MDAS Example in C#
Introduction
Table of Contents
MDAS (Multiplication, Division, Addition, and Subtraction) is a crucial concept in programming that dictates the order of operations for arithmetic expressions. In C#, as in most programming languages, operations are performed according to MDAS rules to ensure accurate results. This guide will walk you through a straightforward example in C# that demonstrates how MDAS works in practice. Whether you’re a beginner or looking to refresh your understanding, this example will clarify how to correctly apply MDAS rules in your C# code.
Objectives
In this guide, our primary goal is to deepen your understanding of the MDAS (Multiplication, Division, Addition, and Subtraction) order of operations in C#. We aim to ensure that you grasp the concept, learn how to implement it, practice through examples, and apply your knowledge effectively in your own C# projects. Here’s a breakdown of our objectives:
- Understand: Gain a clear understanding of the MDAS rules and their significance in arithmetic operations. This foundation will help you comprehend how different operations interact within expressions.
- Learn: Learn how to implement MDAS principles in C# code. We’ll cover syntax and the order of operations to ensure your calculations are performed correctly.
- Practice: Engage in hands-on practice with various examples that demonstrate the application of MDAS rules in C#. This practice will solidify your understanding and improve your coding skills.
- Apply: Apply your knowledge of MDAS to solve real-world problems and write accurate C# code. This final step will help you integrate MDAS principles into your development workflow and projects.
By focusing on these objectives, you’ll be well-equipped to handle arithmetic operations in C# with confidence and precision.
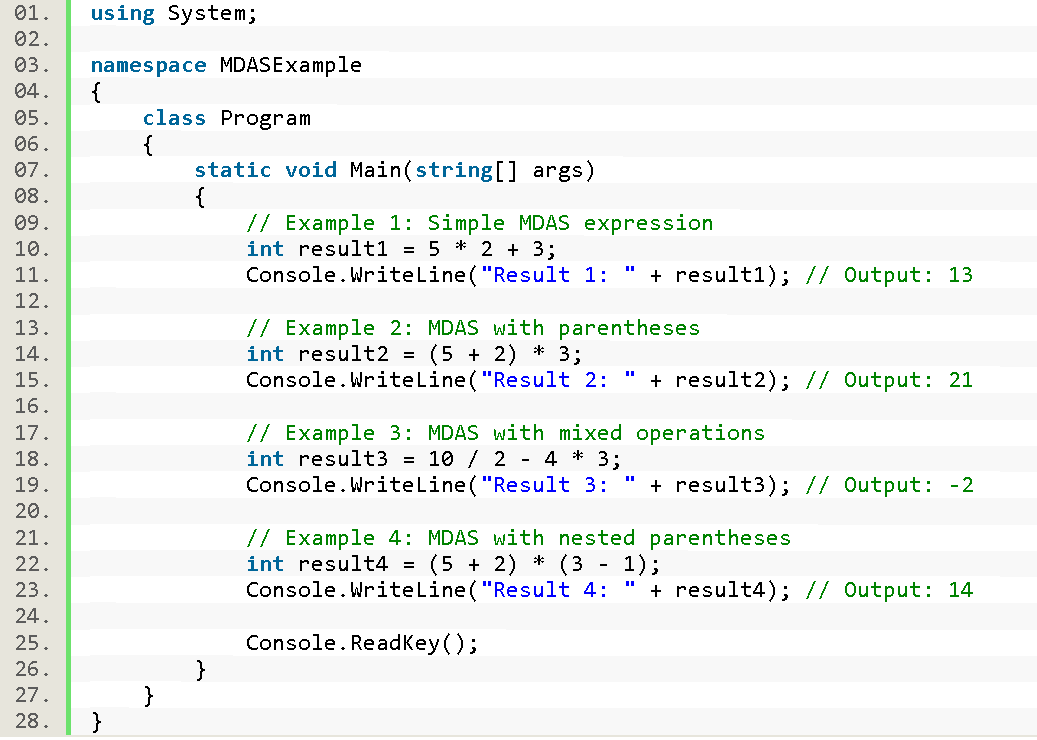
Source code example
using System; namespace MDASExample { class Program { static void Main(string[] args) { // Example 1: Simple MDAS expression int result1 = 5 * 2 + 3; Console.WriteLine("Result 1: " + result1); // Output: 13 // Example 2: MDAS with parentheses int result2 = (5 + 2) * 3; Console.WriteLine("Result 2: " + result2); // Output: 21 // Example 3: MDAS with mixed operations int result3 = 10 / 2 - 4 * 3; Console.WriteLine("Result 3: " + result3); // Output: -2 // Example 4: MDAS with nested parentheses int result4 = (5 + 2) * (3 - 1); Console.WriteLine("Result 4: " + result4); // Output: 14 Console.ReadKey(); } } }
Explanation
- Namespace and Class Declaration:
- using System;: Imports the System namespace, which contains basic classes like Console.
