Stacks in C#
Introduction
In computer science, a stack is a fundamental data structure that follows the LIFO (Last-In, First-Out) principle. Imagine a stack of plates: you can only add a new plate to the top, and you can only remove the topmost plate. Stacks are used in various applications, from managing function calls in programming to implementing undo/redo functionality. This tutorial will guide you through the basics of stacks in C#, providing a clear explanation and a practical code example.
Objectives
Stacks are an essential data structure in programming, widely used in algorithms such as expression evaluation, backtracking, and function calls. This tutorial aims to help you understand the fundamentals of stacks, learn how to implement them in C#, practice writing stack-based programs, and apply your knowledge to solve real-world problems. By the end of this tutorial, you will be able to:
- Understand the stack data structure and its Last In, First Out (LIFO) principle.
- Learn how to implement stack operations (Push, Pop, Peek, and Display) in C#.
- Practice coding exercises to reinforce your understanding of stacks.
- Apply stack concepts to solve programming challenges such as string reversal, expression evaluation, and undo-redo functionality.
Source code example
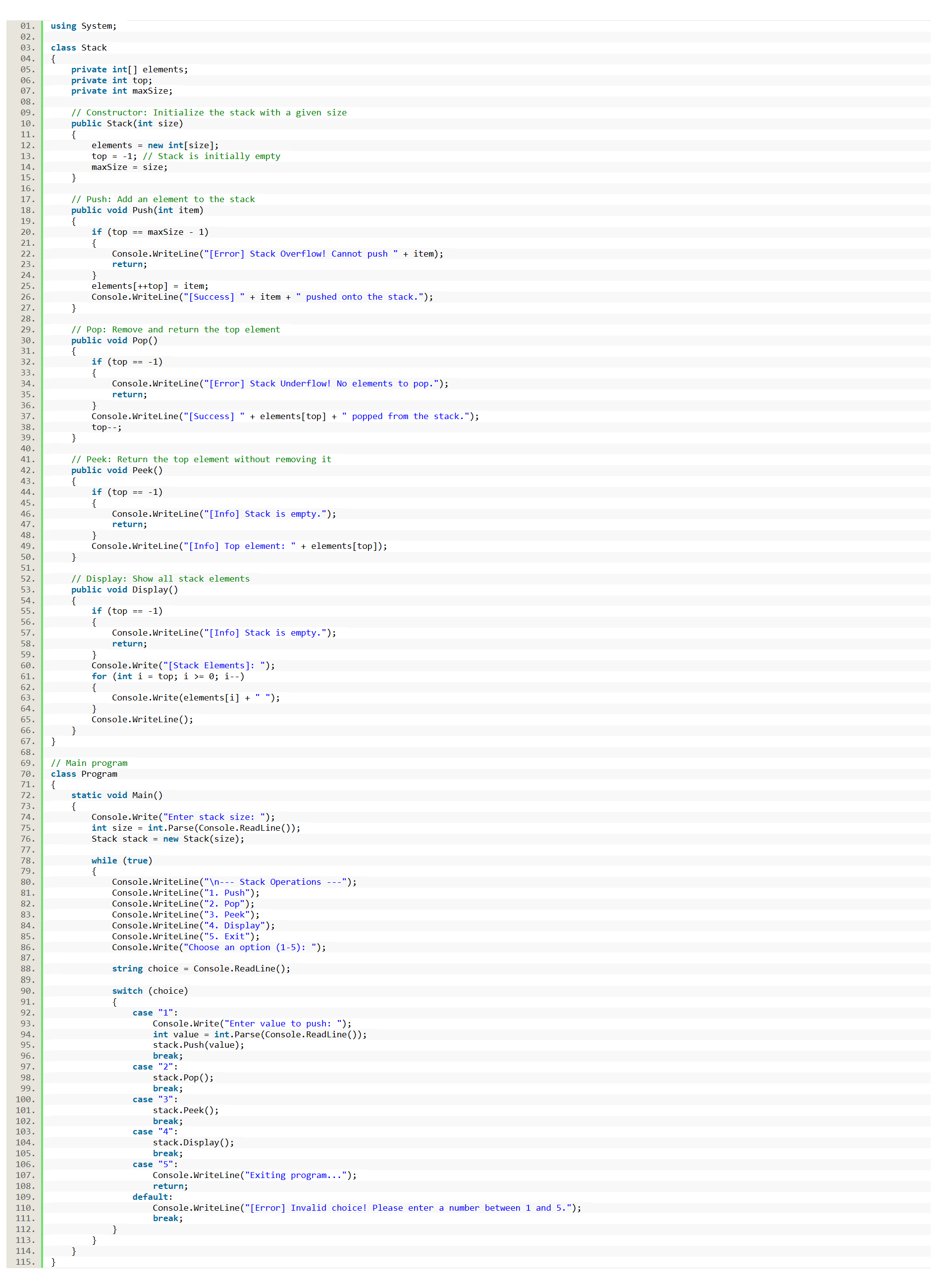
Explanation
Let’s break down this C# code that implements a stack data structure:
- The Stack Class:
- private int[] elements;: This declares a private array named elements of type int. This array will store the actual elements of the stack. The private keyword means this array can only be accessed within the Stack class itself (encapsulation).
