Python Project Ideas
Introduction
Table of Contents
- Introduction
- Web Development Projects (Python + Django)
- API Development Projects (Python + FastAPI)
- Data Analysis Projects (Python + Pandas/NumPy)
- Machine Learning Projects (Python + TensorFlow/PyTorch)
- Web Scraping Projects (Python + BeautifulSoup)
- Computer Vision Projects (Python + OpenCV)
- Data Visualization Projects (Python + Matplotlib)
- Database Management Projects (Python + SQLAlchemy)
- Game Development Projects (Python + Pygame)
- Interactive Computing Projects (Python + Jupyter Notebook)
- Mobile App Development Projects (Python + Kivy)
- Big Data Processing Projects (Python + PySpark)
- Conclusion
Python has solidified its position as one of the most versatile and beginner-friendly programming languages in the world. Its simplicity, readability, and extensive ecosystem of libraries and frameworks make it an ideal choice for a wide range of applications, from web development to machine learning, data analysis, and beyond. Whether you’re a novice coder looking to build your first project or an experienced developer seeking to explore new domains, Python offers tools that cater to every need. The image provided highlights some of the most powerful combinations of Python with its libraries and frameworks, each opening doors to unique project opportunities.
For web development, Python paired with Django allows developers to create robust, scalable websites and applications with ease. Django’s “batteries-included” philosophy provides everything you need to build secure and maintainable web platforms. Similarly, FastAPI has gained popularity for API development, enabling developers to create high-performance APIs with minimal code, thanks to its asynchronous capabilities and automatic documentation features. When it comes to data analysis, the Pandas and NumPy duo is unbeatable, offering efficient data manipulation and numerical computation tools that are essential for working with large datasets.
For those interested in artificial intelligence, Python’s machine learning libraries like TensorFlow and PyTorch provide the foundation for building intelligent systems, from image classifiers to natural language processing models. Web scraping, a critical skill for data gathering, is made simple with BeautifulSoup, which allows developers to extract information from websites effortlessly. In the realm of computer vision, OpenCV empowers developers to work on projects involving image and video processing, such as facial recognition or motion detection.
Data visualization is another area where Python shines, with Matplotlib enabling the creation of insightful charts and graphs to make sense of complex datasets. For database management, SQLAlchemy offers a powerful toolkit to interact with databases, making it easier to build applications that require persistent data storage. Python also caters to game developers through Pygame, a library that simplifies the creation of 2D games with features like sprite handling and collision detection.
Interactive computing is made accessible with Jupyter Notebook, a tool that allows for real-time code execution and visualization, perfect for data exploration and teaching. For mobile app development, Kivy provides a framework to build cross-platform applications with intuitive user interfaces. Finally, for big data processing, PySpark enables developers to handle massive datasets efficiently, leveraging the power of Apache Spark.
This guide will explore project ideas across these categories, offering inspiration for your next Python endeavor. Each project is designed to help you apply the relevant library or framework in a practical, real-world context, whether you’re building a web app, analyzing data, or diving into machine learning. By working on these projects, you’ll not only sharpen your Python skills but also gain hands-on experience with some of the most in-demand tools in the tech industry. Let’s dive into the project ideas and unleash your creativity with Python!
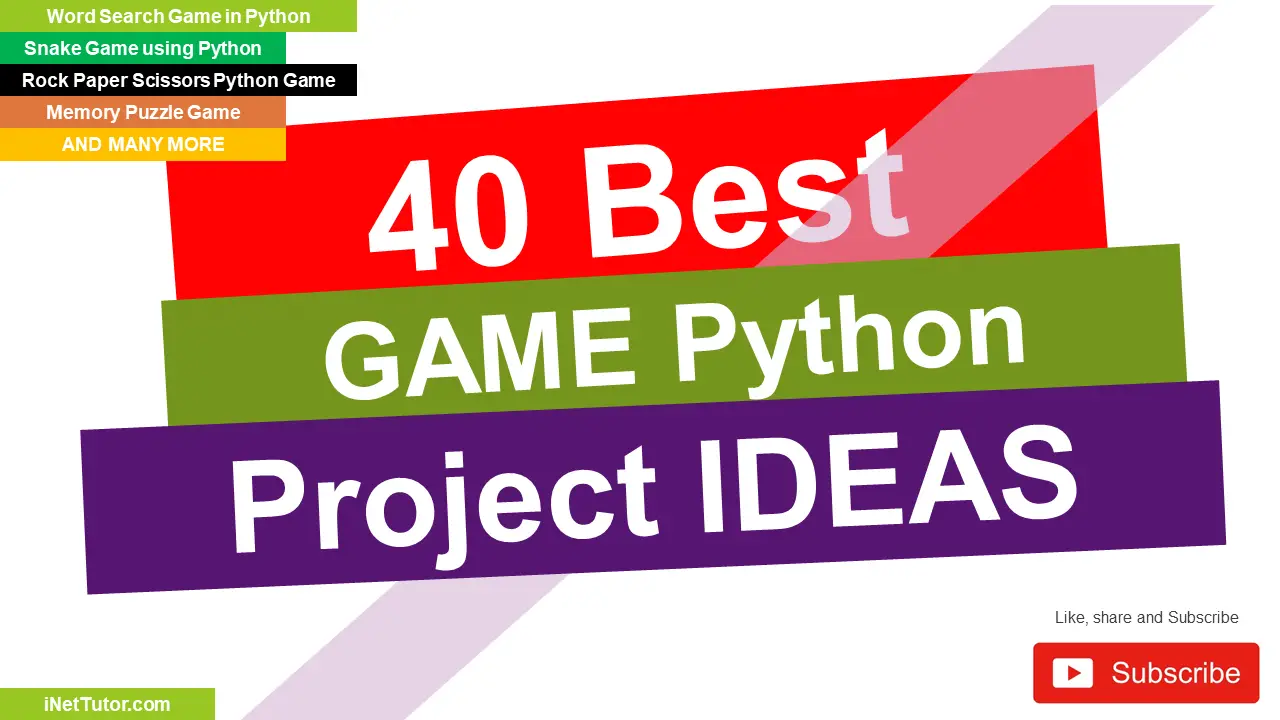
Web Development Projects (Python + Django)
- Personal Finance Tracker
Build a web application that helps users track their income, expenses, and savings goals. With Django, you can create a user-friendly interface where users can log in, input their financial data, and categorize transactions (e.g., groceries, utilities). Use Django’s authentication system to ensure secure user access and store data in a SQLite or PostgreSQL database. Add features like monthly summaries, budget alerts, and visual charts (integrating a library like Chart.js) to display spending patterns. This project will teach you how to handle user authentication, manage databases, and create dynamic web pages with Django’s template engine. You’ll also learn to implement CRUD (Create, Read, Update, Delete) operations for transactions and how to structure a Django project with reusable apps. - Online Recipe Book
