Overwrite Variable value in C#
Introduction
Table of Contents
In C#, variables are fundamental to storing and manipulating data within a program. Understanding how to overwrite variable values is crucial for dynamic and responsive applications. This tutorial will guide you through the process of assigning new values to existing variables, demonstrating the flexibility and control you have over data manipulation in C#. Whether you’re updating user inputs, recalculating values, or simply altering the state of your program, mastering variable overwriting will enhance your coding efficiency and problem-solving skills. Join us as we explore the practical applications and best practices for overwriting variable values in C#.
Objectives
In this lesson, we will focus on the crucial skill of overwriting variable values in C#. By the end of this tutorial, you will have a solid understanding of how to update variable values effectively, which is essential for writing dynamic and efficient C# programs. Our objectives will ensure you not only grasp the concept but also practice and apply it in various scenarios.
- Understand: Comprehend the concept of variables in C# and the importance of being able to overwrite their values.
- Learn: Acquire the knowledge of different methods and best practices for updating variable values in C#.
- Practice: Engage in hands-on coding exercises to reinforce your ability to overwrite variable values accurately.
- Apply: Implement the skills learned to create responsive and adaptive programs by effectively managing variable values.
By focusing on these objectives, you will develop a comprehensive skill set that will allow you to manage and manipulate data within your C# applications efficiently.
Source code example
using System; namespace OverwritingVariables { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com - Overwrite variables"); // Declaring a variable with an initial value int age = 25; Console.WriteLine("Initial age: " + age); // Overwriting the value of the variable age = 30; Console.WriteLine("Updated age: " + age); // Declaring another variable and overwriting it multiple times string name = "Alice"; Console.WriteLine("Name: " + name); name = "Bob"; Console.WriteLine("New name: " + name); name = "Charlie"; Console.WriteLine("Final name: " + name); Console.ReadLine(); } } }
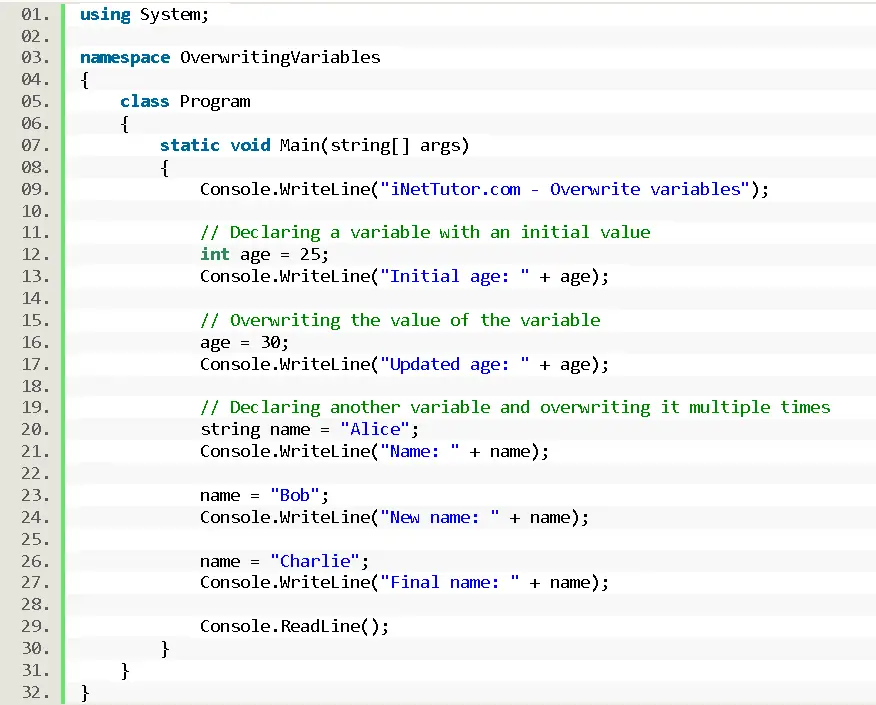
Explanation
The provided code demonstrates the concept of overwriting variables in C#. Let’s break it down:
- The code begins with the necessary using System; statement, which allows us to use classes and methods from the System namespace.