- private int top;: This integer variable top keeps track of the index of the topmost element in the stack. Crucially, top is initialized to -1, signifying that the stack is initially empty.
- private int maxSize;: This integer variable maxSize stores the maximum number of elements the stack can hold. This is determined when the stack is created.
- public Stack(int size) (Constructor): This is a special method called a constructor. It’s executed when you create a new Stack object. It takes an integer size as an argument, which determines the maximum size of the stack. Inside the constructor:
- elements = new int[size];: It creates the elements array with the specified size.
- top = -1;: It initializes top to -1, indicating an empty stack.
- maxSize = size;: It sets maxSize to the provided size.
- public void Push(int item): This method adds an element (item) to the top of the stack.
- if (top == maxSize – 1): It checks for stack overflow. If top is already at the last possible index of the array (maxSize – 1), the stack is full, and an error message is printed.
- elements[++top] = item;: If there’s space, this is the core of the Push operation. ++top increments the top before using its value. So, top is incremented to point to the next available slot, and then the item is placed in that slot.
- A success message is printed.
- public void Pop(): This method removes and returns the top element from the stack.
- if (top == -1): It checks for stack underflow. If top is -1, the stack is empty, and an error message is printed.
- Console.WriteLine(“[Success] ” + elements[top] + ” popped from the stack.”);: The value of the element at the current top is printed as being popped.
- top–;: top is decremented, effectively removing the element from the stack (the value is still in the array, but it’s no longer considered part of the stack).
- public void Peek(): This method returns the top element without removing it.
- if (top == -1): Checks for an empty stack.
- Prints the value of the element at elements[top].
- public void Display(): This method prints all the elements in the stack, starting from the top.
- if (top == -1): Checks for an empty stack.
- It iterates from top down to 0, printing each element.
- The Program Class and Main Method:
- static void Main(): This is the entry point of the program.
- The program first prompts the user to enter the desired size of the stack.
- A Stack object is created with the given size: Stack stack = new Stack(size);
- The program then enters a while (true) loop, creating a menu-driven interface.
- The user is presented with options to Push, Pop, Peek, Display, or Exit.
- A switch statement handles the user’s choice, calling the appropriate Stack methods.
- Error handling is included for invalid user input.
Key Concepts Illustrated:
- LIFO (Last-In, First-Out): The stack operates on this principle. The last element pushed onto the stack is the first one to be popped.
- Stack Overflow/Underflow: The code handles these potential errors.
- Encapsulation: The elements, top, and maxSize members of the Stack class are private, hiding the internal implementation details from the outside world.
- Menu-Driven Interface: The Main method provides a simple way for the user to interact with the stack.
Summary
This tutorial covered the fundamental operations of a stack data structure in C#, including push, pop, peek, and display. We implemented an interactive console program that allows users to perform these operations dynamically while handling errors like stack overflow and underflow.
By practicing this example, beginners can understand how stacks work, improve problem-solving skills, and apply C# control structures like loops and conditionals. This knowledge is essential for mastering data structures and algorithms in programming.
Exercises and Assessment
Modify the given stack program to include the following additional features:
- Check if the stack is full – Add a method IsFull() that returns true if the stack is full and false otherwise.
- Check if the stack is empty – Add a method IsEmpty() that returns true if the stack is empty and false otherwise.
- Reverse the Stack – Implement a method ReverseStack() that reverses the elements of the stack without using built-in functions.
- Count Elements – Add a method Count() that returns the number of elements currently in the stack.
Multiple-Choice Questions
1. What is the primary characteristic of a stack data structure?
A. First In, First Out (FIFO)
B. Last In, First Out (LIFO)
C. Random Access
D. Bidirectional Processing
2.Which of the following best describes the purpose of the Peek() function in a stack?
A. It removes the top element from the stack.
B. It returns the top element without removing it.
C. It checks if the stack is empty.
D. It reverses the stack elements.
3. If you push the numbers 5, 10, and 15 onto a stack and then pop once, what will be the new top element?
A. 5
B. 10
C. 15
D. The stack becomes empty
4. Given the following C# code, what will be the output?
Stack<int> stack = new Stack<int>(); stack.Push(3); stack.Push(6); stack.Push(9); stack.Pop(); Console.WriteLine(stack.Peek());
A. 3
B. 6 ✅
C. 9
D. The stack is empty
5. Why might you choose a stack over an array when implementing an undo feature in a text editor?
A. Stacks allow random access to elements.
B. Stacks efficiently track the most recent actions using LIFO order. ✅
C. Arrays are slower than stacks for undo operations.
D. Stacks can store unlimited data, while arrays cannot.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.