Create a platform where users can browse, add, and share recipes. Implement features like user registration, a search bar to filter recipes by ingredients or cuisine, and a rating system. Use Django’s ORM to manage recipe data, including fields for ingredients, preparation steps, and cooking time. Add image upload functionality for recipe photos using Django’s file-handling capabilities. You can enhance the app by integrating a commenting system for user feedback and a “favorite” feature to save recipes. This project will help you master Django’s forms, model relationships (e.g., one-to-many for users and recipes), and static file management. - Event Management System
Develop a web app for organizing events, such as conferences or meetups. Users should be able to create events, RSVP, and view event details like date, location, and agenda. Use Django to handle user authentication and event registration. Implement a calendar view (using a library like FullCalendar) to display upcoming events. Add features like email notifications for RSVPs using Django’s email framework. This project will teach you how to manage complex relationships in Django models (e.g., users attending multiple events) and integrate third-party JavaScript libraries with Django templates. - E-commerce Storefront
Build a simple online store where users can browse products, add them to a cart, and place orders. Use Django to create product categories, manage inventory, and handle user orders. Integrate a payment gateway like Stripe for processing payments. Add features like product reviews, a wishlist, and order tracking. This project will help you understand Django’s session framework (for managing carts), integrate external APIs, and handle secure transactions. You’ll also learn to optimize database queries for better performance. - Job Board Platform
Create a job posting and application platform where employers can post jobs, and job seekers can apply. Use Django to manage user roles (employers and applicants), job listings, and applications. Implement a search and filter system to find jobs by location, category, or salary range. Add a feature for users to upload resumes and employers to review applications. This project will teach you how to implement role-based access control, handle file uploads, and create a responsive UI with Django templates. - Online Quiz Platform
Develop a platform for creating and taking quizzes on various topics. Users can sign up, create quizzes with multiple-choice questions, and take quizzes created by others. Use Django to store quiz data, track user scores, and display leaderboards. Add a timer for quizzes and a results page with detailed feedback. This project will help you learn how to manage complex forms in Django, handle user sessions for quiz progress, and create dynamic views for quiz-taking. - Social Media Dashboard
Build a dashboard where users can manage their social media posts across platforms (e.g., a mock version). Use Django to create a user interface for scheduling posts, viewing analytics, and managing accounts. Simulate API calls to social media platforms and display mock data like post engagement metrics. This project will teach you how to work with Django’s admin panel for content management, handle asynchronous tasks (e.g., scheduling posts), and create a clean, modular codebase. - Library Management System
Create a web app for managing a library’s book inventory, user memberships, and borrowing history. Use Django to handle book checkouts, returns, and fines for late returns. Implement a search feature for books by title, author, or genre. Add user authentication to track borrowing history. This project will help you understand Django’s model relationships (e.g., many-to-many for users and books), implement search functionality, and manage database migrations. - Fitness Tracker
Develop a web app where users can log their workouts, track progress, and set fitness goals. Use Django to create models for exercises, workout sessions, and user profiles. Add features like workout history, progress charts, and goal-setting tools. You can integrate a third-party API (e.g., a fitness API) to fetch exercise data. This project will teach you how to handle time-series data, create user dashboards, and integrate external APIs with Django. - Online Voting System
Build a secure online voting platform for small-scale elections (e.g., a club or classroom). Use Django to manage candidates, voters, and votes. Implement user authentication and ensure each user can vote only once. Add features like real-time vote counting and result visualization. This project will help you learn about security practices in Django (e.g., preventing duplicate votes), handle form submissions, and create admin dashboards for managing elections.
