String concatenation and interpolation in C#
Welcome to our next lesson in the exciting world of C# programming! In this blog post, we will delve into the fascinating concepts of string concatenation and interpolation. As a C# developer, you’ll often find yourself working with strings, and having a strong grasp of these techniques will elevate your coding prowess to new heights.
Introduction
Table of Contents
Strings are an essential part of programming, allowing developers to manipulate and display text efficiently. In C#, string concatenation and interpolation are two powerful techniques for combining strings and inserting variable values into text. Concatenation involves joining multiple strings end-to-end, often using the + operator, while interpolation, introduced in C# 6.0, provides a more readable and convenient way to embed expressions within string literals using the $ symbol. Understanding these methods is crucial for tasks such as generating dynamic messages, formatting output, and building complex strings in a clean and efficient manner. This lesson will explore the fundamentals of string concatenation and interpolation, demonstrating their practical applications and best practices in C#.
Objectives
In this lesson, our main objectives are to help you understand, learn, practice, and apply the concepts of string concatenation and interpolation in C#. By the end of this lesson, you will have a solid understanding of how these techniques work, the syntax involved, and their practical applications in C# programming. Through a combination of explanations, examples, and hands-on exercises, we aim to equip you with the necessary knowledge and skills to confidently utilize string concatenation and interpolation in your coding projects.
By the end of this tutorial, learners will be able to:
- Understand the concepts of string concatenation and interpolation.
- Learn the syntax and techniques for both concatenation and interpolation.
- Practice building various string combinations through hands-on exercises.
- Apply string manipulation skills to create real-world C# applications.
Source code example
using System; class Program { static void Main() { Console.WriteLine("iNetTutor.com - String Concatenation and Interpolation"); string name = "John"; int age = 25; // String concatenation string greeting = "Hello, " + name + "! You are " + age + " years old."; Console.WriteLine(greeting); // String interpolation string message = $"Hello, {name}! You are {age} years old."; Console.WriteLine(message); Console.ReadKey(); } }
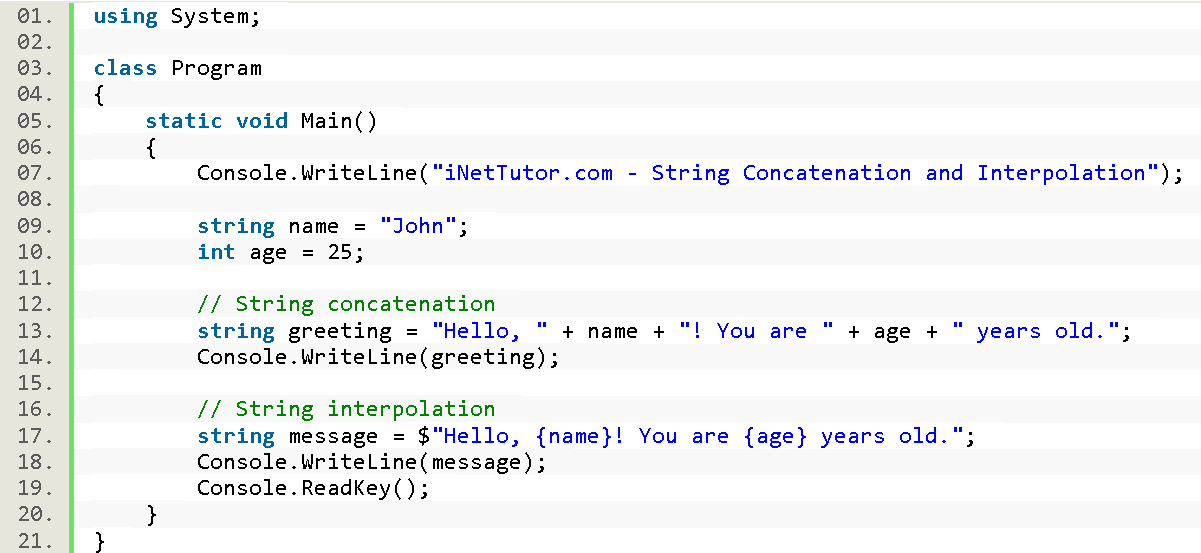
Explanation
The provided code demonstrates the usage of string concatenation and interpolation in C#. Here’s a breakdown of the code:
- The using System; statement at the beginning of the code imports the System namespace, which contains fundamental classes and base classes used in C# programming.
- The class Program defines a class named “Program” that contains the program’s entry point, the Main method.
- Inside the Main method, the code starts by printing a message to the console using Console.WriteLine(). This message serves as an introduction to the topic of string concatenation and interpolation.
- The code then declares a string variable called name and initializes it with the value “John”. It also declares an integer variable called age and sets it to 25.
- Next, the code demonstrates string concatenation by creating a new string called greeting. The + operator is used to concatenate multiple strings and variables together, resulting in a greeting that includes the name and age values.
- After that, the code showcases string interpolation by creating a new string called message. The $ symbol is used to indicate string interpolation, and expressions and variables are enclosed in curly braces {} within the string. This allows the name and age values to be directly embedded in the string.
- Finally, the code uses Console.WriteLine() to print both the greeting and message strings to the console.
Output

Summary
This lesson introduces the concepts of string concatenation and interpolation in C#. String concatenation involves combining strings and variables using the + operator, allowing for the construction of complex strings from simpler ones. String interpolation, on the other hand, uses the $ symbol followed by curly braces {} to embed expressions directly within a string literal, offering a more readable and concise way to build strings. Through practical examples, learners understand how to effectively create dynamic strings using both methods, enhancing their ability to manipulate and present text data in C# applications.
Exercises and Assessment
To solidify your understanding of string concatenation and interpolation in C#, it’s important to practice by implementing these concepts in various scenarios. The following exercises, assessment questions, and lab exam will help reinforce your skills and ensure you can effectively manipulate strings using these techniques.
Exercises
- Basic Concatenation and Interpolation
- Create a program that takes a user’s first name and last name as input and then displays a full greeting message using both concatenation and interpolation.
- Dynamic String Construction
- Write a program that prompts the user to enter their favorite color and favorite food. Display a message using concatenation that says, “Your favorite color is [color] and your favorite food is [food].” Repeat the same message using interpolation.
- Math Operation Message
- Write a program that takes two numbers as input from the user and calculates their sum. Display a message using concatenation and interpolation that states, “The sum of [number1] and [number2] is [sum].”
Quiz
- Identify the Correct Concatenation
- Which of the following correctly concatenates the strings “Hello” and “World”?
- a) “Hello World”
- b) Hello + World
- c) “Hello” + ” World”
- d) $”Hello World”
- Benefits of Interpolation
- What is one advantage of using string interpolation over string concatenation?
- a) It is faster to type.
- b) It allows for embedding variables directly in the string.
- c) It requires less memory.
- d) It uses fewer characters.
- String Interpolation Syntax
- Which of the following is the correct syntax for string interpolation in C#?
- a) “Hello, {name}!”
- b) $”Hello, {name}!”
- c) $”Hello, name!”
- d) “Hello, + name + !”
- Invalid Interpolation Example
- Identify the incorrect usage of string interpolation:
- a) $”The result is: {result}”
- b) $”User: {user}, Score: {score}”
- c) $”Sum: {a + b}”
- d) $”Age: ” + {age}
- Understanding: Practical Application
- Which of the following statements best describes string interpolation?
- a) It concatenates strings using the + operator.
- b) It formats strings using placeholders.
- c) It allows embedding expressions inside strings prefixed with $.
- d) It uses StringBuilder for efficient string manipulation.
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.