Queues in C#
Introduction
Table of Contents
In C#, a queue is a data structure that follows the First-In, First-Out (FIFO) principle. This means that the first element added to the queue is the first one to be removed. Queues are often used in scenarios where the order of processing matters, such as task scheduling, handling requests in a web server, or managing customer service operations. The Queue<T> class in C# provides a simple yet powerful way to manage a collection of items in a queue, offering methods to enqueue, dequeue, and peek at elements without exposing the underlying data structure directly.
The Queue<T> class is part of the System.Collections.Generic namespace, and it provides an easy-to-use interface for managing elements in a queue. It supports several operations such as Enqueue() to add an item, Dequeue() to remove and return the first item, and Peek() to view the first item without removing it. Queues are ideal for scenarios where items need to be processed sequentially in the order they were added, making them essential in many real-world applications like message processing systems, print job management, or event handling.
Objectives
The objectives of this module on Queues in C# are designed to ensure a comprehensive understanding and practical implementation of queues in real-world applications. By the end of this module, learners will have gained the necessary knowledge and skills to effectively work with queues, incorporating them into their projects and applications. The learning journey will be structured around the following key objectives:
- Understand the concept of queues and their importance in various applications, specifically focusing on the First-In, First-Out (FIFO) principle and how it differs from other data structures.
- Learn how to implement queues in C# using the built-in Queue<T> class, exploring key methods such as Enqueue(), Dequeue(), and Peek(), and how these operations are used in different scenarios.
- Practice applying queues in practical coding exercises, with hands-on experience in designing programs that require sequential processing, such as task management or event handling systems.
- Apply the knowledge of queues to real-world problem-solving, utilizing queues in more complex applications like message processing systems, print job queues, and web request handling, thereby solidifying your understanding of when and how to use queues effectively.
Source code example
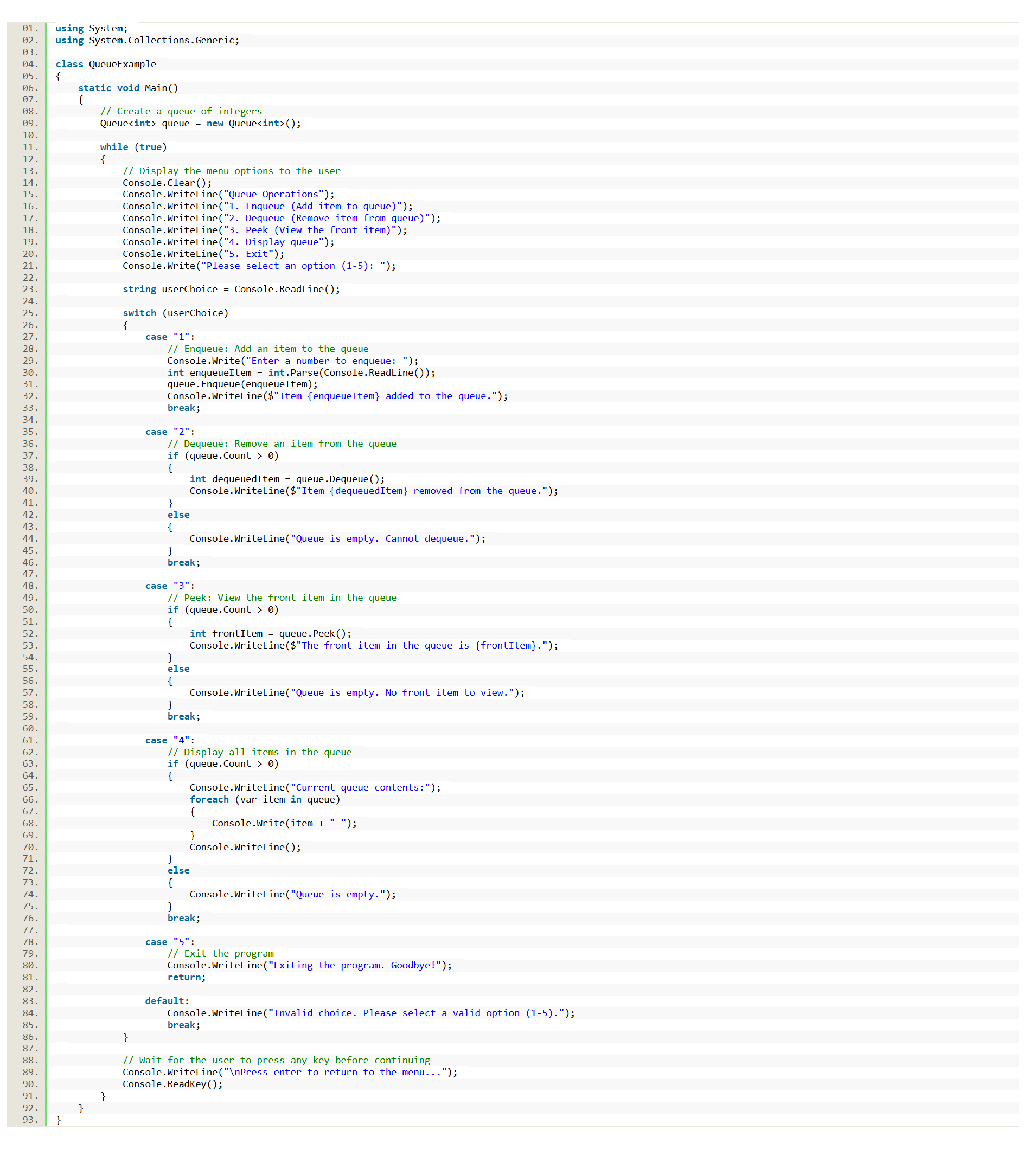
Explanation