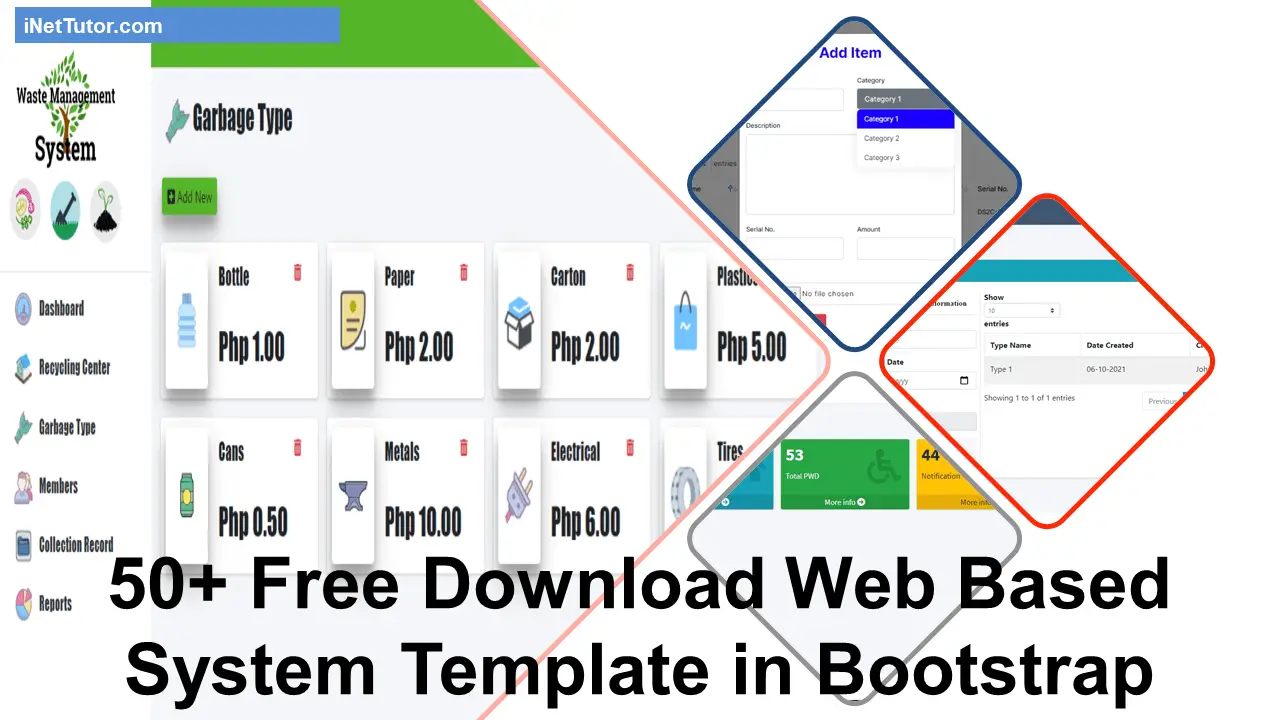
API Development Projects (Python + FastAPI)
- Weather Forecast API
Create an API that provides weather forecasts for a given location using FastAPI. Integrate a third-party weather API (e.g., OpenWeatherMap) to fetch real-time data. Users can send a GET request with a city name, and the API returns the current weather, temperature, and a 5-day forecast. Use FastAPI’s automatic documentation to make the API user-friendly. Add error handling for invalid locations and rate limiting to prevent abuse. This project will teach you how to work with external APIs, handle asynchronous requests in FastAPI, and implement proper error handling for robust API design. - To-Do List API
Build a RESTful API for managing a to-do list. Users can create, read, update, and delete tasks via API endpoints. Use FastAPI with a database like SQLite (via SQLAlchemy) to store tasks. Add authentication using JWT tokens to secure the API, ensuring only authorized users can access their tasks. Include features like task prioritization and due dates. This project will help you understand FastAPI’s dependency injection, integrate databases with APIs, and implement token-based authentication for security. - Book Recommendation API
Develop an API that recommends books based on user preferences (e.g., genre, author). Use FastAPI to create endpoints where users can input their preferences, and the API returns a list of recommended books. Store book data in a database and implement a simple recommendation algorithm (e.g., filtering by genre). Add endpoints for adding new books to the database. This project will teach you how to design API endpoints, handle query parameters, and implement basic recommendation logic with FastAPI. - Currency Converter API
Create an API that converts amounts between different currencies. Use FastAPI to build endpoints where users can specify the source currency, target currency, and amount. Integrate a third-party API (e.g., ExchangeRate-API) to fetch real-time exchange rates. Add caching to reduce API calls and improve performance. This project will help you learn how to integrate external APIs, implement caching with FastAPI, and handle numeric data in API responses. - Fitness Tracker API
Build an API for a fitness app where users can log workouts, track calories, and view progress. Use FastAPI to create endpoints for adding workouts, fetching user stats, and calculating calorie burn based on exercise type. Store data in a database and add user authentication to secure endpoints. This project will teach you how to design a scalable API, handle relational data, and implement user-specific endpoints with FastAPI. - News Aggregator API
Develop an API that aggregates news articles from multiple sources. Use FastAPI to create endpoints for fetching news by category (e.g., technology, sports). Integrate a third-party news API (e.g., NewsAPI) to fetch articles and return them in a structured format. Add filtering options like date range or source. This project will help you understand how to handle large datasets in API responses, implement filtering, and manage external API integrations with FastAPI. - Inventory Management API
Create an API for managing inventory in a small business. Use FastAPI to build endpoints for adding products, updating stock levels, and fetching inventory reports. Store data in a database and add authentication to restrict access. Include features like low-stock alerts and product categorization. This project will teach you how to design a business-oriented API, handle CRUD operations, and implement role-based access control with FastAPI. - Event Scheduler API
Build an API for scheduling events, such as meetings or appointments. Use FastAPI to create endpoints for creating events, fetching upcoming events, and sending reminders. Integrate a database to store event data and add user authentication. Include features like time zone support and conflict detection. This project will help you learn how to handle datetime data in APIs, implement scheduling logic, and manage user-specific data with FastAPI. - Chatbot API
Develop an API that powers a simple chatbot. Use FastAPI to create an endpoint where users can send messages, and the API responds with pre-programmed replies (or integrate a basic NLP model). Store conversation history in a database for context-aware responses. This project will teach you how to handle real-time interactions, store session data, and integrate natural language processing with FastAPI. - URL Shortener API
Create an API that shortens URLs and tracks click statistics. Use FastAPI to build endpoints for shortening URLs, redirecting to the original URL, and fetching click stats. Store data in a database and add rate limiting to prevent abuse. This project will help you learn how to implement URL redirection, track usage metrics, and secure APIs with FastAPI.
