Sorting Array of Integers in C#
Introduction
Table of Contents
Sorting is a fundamental operation in programming that plays a crucial role in organizing and manipulating data efficiently. Whether you’re working with large datasets or simply need to arrange a list of items in a specific order, understanding sorting algorithms is essential. In this lesson, we will focus on the specific task of sorting an array of integers in C#.
Why is sorting important? Efficient data manipulation heavily relies on the ability to arrange information in a meaningful way. Sorting allows us to bring order to chaos, making it easier to search, retrieve, and analyze data. It enables us to optimize algorithms, improve search efficiency, and enhance overall system performance.
To sort an array of integers, we have a variety of sorting algorithms at our disposal. Each algorithm follows a different approach to rearranging the elements of the array. Some common sorting algorithms include Bubble Sort, Selection Sort, Insertion Sort, Quick Sort, and Merge Sort. Understanding these algorithms and their characteristics empowers us to choose the most suitable solution for our specific requirements.
Throughout this lesson, we will explore these sorting algorithms in detail, focusing on their implementation in C#. We will examine their time and space complexity, learn how to apply them to an array of integers, and understand the trade-offs involved in selecting a particular algorithm. By the end of this lesson, you will have a solid understanding of sorting algorithms and be equipped with the knowledge to efficiently sort arrays of integers in your C# programs.
Objectives
In this lesson, our objectives revolve around the key aspects of understanding, learning, practicing, and applying sorting algorithms to effectively sort arrays of integers in C#. By accomplishing these objectives, you will gain a comprehensive understanding of the importance of sorting in programming, learn various sorting algorithms, practice implementing them in C#, and apply the knowledge to efficiently manipulate data. Let’s delve into the specific objectives of this lesson:
- Understand the Significance of Sorting
Gain a comprehensive understanding of the importance of sorting in programming. Explore how sorting contributes to efficient data manipulation and enhances the performance of algorithms working with arrays of integers.
- Learn Common Sorting Algorithms
Learn and grasp the concepts of common sorting algorithms such as Bubble Sort, Selection Sort, Insertion Sort, and the built-in Array.Sort() method in C#. Understand the principles behind each algorithm and their respective strengths and weaknesses.
- Practice Sorting Techniques
Engage in hands-on practice sessions to implement and execute sorting algorithms on arrays of integers. Develop a practical understanding of the step-by-step processes involved in sorting, reinforcing your knowledge through coding exercises and examples.
- Apply Sorting Skills in Real-world Scenarios
Apply the acquired sorting skills to solve real-world programming challenges. Implement sorting algorithms in scenarios where ordered data is essential, showcasing the practical application of sorting techniques in various programming contexts.
By accomplishing these objectives, you will develop a strong foundation in sorting algorithms, enabling you to effectively sort arrays of integers in your C# programs. Let’s embark on this journey of understanding, learning, practicing, and applying sorting algorithms to enhance your data manipulation skills.