This C# code demonstrates the use of a Queue data structure. A queue is a First-In, First-Out (FIFO) collection, meaning the first element added is the first one removed. The code provides a simple menu-driven interface to interact with the queue. Let’s break down the code step by step:
- using System; and using System.Collections.Generic;: These lines import necessary namespaces. System provides fundamental classes and base types, and System.Collections.Generic provides interfaces and classes that define generic collections, including Queue<T>.
- class QueueExample: This declares the class that contains the main logic of the program.
- static void Main(): This is the entry point of the program.
- Queue<int> queue = new Queue<int>();: This line creates a new queue named queue that will store integers (int). The Queue<int> is a generic collection, so it can only hold integers in this example.
- while (true): This loop creates the main menu structure, running indefinitely until the user chooses to exit.
- Menu Display: The code within the loop first clears the console (Console.Clear()) and then displays the menu options to the user: Enqueue, Dequeue, Peek, Display, and Exit.
- string userChoice = Console.ReadLine();: This line reads the user’s input from the console and stores it in the userChoice variable.
- switch (userChoice): This switch statement handles the different menu options based on the user’s input.
- case “1” (Enqueue):
- Prompts the user to enter a number to enqueue.
- int enqueueItem = int.Parse(Console.ReadLine()); converts the user’s input (which is a string) to an integer.
- queue.Enqueue(enqueueItem); adds the integer to the back of the queue.
- A confirmation message is displayed.
- case “2” (Dequeue):
- Checks if the queue is not empty (queue.Count > 0).
- If not empty, int dequeuedItem = queue.Dequeue(); removes and returns the element at the front of the queue.
- A message is displayed indicating the dequeued item.
- If the queue is empty, a message is displayed informing the user.
- case “3” (Peek):
- Checks if the queue is not empty.
- If not empty, int frontItem = queue.Peek(); retrieves (but does not remove) the element at the front of the queue.
- A message is displayed showing the front item.
- If the queue is empty, a message is displayed.
- case “4” (Display):
- Checks if the queue is not empty.
- If not empty, it iterates through the queue using a foreach loop and prints each element followed by a space. Note that iterating through the queue does not remove elements.