Data Analysis Projects (Python + Pandas/NumPy)
- Sales Data Analysis
Analyze a dataset of sales transactions to identify trends and insights. Use Pandas to load the data, clean it (e.g., handle missing values), and perform calculations like total revenue per product. Use NumPy for numerical operations, such as calculating growth rates. Create visualizations (e.g., sales over time) to present your findings. This project will teach you how to manipulate large datasets, perform aggregations with Pandas, and use NumPy for efficient numerical computations. - Financial Dashboard
Build a dashboard to analyze personal or business finances using Pandas and NumPy. Load financial data (e.g., expenses, income) and calculate metrics like net profit, expense categories, and savings rate. Use Pandas to group data by time periods (e.g., monthly) and NumPy to compute statistical measures like variance. Visualize the results with a library like Matplotlib. This project will help you learn data aggregation, time-series analysis, and how to create actionable insights from financial data. - Student Performance Analysis
Analyze a dataset of student grades to identify patterns, such as average scores per subject or class. Use Pandas to clean the data, handle outliers, and calculate metrics like pass rates. Use NumPy to perform statistical analysis, such as standard deviation of scores. Create visualizations to compare performance across subjects. This project will teach you how to handle educational data, perform statistical analysis, and present findings effectively. - Stock Market Analysis
Analyze historical stock price data to identify trends and calculate returns. Use Pandas to load and preprocess the data, calculating metrics like daily returns and moving averages. Use NumPy to compute volatility and correlation between stocks. Visualize price trends and returns using Matplotlib. This project will help you learn time-series analysis, financial calculations, and how to work with real-world datasets in Pandas. - Weather Data Analysis
Analyze a weather dataset to identify seasonal patterns, such as temperature or rainfall trends. Use Pandas to load and clean the data, handling missing values and converting timestamps. Use NumPy to calculate averages, max/min values, and anomalies. Create visualizations to show trends over time. This project will teach you how to handle environmental data, perform time-series analysis, and use Pandas for data wrangling. - E-commerce Customer Analysis
Analyze customer purchase data from an e-commerce platform to understand buying behavior. Use Pandas to segment customers by demographics, calculate metrics like average order value, and identify repeat buyers. Use NumPy for statistical analysis, such as customer lifetime value. Visualize purchasing patterns with charts. This project will help you learn customer segmentation, behavioral analysis, and how to derive business insights from data. - Traffic Data Analysis
Analyze traffic data (e.g., vehicle counts, speeds) to identify peak hours or congestion patterns. Use Pandas to clean and aggregate the data, calculating metrics like average speed per hour. Use NumPy to perform statistical analysis, such as identifying outliers. Visualize traffic trends with graphs. This project will teach you how to handle transportation data, perform temporal analysis, and use Pandas for data summarization. - Healthcare Data Analysis
Analyze a healthcare dataset (e.g., patient records) to identify trends, such as common diagnoses or treatment outcomes. Use Pandas to clean the data, handle categorical variables, and calculate metrics like average recovery time. Use NumPy for statistical analysis, such as correlation between variables. Visualize health trends with charts. This project will help you learn how to handle sensitive data, perform medical analysis, and use Pandas for data preprocessing. - Social Media Engagement Analysis
Analyze a dataset of social media posts to measure engagement (likes, comments, shares). Use Pandas to clean the data, calculate engagement rates, and segment posts by type (e.g., video, image). Use NumPy to compute statistical measures like variance in engagement. Visualize engagement trends over time. This project will teach you how to handle social media data, perform engagement analysis, and use Pandas for data grouping. - Energy Consumption Analysis
Analyze energy usage data (e.g., electricity consumption) to identify patterns, such as peak usage times. Use Pandas to load and preprocess the data, calculating metrics like daily consumption averages. Use NumPy to perform statistical analysis, such as identifying anomalies. Visualize usage trends with graphs. This project will help you learn how to handle utility data, perform time-series analysis, and use Pandas for energy insights.
Machine Learning Projects (Python + TensorFlow/PyTorch)
- Image Classifier for Cats and Dogs
Build a model to classify images as either cats or dogs using TensorFlow or PyTorch. Use a dataset like the Kaggle Cats vs. Dogs dataset, preprocess the images (e.g., resize, normalize), and build a convolutional neural network (CNN). Train the model, evaluate its accuracy, and test it on new images. This project will teach you how to handle image data, build and train a CNN, and evaluate model performance in TensorFlow or PyTorch. - Chatbot for Customer Support
Create a chatbot that answers common customer support queries using PyTorch. Use a dataset of customer queries and responses, preprocess the text with tokenization, and build a sequence-to-sequence model (e.g., LSTM or Transformer). Train the model to generate relevant responses and test it with sample queries. This project will help you learn natural language processing, build sequence models, and use PyTorch for text generation. - Handwritten Digit Recognition
Build a model to recognize handwritten digits using the MNIST dataset with TensorFlow. Preprocess the images, build a neural network (e.g., a simple CNN), and train the model to classify digits (0-9). Evaluate the model’s accuracy and test it on new handwritten digits. This project will teach you how to work with image classification, build neural networks, and use TensorFlow for machine learning tasks. - Sentiment Analysis for Reviews
Develop a model to classify product reviews as positive, negative, or neutral using PyTorch. Use a dataset of reviews, preprocess the text (e.g., tokenization, stemming), and build a model like an LSTM or BERT. Train the model and evaluate its performance on a test set. This project will help you learn text classification, handle NLP tasks, and use PyTorch for sentiment analysis. - Stock Price Prediction
Build a model to predict stock prices using historical data with TensorFlow. Use a dataset of stock prices, preprocess the data (e.g., normalize, create sequences), and build a model like an LSTM. Train the model to predict future prices and evaluate its performance. This project will teach you how to handle time-series data, build predictive models, and use TensorFlow for financial forecasting. - Facial Expression Recognition
Create a model to recognize facial expressions (e.g., happy, sad) using PyTorch. Use a dataset like FER2013, preprocess the images, and build a CNN to classify expressions. Train the model and test it on new images. This project will help you learn how to handle facial data, build CNNs for classification, and use PyTorch for computer vision tasks. - Spam Email Classifier
Build a model to classify emails as spam or not spam using TensorFlow. Use a dataset of emails, preprocess the text (e.g., tokenization, TF-IDF), and build a model like a neural network or logistic regression. Train the model and evaluate its accuracy. This project will teach you how to handle text data, build classification models, and use TensorFlow for spam detection. - Music Genre Classification
Develop a model to classify music clips by genre (e.g., rock, classical) using PyTorch. Use a dataset like GTZAN, extract audio features (e.g., MFCCs), and build a model like a CNN or RNN. Train the model and test it on new clips. This project will help you learn how to handle audio data, build classification models, and use PyTorch for audio processing. - Credit Risk Prediction
Build a model to predict credit risk (e.g., default likelihood) using TensorFlow. Use a dataset of credit data, preprocess the features (e.g., handle missing values), and build a model like a neural network. Train the model and evaluate its performance. This project will teach you how to handle financial data, build predictive models, and use TensorFlow for risk assessment. - Traffic Sign Recognition
Create a model to recognize traffic signs using the GTSRB dataset with PyTorch. Preprocess the images, build a CNN to classify signs (e.g., stop, yield), and train the model. Test it on new images. This project will help you learn how to handle real-world image data, build CNNs for classification, and use PyTorch for computer vision applications.