- Inside the Main method, we start by printing a message to the console using Console.WriteLine() to indicate the purpose of the program.
- Next, we declare an integer variable called age and assign it an initial value of 25. We then display the initial value of age using Console.WriteLine().
- We proceed to overwrite the value of the age variable by assigning it a new value of 30. The updated value is then printed to the console.
- Following that, we declare a string variable called name and assign it the value “Alice”. We display the initial value of name using Console.WriteLine().
- We proceed to overwrite the value of the name variable multiple times. First, we assign it the value “Bob” and then “Charlie”. After each assignment, we print the updated value of name to the console.
- Finally, we use Console.ReadLine() to wait for user input before closing the console window.
This code provides a simple and beginner-friendly example of how to overwrite variable values in C#. It demonstrates the process of declaring variables, assigning initial values, and updating those values as needed.
Output
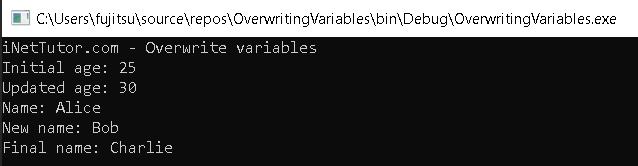
Summary
In this lesson, we explored the concept of overwriting variable values in C#. We learned how to declare and initialize variables, and then update their values during the execution of a program. The example program demonstrated these concepts by initializing an integer variable age and a string variable name, and then overwriting these variables with new values. The program printed the values of these variables at each step to show the changes. This lesson highlights the importance of understanding variable assignment and reassignment, which is fundamental in programming for managing and manipulating data dynamically. Through this exercise, we gained practical experience with basic variable operations, providing a foundation for more complex data handling in future programming tasks.
Exercises and Assessment
To solidify your understanding of variable assignment and overwriting in C#, it is important to practice these concepts through targeted exercises and assessments. The following exercises and assessments are designed to help you grasp the fundamentals of variable manipulation, enhance your coding skills, and prepare you for more complex programming tasks. By engaging in these activities, you will gain confidence in managing variables, understanding their scope and lifetime, and applying these skills to real-world scenarios.
Exercises
- Exercise 1: Basic Variable Overwriting
- Declare an integer variable with an initial value.
- Print the initial value.
- Overwrite the variable with a new value.
- Print the updated value.
- Exercise 2: Multiple Overwrites
- Declare a string variable and initialize it with a name.
- Overwrite the variable with three different names in sequence.
- Print the variable’s value after each overwrite.
- Exercise 3: Using Different Data Types
- Declare and initialize variables of different data types (e.g., int, float, char, bool).
- Overwrite each variable with a new value.
- Print the initial and updated values for each variable.
- Exercise 4: User Input and Variable Overwriting
- Prompt the user to enter their age and store it in an integer variable.
- Print a message displaying the entered age.
- Prompt the user to enter a new age and overwrite the variable.
- Print a message displaying the updated age.
Quiz
1. Remembering: Which of the following is the correct syntax to declare an integer variable in C#?
a) int number = 10;
b) integer number = 10;
c) var number = 10;
d) float number = 10;
2. Understanding: What is the purpose of overwriting a variable’s value in C#? 00
a) To declare a new variable
b) To assign an initial value to a variable
c) To update the value of an existing variable
d) To delete a variable from memory
3. Analyzing: Consider the following code snippet:
int x = 5; int y = 10; x = y; y = 15;
What will be the final value of x after executing this code?
a) 5
b) 10
c) 15
d) 20
4. Which of the following statements about variable overwriting is correct?
a) You can overwrite a const variable.
b) You cannot overwrite a variable once it’s declared.
c) You can overwrite a variable as many times as needed.
d) Overwriting a variable requires the use of the ref keyword.
5. When a variable’s value is overwritten, what happens to the original value?
a) It is permanently deleted.
b) It is stored in a temporary memory location.
c) It is replaced by the new value.
d) It remains unchanged.
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.