- If the queue is empty, a message is displayed.
- case “5” (Exit):
- Prints an exit message.
- return; exits the Main method, effectively ending the program.
- default:
- Handles invalid user input (anything other than 1-5).
- Prints an error message.
- case “1” (Enqueue):
- Console.WriteLine(“\nPress enter to return to the menu…”); and Console.ReadKey();: These lines pause the program after each operation, waiting for the user to press Enter before redisplaying the menu. This makes the interaction more user-friendly.
Key Concepts Demonstrated:
- Queues: The core concept of a FIFO data structure.
- Generic Collections: Using Queue<int> to create a queue specifically for integers.
- Menu-Driven Interface: Creating a simple interactive program using a menu.
- switch Statement: Handling different user choices.
- Error Handling: Checking for an empty queue before attempting to dequeue or peek.
- foreach Loop: Iterating through the queue to display its contents.
- Console.ReadLine() and int.Parse(): Getting user input and converting it to an integer.
This code provides a basic but functional example of how to use queues in C#. It’s a good starting point for learning about data structures and building interactive console applications.
Summary
In this lesson, we explored the concept of queues in C# through practical examples and user interaction. We learned how a queue follows the First-In, First-Out (FIFO) principle, where the first item added is the first one to be removed. Using the Queue<T> class, we demonstrated basic queue operations such as Enqueue (adding items), Dequeue (removing items), and Peek (viewing the front item). By allowing the user to input data and perform these operations through a simple menu-driven program, the lesson provided a hands-on approach to understanding how queues work and how to implement them in real-world scenarios.
Exercises and Assessment
Exercise: Queue-based Task Scheduler
Objective:
Create a simple task scheduler using a queue. The program will allow the user to add tasks, remove completed tasks, and view the next task to be completed. The tasks will be processed in the order they are added (FIFO).
Instructions:
- Create a Queue<string> to store task names.
- The program should display the following menu options:
- 1. Add Task – Prompt the user to enter a task description and add it to the queue.
- 2. Complete Task – Remove the first task from the queue and display the task that has been completed.
- 3. View Next Task – Show the first task in the queue without removing it.
- 4. View All Tasks – Display all tasks currently in the queue.
- 5. Exit – Exit the program.
- Use a loop to repeatedly show the menu until the user selects “Exit.”
- Ensure the program handles edge cases, such as attempting to complete a task when the queue is empty.
Example Output:
Task Scheduler
- Add Task
- Complete Task
- View Next Task
- View All Tasks
- Exit
Please select an option (1-5): 1
Enter task description: Finish report
Please select an option (1-5): 2
Completed task: Finish report
Please select an option (1-5): 3
Next task: [None, as the queue is empty]
Please select an option (1-5): 4
No tasks in the queue.
QUIZ
- What is the key principle that defines the behavior of a queue in C#?
a) Last-In, First-Out (LIFO)
b) First-In, First-Out (FIFO)
c) Random Access
d) First-In, Last-Out (FILO) - Which method in C#’s Queue<T> class would you use to remove and return the first item in the queue?
a) Peek()
b) Enqueue()
c) Dequeue()
d) Clear() - If you want to add a task “Complete homework” to the queue, which of the following C# statements is correct?
a) queue.Push(“Complete homework”);
b) queue.Enqueue(“Complete homework”);
c) queue.Add(“Complete homework”);
d) queue.Insert(“Complete homework”); - Consider a queue with the following items: [1, 2, 3, 4]. After performing a Dequeue() operation, what will be the content of the queue?
a) [2, 3, 4]
b) [1, 2, 3]
c) [3, 4]
d) [4, 3, 2] - Which of the following scenarios would be best suited for using a queue in a C# application?
a) Managing login history in reverse order
b) Scheduling print jobs for multiple users
c) Tracking user actions for undo/redo functionality
d) Sorting a list of numbers in ascending order
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.