Web Scraping Projects (Python + BeautifulSoup)
- E-commerce Price Tracker
Build a script to scrape product prices from an e-commerce website (e.g., Amazon) using BeautifulSoup. Extract product names, prices, and ratings, and store the data in a CSV file. Add a feature to compare prices across multiple products and alert users if a price drops. This project will teach you how to navigate HTML structures, extract data with BeautifulSoup, and handle data storage for price tracking. - News Aggregator
Create a script to scrape news articles from a website (e.g., BBC) using BeautifulSoup. Extract headlines, summaries, and publication dates, and store them in a database. Add a feature to categorize articles by topic (e.g., politics, technology). This project will help you learn how to scrape dynamic websites, handle text data, and organize scraped data into a structured format. - Job Listings Scraper
Build a script to scrape job listings from a job board (e.g., Indeed) using BeautifulSoup. Extract job titles, companies, locations, and salaries, and store the data in a CSV file. Add a feature to filter jobs by keyword or location. This project will teach you how to handle pagination on websites, extract structured data, and process job-related information with BeautifulSoup. - Weather Data Scraper
Create a script to scrape weather data from a website (e.g., Weather Underground) using BeautifulSoup. Extract temperature, humidity, and forecasts for a given city, and store the data in a JSON file. Add a feature to fetch historical weather data. This project will help you learn how to scrape tabular data, handle datetime information, and store environmental data with BeautifulSoup. - Recipe Scraper
Build a script to scrape recipes from a cooking website (e.g., AllRecipes) using BeautifulSoup. Extract recipe names, ingredients, and instructions, and store them in a database. Add a feature to search recipes by ingredient. This project will teach you how to handle nested HTML elements, extract culinary data, and organize recipes into a searchable format. - Social Media Trends Scraper
Create a script to scrape trending topics or hashtags from a social media platform (e.g., Twitter, if accessible) using BeautifulSoup. Extract trending topics, post counts, and timestamps, and store the data in a CSV file. Add a feature to track trends over time. This project will help you learn how to scrape dynamic content, handle social media data, and analyze trends with BeautifulSoup. - Real Estate Listings Scraper
Build a script to scrape real estate listings from a website (e.g., Zillow) using BeautifulSoup. Extract property details like price, location, and square footage, and store the data in a database. Add a feature to filter listings by price range. This project will teach you how to scrape structured data, handle real estate information, and build a searchable database with BeautifulSoup. - Event Listings Scraper
Create a script to scrape event listings from a website (e.g., Eventbrite) using BeautifulSoup. Extract event names, dates, locations, and ticket prices, and store the data in a CSV file. Add a feature to filter events by date or category. This project will help you learn how to handle event data, scrape dynamic websites, and organize scraped data with BeautifulSoup. - Stock Market Data Scraper
Build a script to scrape stock market data from a financial website (e.g., Yahoo Finance) using BeautifulSoup. Extract stock prices, volumes, and company names, and store the data in a JSON file. Add a feature to track price changes over time. This project will teach you how to scrape financial data, handle numerical information, and store time-series data with BeautifulSoup. - Book Reviews Scraper
Create a script to scrape book reviews from a website (e.g., Goodreads) using BeautifulSoup. Extract book titles, ratings, and reviews, and store the data in a database. Add a feature to calculate average ratings per book. This project will help you learn how to scrape user-generated content, handle text data, and analyze reviews with BeautifulSoup.
Computer Vision Projects (Python + OpenCV)
- Face Detection App
Build an application to detect faces in images or live video using OpenCV. Use OpenCV’s Haar Cascade classifier to identify faces, draw bounding boxes around them, and display the results. Add a feature to count the number of faces detected. This project will teach you how to work with image processing, use pre-trained models in OpenCV, and handle real-time video streams. - Object Tracking System
Create a system to track moving objects in a video using OpenCV. Use techniques like background subtraction and contour detection to identify objects, and track their movement across frames. Add a feature to display the object’s trajectory. This project will help you learn how to handle video data, implement tracking algorithms, and use OpenCV for motion analysis. - Color Detection Tool
Build a tool to detect specific colors in an image or video using OpenCV. Convert the image to HSV color space, set a color range (e.g., for blue), and create a mask to highlight the color. Add a feature to display the percentage of the image covered by the color. This project will teach you how to work with color spaces, apply image masking, and use OpenCV for color analysis. - Barcode Scanner
Create an application to scan and decode barcodes using OpenCV. Use OpenCV to detect barcodes in an image, extract the barcode region, and decode it using a library like pyzbar. Add a feature to display the decoded information. This project will help you learn how to process images, detect patterns, and integrate barcode decoding with OpenCV. - Motion Detection System
Build a system to detect motion in a video feed using OpenCV. Use frame differencing to identify changes between frames, and highlight areas of motion with bounding boxes. Add a feature to trigger an alert when motion is detected. This project will teach you how to handle video streams, implement motion detection algorithms, and use OpenCV for security applications. - Text Recognition in Images
Create an application to extract text from images using OpenCV and Tesseract OCR. Use OpenCV to preprocess the image (e.g., grayscale, thresholding), and pass it to Tesseract for text extraction. Add a feature to highlight the detected text. This project will help you learn how to preprocess images for OCR, integrate Tesseract with OpenCV, and handle text data. - Lane Detection for Autonomous Driving
Build a system to detect lanes in a road video using OpenCV. Use edge detection (e.g., Canny) and Hough Transform to identify lane lines, and overlay them on the video. Add a feature to warn if the vehicle deviates from the lane. This project will teach you how to apply computer vision in autonomous driving, use geometric transforms, and handle video processing with OpenCV. - Gesture Recognition System
Create a system to recognize hand gestures (e.g., wave, fist) using OpenCV. Use skin color detection to segment the hand, track its movement, and classify gestures based on shape or motion. Add a feature to map gestures to actions (e.g., play/pause). This project will help you learn how to handle gesture recognition, apply image segmentation, and use OpenCV for human-computer interaction. - Augmented Reality App
Build a simple AR app to overlay virtual objects on a live video feed using OpenCV. Use marker detection (e.g., ArUco markers) to identify positions, and overlay 3D objects or text on the video. Add a feature to interact with the objects. This project will teach you how to implement augmented reality, use marker-based tracking, and handle 3D rendering with OpenCV. - Traffic Light Detection
Create a system to detect traffic lights in a video using OpenCV. Use color segmentation to identify red, yellow, and green lights, and draw bounding boxes around them. Add a feature to classify the light state (e.g., stop, go). This project will help you learn how to handle color-based detection, process video data, and use OpenCV for traffic applications.
