Print a-z Letters in CSharp
Dive into the basics of C# programming with our lesson on “Printing Letters from a to z.” We’ll guide you through the creation of a simple console program, highlighting the efficiency of loops. As we journey through the alphabet, you’ll grasp how loops simplify repetitive tasks in programming. This lesson is more than just printing letters; it’s a step towards mastering fundamental concepts that empower you to tackle challenges in C# development. Let’s uncover the simplicity and power of loops in this engaging learning experience.
Objectives
- Understanding Loop Statements:
- Objective: Introduce learners to the concept of loop statements in C#.
- Explanation: The primary goal is to help learners understand how loop statements work and how they can be utilized for repetitive tasks in programming.
- Hands-On Application of For Loop:
- Objective: Provide practical experience in using the for loop.
- Explanation: Through the task of printing letters from ‘a’ to ‘z,’ learners will gain hands-on experience with a fundamental loop construct in C#.
- Grasping Loop Initialization and Incrementation:
- Objective: Help learners understand the initialization and incrementation aspects of a loop.
- Explanation: By iterating through letters in a specified range, learners will comprehend how loop variables are initialized, conditionally evaluated, and incremented.
- Demonstrating the Role of Console Output:
- Objective: Showcase the importance of console output in C# console applications.
- Explanation: The lesson will demonstrate how to use Console.Write to print each letter on the same line and conclude with Console.ReadLine to keep the console window open.
- Reinforcing the Concept of Code Readability:
- Objective: Emphasize the importance of writing clear and readable code.
- Explanation: By guiding learners through the process of printing letters in a structured manner, the lesson aims to instill good coding practices, focusing on code readability.
- Encouraging Exploration of Loop Variations:
- Objective: Spark curiosity in learners to explore variations of loop statements.
- Explanation: While the lesson uses a for loop, learners are encouraged to explore other loop types such as while and do-while on their own, broadening their understanding of loop constructs.
- Promoting Application Beyond Basic Concepts:
- Objective: Encourage learners to think about how loop statements can be applied to solve real-world programming challenges.
- Explanation: While the primary focus is on printing letters, learners are prompted to consider how similar loop structures can be applied to address more complex scenarios in practical programming.
By the end of this lesson, learners should have a solid understanding of basic loop concepts in C# and be prepared to apply this knowledge to solve more complex problems using loop statements. The objectives aim to create a foundation for future exploration into more advanced programming concepts.
Source code example
using System; namespace PrintLettersApp { class Program { static void Main() { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Printing letters from a to z:"); for (char letter = 'a'; letter <= 'z'; letter++) { Console.Write(letter + " "); } Console.ReadLine(); // Keep the console window open } } }
Explanation
The program begins by displaying introductory messages. It then uses a for loop to iterate through the letters from ‘a’ to ‘z,’ printing each letter along with a space. Finally, the program waits for user input, keeping the console window open for the user to view the output. The code provides a simple demonstration of using a loop to achieve a repetitive task, printing letters in a structured manner.
Now, let us discuss the specifics:
- Namespace and Class:
- The code is organized within the PrintLettersApp namespace, providing a container for related code elements. (line 3)
- Inside the namespace, there’s a class named Program that contains the main logic of the application. (line 5)
- Main Method (line 7):
The Main method is the entry point of the program. It is automatically called when the program starts.
- Console Output (line 9-10):
These lines print informative messages to the console. The first line prints “iNetTutor.com,” and the second line prints “Printing letters from a to z.”
- For Loop (line 12-15):
- This for loop is responsible for iterating through the letters from ‘a’ to ‘z.’
- It starts with the initialization char letter = ‘a’, setting the loop variable to ‘a.’
- The loop continues as long as letter is less than or equal to ‘z’ (letter <= ‘z’).
- In each iteration, it prints the current letter followed by a space using Console.Write.
- Keeping the Console Open (line 17):
This line uses Console.ReadLine() to wait for user input, effectively keeping the console window open after printing the letters. This is commonly used to prevent the console window from closing immediately.
Output
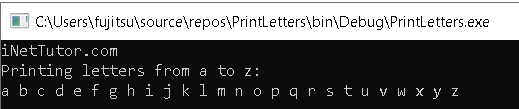
Summary
In this lesson, we embarked on a practical exploration of fundamental programming concepts in C#, focusing on the efficiency and versatility of loop statements. The provided code, contained within the PrintLettersApp namespace and the Program class, demonstrated the application of a for loop to print letters from ‘a’ to ‘z.’ Key takeaways from this lesson include an understanding of loop initialization, condition evaluation, and variable incrementation. Learners were encouraged to appreciate the importance of console output in C# console applications and to foster good coding practices for readability. By guiding users through the process of printing letters, the lesson laid a foundation for more advanced topics, encouraging exploration of various loop types and real-world applications. This practical hands-on experience sets the stage for learners to confidently apply loop constructs to solve diverse programming challenges in their C# journey.
Exercises and Assessment
Exercises:
- Reverse Order Printing:
- Modify the program to print letters from ‘z’ to ‘a’ instead of ‘a’ to ‘z.’ Utilize a loop for this task.
- Uppercase Letters:
- Enhance the program to print both lowercase and uppercase letters from ‘a’ to ‘z’ in alternating fashion. Use a single loop to achieve this.
- Customizable Range:
- Allow users to input two characters representing the start and end of the range (e.g., ‘c’ to ‘m’) and modify the program to print letters within this customized range.
- Interactive User Input:
- Instead of a fixed range, let users decide the number of letters to print. Prompt users to enter an integer, and print that many letters starting from ‘a.’
Assessment:
Objective: Evaluate learners’ proficiency in loop usage, code enhancement, and user interaction.
Criteria:
- Code Modification for Reverse Order (10 points):
- Award points based on the correct implementation of the program to print letters in reverse order (‘z’ to ‘a’).
- Inclusion of Uppercase Letters (15 points):
- Assess the ability to modify the code to print both lowercase and uppercase letters in alternating fashion. Award points for correctness and code readability.
- Customizable Range Implementation (20 points):
- Evaluate learners’ capability to implement user-defined ranges. Award points based on the correct modification of the program to print letters within a specified range.
- Interactive User Input Handling (15 points):
- Assess the implementation of user interaction for specifying the number of letters to print. Award points for a functional and user-friendly approach.
- Code Readability and Comments (10 points):
- Consider code readability and the presence of meaningful comments. Award points for well-documented and understandable code.
- Bonus Challenge: Additional Enhancements (10 points):
- Encourage learners to propose and implement additional enhancements to the program, showcasing creativity and deeper understanding.
Total Points: 80 (with a bonus of 10 points)
This assessment is designed to evaluate learners’ ability to not only modify existing code but also enhance it with additional features, fostering a deeper understanding of loop constructs and user interaction in C# programming.
Related Topics and Articles:
C# For Loop Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.