Source code example
using System; namespace SortArrayCSharp { class Program { static void Main() { Console.WriteLine("iNetTutor.com - Array Sorting Example"); // Initialize an array of integers int[] numbers = { 5, 2, 8, 1, 7 }; // Display the original array Console.WriteLine("Original array:"); foreach (var number in numbers) { Console.Write(number + " "); } Console.WriteLine(); // Sort the array using Array.Sort() method Array.Sort(numbers); // Display the sorted array Console.WriteLine("\nSorted array:"); foreach (var number in numbers) { Console.Write(number + " "); } Console.WriteLine(); Console.ReadKey(); } } }
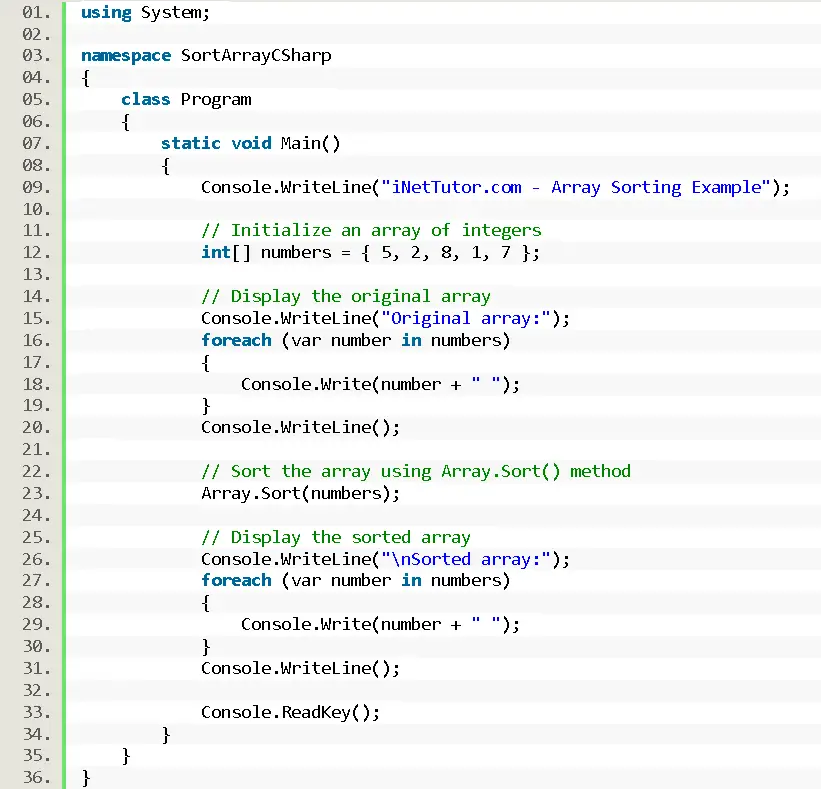
Explanation
This C# code demonstrates the use of the built-in Array.Sort() method to sort an array of integers:
- Introduction and Array Initialization:
- Prints a welcome message to the user.
- Declares and initializes an integer array named numbers containing sample values.
- Displaying Original Array:
- Prints “Original array:” to the console.
- Uses a foreach loop to iterate through each element (number) in the numbers array and prints each value followed by a space.
- Sorting the Array:
- Calls the Array.Sort(numbers) method to sort the elements in the numbers array in ascending order by default.
- Displaying Sorted Array:
- Prints “Sorted array:” to the console.
- Again, utilizes a foreach loop to iterate and print each element (number) after sorting, separated by spaces.
- Waiting for Input (Optional):
- Calls Console.ReadKey() to pause the console and wait for the user to press any key before exiting.
Key Points:
- This code demonstrates a concise and efficient approach to sorting an array using the Array.Sort method.
- The code utilizes clear and well-formatted output for both the original and sorted arrays.
- The optional Console.ReadKey() can be beneficial for beginners to observe the output before the program exits.
While this code is beginner-friendly, it’s important to note that:
- The Array.Sort method sorts the array in ascending order by default. If you need to sort in descending order or based on custom criteria, you’ll need to use additional techniques.
- This method directly modifies the original array (numbers). If you want to preserve the original data, consider creating a copy of the array before sorting.
Remember, this code offers a starting point for understanding array sorting in C#. As you progress, explore other sorting algorithms and techniques to gain a deeper understanding of data manipulation.
Output
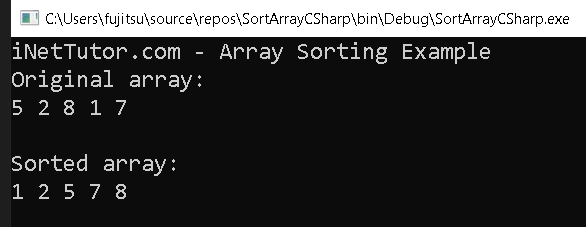
Summary
This lesson equipped you with the power to sort arrays of integers in C#, transforming an unorganized collection of numbers into a neatly arranged sequence. Here’s what you accomplished:
Understanding:
- Grasped the importance of data organization and the role of sorting in various programming applications.
- Gained fundamental knowledge of sorting algorithms, specifically the selection sort approach.
Learning:
- Mastered the implementation of the selection sort algorithm for integer arrays, solidifying your understanding of searching and swapping elements.
- Explored the built-in Array.Sort() method, a convenient tool for efficient array sorting.