Data Visualization Projects (Python + Matplotlib)
- Stock Market Trends Visualization
Create visualizations to analyze stock market trends using Matplotlib. Use a dataset of stock prices, plot price trends over time, and add moving averages to identify patterns. Include volume charts and annotations for significant events (e.g., price spikes). This project will teach you how to create time-series visualizations, add multiple plots, and use Matplotlib for financial analysis. - Interactive Data Dashboard
Build an interactive dashboard to visualize a dataset (e.g., sales data) using Matplotlib. Create plots like bar charts for sales by region, line charts for trends, and pie charts for category breakdowns. Add interactivity using a library like Plotly for zooming or hovering. This project will help you learn how to create comprehensive dashboards, combine multiple visualizations, and use Matplotlib for interactive plotting. - Weather Data Visualization
Visualize weather data (e.g., temperature, rainfall) using Matplotlib. Create line plots for temperature trends, bar charts for rainfall by month, and scatter plots for anomalies. Add annotations for extreme weather events. This project will teach you how to handle environmental data, create multi-axis plots, and use Matplotlib for weather analysis. - Student Performance Dashboard
Create a dashboard to visualize student performance data using Matplotlib. Plot average scores by subject, distribution of grades with histograms, and pass rates with bar charts. Add a feature to compare performance across classes. This project will help you learn how to visualize educational data, create statistical plots, and use Matplotlib for performance analysis. - Social Media Engagement Visualization
Visualize social media engagement data (e.g., likes, comments) using Matplotlib. Create line plots for engagement over time, bar charts for post types, and heatmaps for peak activity hours. Add annotations for viral posts. This project will teach you how to handle social media data, create heatmaps, and use Matplotlib for engagement analysis. - Healthcare Trends Visualization
Create visualizations for healthcare data (e.g., patient recovery rates) using Matplotlib. Plot recovery trends over time, bar charts for treatment outcomes, and scatter plots for correlations (e.g., age vs. recovery). Add a feature to highlight outliers. This project will help you learn how to visualize medical data, handle correlations, and use Matplotlib for health insights. - Energy Consumption Visualization
Visualize energy consumption data using Matplotlib. Create line plots for usage over time, bar charts for consumption by device, and pie charts for energy sources. Add a feature to compare usage across months. This project will teach you how to handle utility data, create comparative visualizations, and use Matplotlib for energy analysis. - Traffic Data Visualization
Create visualizations for traffic data (e.g., vehicle counts) using Matplotlib. Plot traffic volume over time, bar charts for peak hours, and heatmaps for congestion patterns. Add annotations for accidents or roadworks. This project will help you learn how to visualize transportation data, create temporal plots, and use Matplotlib for traffic analysis. - E-commerce Sales Visualization
Visualize e-commerce sales data using Matplotlib. Create line plots for sales trends, bar charts for top products, and pie charts for customer demographics. Add a feature to compare sales across regions. This project will teach you how to handle business data, create multi-category visualizations, and use Matplotlib for sales analysis. - Fitness Progress Visualization
Create visualizations for fitness data (e.g., workout logs) using Matplotlib. Plot progress over time (e.g., weight lifted), bar charts for workout types, and scatter plots for calorie burn. Add a feature to highlight personal bests. This project will help you learn how to visualize fitness data, create progress trackers, and use Matplotlib for personal analytics.
Database Management Projects (Python + SQLAlchemy)
- Student Management System
Build a system to manage student records using SQLAlchemy. Create models for students, courses, and grades, and store them in a database. Add features to register students, assign them to courses, and calculate GPAs. This project will teach you how to define database models, manage relationships (e.g., many-to-many for students and courses), and use SQLAlchemy for educational applications. - Inventory Management System
Create a system to manage inventory for a small business using SQLAlchemy. Define models for products, categories, and stock levels, and store them in a database. Add features to update stock, track sales, and generate low-stock alerts. This project will help you learn how to handle business data, implement CRUD operations, and use SQLAlchemy for inventory tracking. - Library Management System
Build a system to manage a library’s book inventory using SQLAlchemy. Create models for books, members, and borrowing records, and store them in a database. Add features to track book checkouts, returns, and fines. This project will teach you how to manage relational data, handle datetime fields, and use SQLAlchemy for library applications. - Event Registration System
Create a system to manage event registrations using SQLAlchemy. Define models for events, attendees, and registrations, and store them in a database. Add features to register attendees, send confirmation emails, and track attendance. This project will help you learn how to handle event data, manage relationships, and use SQLAlchemy for registration systems. - Expense Tracker
Build a system to track personal expenses using SQLAlchemy. Create models for expenses, categories, and users, and store them in a database. Add features to log expenses, categorize them, and generate monthly summaries. This project will teach you how to handle financial data, implement aggregations, and use SQLAlchemy for personal finance applications. - Healthcare Records System
Create a system to manage patient records using SQLAlchemy. Define models for patients, appointments, and treatments, and store them in a database. Add features to schedule appointments, record treatments, and track patient history. This project will help you learn how to handle medical data, manage sensitive information, and use SQLAlchemy for healthcare applications. - E-commerce Order Management
Build a system to manage e-commerce orders using SQLAlchemy. Create models for products, orders, and customers, and store them in a database. Add features to place orders, track order status, and generate sales reports. This project will teach you how to handle business transactions, manage order workflows, and use SQLAlchemy for e-commerce applications. - Task Management System
Create a system to manage tasks and projects using SQLAlchemy. Define models for tasks, projects, and users, and store them in a database. Add features to assign tasks, set deadlines, and track progress. This project will help you learn how to handle project data, manage user-task relationships, and use SQLAlchemy for task management. - Fitness Log System
Build a system to log fitness activities using SQLAlchemy. Create models for workouts, exercises, and users, and store them in a database. Add features to log workouts, track progress, and generate fitness reports. This project will teach you how to handle fitness data, manage time-series records, and use SQLAlchemy for personal tracking. - Voting System
Create a system to manage votes for an election using SQLAlchemy. Define models for candidates, voters, and votes, and store them in a database. Add features to cast votes, count results, and ensure voting integrity. This project will help you learn how to handle election data, prevent duplicate votes, and use SQLAlchemy for voting applications.
