarios.
Exercises and Assessment
To reinforce your understanding of strings in C# and improve your coding skills, this lab exam provides a series of hands-on exercises. These tasks are designed to challenge you to apply what you’ve learned about string manipulation, from basic operations to more advanced techniques. By completing these exercises, you’ll gain confidence in working with strings and develop the ability to write efficient and clean code. Let’s dive into the tasks and take your skills to the next level!
Exercise 1: Reverse a String
Write a program that takes a string input from the user and reverses it. For example, if the input is “hello”, the output should be “olleh”.
Hint: Use a loop or the Array.Reverse() method.
Exercise 2: Count the Number of Words
Create a program that counts the number of words in a given string. Assume words are separated by spaces. For example, the string “C# is fun” should return 3.
Hint: Use the Split() method to divide the string into an array of words.
QUIZ – Multiple Choice Questions
- What is the primary purpose of a string in C#?
A. To store and manipulate text data
B. To perform mathematical calculations
C. To store boolean values
D. To execute system commands - Which of the following best describes how strings are stored in C#?
A. Strings are stored as arrays of integers
B. Strings are immutable sequences of characters
C. Strings are dynamically allocated and can be modified directly in memory
D. Strings are only available as numeric representations - What will be the output of the following C# code?
string message = ” Hello, C# “;
Console.WriteLine(message.Trim());
A. ” Hello, C# ”
B. “Hello, C#”
C. “Hello, C# ”
D. ” Hello, C#”
- Which method would be most appropriate to extract the word “C#” from the following string?
string sentence = “Learning C# is fun!”;
A. sentence.Substring(9, 2);
B. sentence.Remove(9, 2);
C. sentence.Split(‘ ‘)[0];
D. sentence.ToUpper();
- Which of the following statements best explains why StringBuilder is preferred over string for frequent modifications?
A. StringBuilder allows modification without creating new string instances, improving performance
B. StringBuilder prevents memory leaks by automatically deallocating unused memory
C. StringBuilder can store numerical values directly without conversion
D. StringBuilder has built-in methods for mathematical operations
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.