Practicing:
- Experienced the sorting process firsthand through the provided code example, reinforcing your conceptual understanding.
- (Optional: If exercises were included) Honed your skills through exercises and experimentation with different sorting scenarios.
Applying:
- Recognized how the ability to sort arrays translates into practical applications across various programming domains.
- (Imagine incorporating application examples discussed here)
Remember, this is just the beginning of your journey into the world of data manipulation. As you progress, explore alternative sorting algorithms, delve deeper into the complexities of performance characteristics, and unlock the power of efficient data organization in your C# programs!
Exercises and Assessment
Exercise:
- Exercise: Implement a Sorting Algorithm
- Objective: Implement a sorting algorithm of your choice to sort an array of integers.
- Instructions:
- Choose a sorting algorithm (e.g., Bubble Sort, Selection Sort, Insertion Sort, Quick Sort, Merge Sort).
- Write a function that takes an array of integers as input and sorts it using the chosen algorithm.
- Test your sorting function with different arrays of integers to ensure it produces the correct sorted output.
- Optional: Compare the performance (time complexity) of your chosen algorithm with other sorting algorithms.
- Resources: You can refer to the code examples and explanations provided in the lesson to guide you in implementing the sorting algorithm.
Assessment:
- Assessment: Code Review
- Objective: Perform a code review of a given code snippet and identify any potential issues or improvements.
- Instructions:
- Review the provided code snippet and analyze it for potential vulnerabilities, bugs, or areas of improvement.
- Identify any security concerns, performance issues, or code quality problems.
- Provide detailed feedback on the identified issues and suggest improvements or fixes.
- Explain the impact of the identified issues and the importance of addressing them.
- Resources: You can refer to the code review principles and best practices discussed in the lesson to guide your assessment.
Lab Exam:
- Lab Exam: Sorting Algorithm Implementation
- Objective: Implement a sorting algorithm to sort an array of integers within a given time limit.
- Instructions:
- Choose a sorting algorithm (different from the one implemented in the exercise) to implement.
- Write a complete program that takes an array of integers as input and sorts it using the chosen algorithm.
- Ensure that your implementation is correct and produces the expected sorted output.
- Optimize your code for efficiency and consider factors such as time complexity and space complexity.
- Test your program with various input arrays to validate its correctness and efficiency.
- Resources: You can refer to the sorting algorithm explanations and code examples provided in the lesson to guide you in implementing the chosen algorithm.
These exercises, assessment, and lab exam will provide you with opportunities to further improve your understanding and implementation of sorting algorithms. They will also help you practice code review skills and assess your ability to apply sorting algorithms in real-world scenarios. Remember to refer to the relevant resources and code examples from the lesson to support your learning and implementation.
Quiz
- Which of the following statements is NOT true about the Array.Sort() method in C#?
a) It sorts the elements in ascending order by default.
b) It modifies the original array in place.
c) It can be used to sort arrays of different data types like strings and doubles.
d) It allows specifying a custom comparison function for complex sorting logic. - What is the main purpose of sorting an array?
a) To remove duplicate elements from the array.
b) To reverse the order of elements in the array.
c) To organize the elements in a specific order (e.g., ascending, descending).
d) To increase the size of the array. - Which of the following is NOT a step involved in the selection sort algorithm?
a) Find the minimum element in the unsorted part of the array.
b) Swap the minimum element with the first element of the unsorted part.
c) Compare each element in the unsorted part with the current minimum element.
d) Remove the minimum element from the array after it’s found. - What is the advantage of using the built-in Array.Sort() method compared to implementing a custom sorting algorithm like selection sort?
a) The custom algorithm is always more efficient for all scenarios.
b) The Array.Sort() method offers a concise and optimized approach.
c) Selection sort provides a clearer understanding of the sorting process.
d) The custom algorithm allows for more control over the sorting logic. - Which of the following applications doesn’t benefit from using array sorting?
a) Ordering a list of student names alphabetically.
b) Analyzing financial data based on specific criteria.
c) Searching for a specific item in a large inventory list.
d) Displaying the highest score achieved in a game.
Related Topics and Articles:
Counting Positive and Negative Numbers Array
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.