Game Development Projects (Python + Pygame)
- Snake Game
Build a classic Snake game using Pygame. Create a snake that moves around the screen, eats food to grow, and avoids hitting the walls or itself. Add a scoring system and a game-over screen. This project will teach you how to handle game loops, implement collision detection, and use Pygame for 2D game development. - Quiz Game
Create a quiz game where players answer multiple-choice questions using Pygame. Display questions on the screen, allow players to select answers with mouse clicks, and track their score. Add a timer for each question. This project will help you learn how to handle user input, create interactive UI elements, and use Pygame for educational games. - Space Invaders Clone
Build a simplified version of Space Invaders using Pygame. Create a player ship that shoots bullets, enemies that move across the screen, and a scoring system. Add a feature to increase difficulty over time. This project will teach you how to handle sprites, implement shooting mechanics, and use Pygame for action games. - Platformer Game
Create a 2D platformer game where a character jumps between platforms using Pygame. Add gravity, collectible items, and enemies to avoid. Include a level system with increasing difficulty. This project will help you learn how to implement physics (e.g., gravity, jumping), handle level design, and use Pygame for platformer games. - Tic-Tac-Toe with AI
Build a Tic-Tac-Toe game with an AI opponent using Pygame. Create a grid where players take turns placing X or O, and implement an AI using the minimax algorithm to play against the user. Add a win/lose screen. This project will teach you how to handle game logic, implement AI, and use Pygame for board games. - Pong Game
Create a two-player Pong game using Pygame. Build two paddles that players control with keyboard inputs, a ball that bounces between them, and a scoring system. Add sound effects for ball hits. This project will help you learn how to handle multiplayer mechanics, implement ball physics, and use Pygame for classic arcade games. - Maze Game
Build a maze game where the player navigates to the exit using Pygame. Generate a random maze, add a player character, and include collectibles or obstacles. Add a timer to track completion time. This project will teach you how to generate mazes, handle player movement, and use Pygame for puzzle games. - Flappy Bird Clone
Create a simplified version of Flappy Bird using Pygame. Build a bird that flaps through a series of pipes, avoiding collisions. Add a scoring system based on the number of pipes passed. This project will help you learn how to handle continuous movement, implement obstacle generation, and use Pygame for endless runner games. - Memory Matching Game
Build a memory matching game where players flip cards to find pairs using Pygame. Create a grid of cards, allow players to flip two cards at a time, and check for matches. Add a feature to track the number of moves. This project will teach you how to handle card mechanics, implement matching logic, and use Pygame for memory games. - Shooting Gallery Game
Create a shooting gallery game where players shoot at moving targets using Pygame. Build targets that move across the screen, a crosshair controlled by the mouse, and a scoring system. Add a time limit for each round. This project will help you learn how to handle mouse input, implement target movement, and use Pygame for shooting games.
Interactive Computing Projects (Python + Jupyter Notebook)
- Data Analysis Tutorial
Create an interactive tutorial for beginners to learn data analysis using Jupyter Notebook. Load a dataset (e.g., Iris), use Pandas to explore the data, and visualize it with Matplotlib. Add explanatory markdown cells and interactive widgets to filter data. This project will teach you how to create educational content, use Jupyter for interactive analysis, and combine code with documentation. - Stock Price Explorer
Build an interactive tool to explore stock prices using Jupyter Notebook. Load historical stock data, create visualizations with Matplotlib, and add widgets to select stocks or date ranges. Include calculations like moving averages. This project will help you learn how to create interactive financial tools, use Jupyter widgets, and visualize time-series data. - Weather Data Explorer
Create an interactive tool to explore weather data using Jupyter Notebook. Load a weather dataset, visualize temperature trends, and add widgets to filter by city or date. Include statistical summaries like average rainfall. This project will teach you how to handle environmental data, create interactive visualizations, and use Jupyter for data exploration. - Student Grade Analyzer
Build an interactive tool to analyze student grades using Jupyter Notebook. Load a dataset of grades, create visualizations (e.g., histograms), and add widgets to filter by subject or class. Include metrics like average scores. This project will help you learn how to handle educational data, create interactive dashboards, and use Jupyter for performance analysis. - Social Media Engagement Explorer
Create an interactive tool to explore social media engagement using Jupyter Notebook. Load a dataset of posts, visualize engagement metrics, and add widgets to filter by post type or date. Include heatmaps for peak activity. This project will teach you how to handle social media data, create interactive heatmaps, and use Jupyter for engagement analysis. - Healthcare Data Explorer
Build an interactive tool to explore healthcare data using Jupyter Notebook. Load a dataset of patient records, visualize recovery trends, and add widgets to filter by age or treatment. Include correlations between variables. This project will help you learn how to handle medical data, create interactive visualizations, and use Jupyter for health insights. - Energy Usage Analyzer
Create an interactive tool to analyze energy usage using Jupyter Notebook. Load a dataset of consumption, visualize usage trends, and add widgets to filter by device or time period. Include metrics like peak usage. This project will teach you how to handle utility data, create interactive plots, and use Jupyter for energy analysis. - Traffic Data Explorer
Build an interactive tool to explore traffic data using Jupyter Notebook. Load a dataset of vehicle counts, visualize traffic patterns, and add widgets to filter by hour or location. Include congestion heatmaps. This project will help you learn how to handle transportation data, create interactive visualizations, and use Jupyter for traffic analysis. - E-commerce Sales Explorer
Create an interactive tool to explore e-commerce sales using Jupyter Notebook. Load a dataset of sales, visualize trends, and add widgets to filter by product or region. Include metrics like average order value. This project will teach you how to handle business data, create interactive dashboards, and use Jupyter for sales analysis. - Fitness Progress Tracker
Build an interactive tool to track fitness progress using Jupyter Notebook. Load a dataset of workouts, visualize progress (e.g., weight lifted), and add widgets to filter by exercise or date. Include personal bests. This project will help you learn how to handle fitness data, create interactive trackers, and use Jupyter for personal analytics.
Mobile App Development Projects (Python + Kivy)
- Calculator App
Build a simple calculator app using Kivy. Create a user interface with buttons for digits and operations, and implement basic arithmetic functions (e.g., addition, subtraction). Add a display to show the input and results. This project will teach you how to create a UI with Kivy, handle user input, and build functional mobile apps. - Weather App
Create a weather app that displays forecasts for a given location using Kivy. Integrate a weather API (e.g., OpenWeatherMap) to fetch data, and design a UI to show temperature, humidity, and forecasts. Add a feature to search for cities. This project will help you learn how to integrate APIs, design mobile UIs, and use Kivy for weather apps. - To-Do List App
Build a to-do list app where users can add, edit, and delete tasks using Kivy. Create a UI with a text input for tasks and buttons for actions. Store tasks in a local file or database. Add a feature to mark tasks as completed. This project will teach you how to handle user interactions, manage data storage, and use Kivy for productivity apps. - Fitness Tracker App
Create a fitness tracker app where users can log workouts using Kivy. Design a UI to input exercise details (e.g., type, duration), and display a history of workouts. Add a feature to calculate calories burned. This project will help you learn how to design fitness UIs, handle user data, and use Kivy for health apps. - Expense Tracker App
Build an expense tracker app where users can log expenses using Kivy. Create a UI to input expense details (e.g., amount, category), and display a summary of spending. Add a feature to filter by category. This project will teach you how to handle financial data, design mobile interfaces, and use Kivy for personal finance apps. - Quiz App
Create a quiz app where users can answer multiple-choice questions using Kivy. Design a UI to display questions and options, and track the user’s score. Add a feature to show results at the end. This project will help you learn how to create interactive UIs, handle quiz logic, and use Kivy for educational apps. - Recipe App
Build a recipe app where users can browse and save recipes using Kivy. Create a UI to display recipe details (e.g., ingredients, steps), and add a feature to favorite recipes. Store data locally or fetch from an API. This project will teach you how to handle recipe data, design culinary UIs, and use Kivy for lifestyle apps. - Event Reminder App
Create an event reminder app where users can schedule events using Kivy. Design a UI to input event details (e.g., date, time), and display upcoming events. Add a feature to send notifications. This project will help you learn how to handle datetime data, design reminder UIs, and use Kivy for scheduling apps. - Music Player App
Build a simple music player app using Kivy. Create a UI to play, pause, and skip tracks, and display the current song. Add a feature to create playlists. This project will teach you how to handle audio playback, design media UIs, and use Kivy for entertainment apps. - Drawing App
Create a drawing app where users can draw on the screen using Kivy. Design a UI with a canvas for drawing, color options, and a clear button. Add a feature to save drawings. This project will help you learn how to handle touch input, design creative UIs, and use Kivy for drawing apps.
Big Data Processing Projects (Python + PySpark)
- Log Data Analysis
Analyze large-scale server log data to identify patterns (e.g., errors, peak traffic) using PySpark. Load the data into a Spark DataFrame, filter for specific events, and calculate metrics like error rates. Visualize the results with Matplotlib. This project will teach you how to handle log data, perform distributed processing, and use PySpark for big data analysis. - Recommendation System
Build a recommendation system for an e-commerce platform using PySpark. Use a dataset of user purchases, apply collaborative filtering (e.g., ALS algorithm), and recommend products to users. Evaluate the system’s performance with metrics like RMSE. This project will help you learn how to build recommendation systems, handle large datasets, and use PySpark for machine learning. - Traffic Data Processing
Process large-scale traffic data to identify congestion patterns using PySpark. Load the data into a Spark DataFrame, aggregate vehicle counts by hour, and calculate peak traffic times. Visualize the results with graphs. This project will teach you how to handle transportation data, perform aggregations, and use PySpark for big data processing. - Social Media Sentiment Analysis
Analyze social media posts to determine sentiment (positive, negative) using PySpark. Load a large dataset of posts, preprocess the text (e.g., tokenization), and apply a sentiment analysis model. Aggregate sentiment by topic or time. This project will help you learn how to handle social media data, perform NLP tasks, and use PySpark for sentiment analysis. - Energy Consumption Analysis
Process large-scale energy consumption data to identify usage patterns using PySpark. Load the data into a Spark DataFrame, calculate metrics like average usage per household, and identify peak times. Visualize the results with charts. This project will teach you how to handle utility data, perform distributed analytics, and use PySpark for energy insights. - Fraud Detection in Transactions
Build a system to detect fraudulent transactions in a large dataset using PySpark. Load transaction data, apply rules or a machine learning model to flag suspicious activities, and generate a report. This project will help you learn how to handle financial data, implement fraud detection logic, and use PySpark for security applications. - Healthcare Data Analysis
Analyze large-scale healthcare data (e.g., patient records) to identify trends using PySpark. Load the data into a Spark DataFrame, calculate metrics like average recovery time, and segment patients by demographics. Visualize the results. This project will teach you how to handle medical data, perform distributed analysis, and use PySpark for health insights. - E-commerce Sales Analysis
Process large-scale e-commerce sales data to identify trends using PySpark. Load the data into a Spark DataFrame, calculate metrics like total revenue per product, and segment sales by region. Visualize the results with charts. This project will help you learn how to handle business data, perform aggregations, and use PySpark for sales analysis. - Weather Data Processing
Analyze large-scale weather data to identify seasonal patterns using PySpark. Load the data into a Spark DataFrame, calculate metrics like average temperature per month, and identify anomalies. Visualize the results with graphs. This project will teach you how to handle environmental data, perform distributed processing, and use PySpark for weather analysis. - Customer Segmentation
Segment customers in a large dataset based on purchasing behavior using PySpark. Load the data into a Spark DataFrame, apply clustering (e.g., K-means), and analyze segments (e.g., high spenders). Visualize the results. This project will help you learn how to perform customer segmentation, apply machine learning, and use PySpark for marketing insights.
Conclusion
These project ideas span a wide range of applications, showcasing Python’s versatility across web development, data analysis, machine learning, and more. Experiment with these projects to build your skills, and explore additional resources like Python’s official documentation or online communities for further learning. Happy coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.