- namespace MDASExample: Defines a namespace to organize code.
- class Program: Declares a class named Program.
- Main Method:
- static void Main(string[] args): The entry point of the program. It’s where execution begins.
- Example 1: Simple MDAS Expression:
- int result1 = 5 * 2 + 3;
- The expression 5 * 2 + 3 is evaluated using MDAS rules.
- Multiplication is performed first: 5 * 2 = 10.
- Addition is then performed: 10 + 3 = 13.
- Console.WriteLine(“Result 1: ” + result1);
- Outputs Result 1: 13.
- int result1 = 5 * 2 + 3;
- Example 2: MDAS with Parentheses:
- int result2 = (5 + 2) * 3;
- Parentheses change the order of operations.
- Addition inside the parentheses: 5 + 2 = 7.
- Multiplication is then performed: 7 * 3 = 21.
- Console.WriteLine(“Result 2: ” + result2);
- Outputs Result 2: 21.
- int result2 = (5 + 2) * 3;
- Example 3: MDAS with Mixed Operations:
- int result3 = 10 / 2 – 4 * 3;
- Division is performed first: 10 / 2 = 5.
- Multiplication is next: 4 * 3 = 12.
- Subtraction is performed last: 5 – 12 = -7.
- Console.WriteLine(“Result 3: ” + result3);
- Outputs Result 3: -7.
- int result3 = 10 / 2 – 4 * 3;
- Example 4: MDAS with Nested Parentheses:
- int result4 = (5 + 2) * (3 – 1);
- Addition inside the first set of parentheses: 5 + 2 = 7.
- Subtraction inside the second set of parentheses: 3 – 1 = 2.
- Multiplication of the results: 7 * 2 = 14.
- Console.WriteLine(“Result 4: ” + result4);
- Outputs Result 4: 14.
- int result4 = (5 + 2) * (3 – 1);
- Console.ReadKey();:
- Waits for a key press before closing the console window. This allows you to see the results before the program exits.
This code provides a practical demonstration of how to apply MDAS rules and parentheses to control the order of operations in arithmetic expressions in C#.
Output
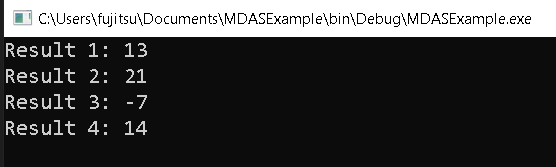
Summary
In this lesson, we explored the MDAS (Multiplication, Division, Addition, and Subtraction) order of operations in C# through a series of straightforward examples. We demonstrated how to correctly evaluate arithmetic expressions using MDAS rules, with and without parentheses, to control the order of operations. By examining various scenarios, including simple expressions and those involving nested parentheses, we illustrated how these principles ensure accurate calculations in C# programming. This foundational understanding is essential for writing correct and effective arithmetic code.
Exercises and Assessment
To reinforce your understanding of the MDAS rule and its application in C#, we have prepared a series of exercises, an assessment, and a lab exam. These activities will challenge you to solve various MDAS-related problems and apply your knowledge in different scenarios.
Exercises:
- Evaluate Expressions: Given a set of C# expressions, determine the correct result based on the MDAS rule.
- Write Expressions: Write C# expressions to represent mathematical equations involving MDAS.
- Error Correction: Identify and correct errors in C# code that violate the MDAS rule.
- Real-World Applications: Apply MDAS to solve real-world problems, such as calculating compound interest or determining the total cost of a purchase with taxes and discounts.
Assessment:
Create a C# program that simulates a simple calculator. The calculator should allow users to input two numbers and perform basic arithmetic operations (addition, subtraction, multiplication, division, and modulus). Ensure that the program adheres to the MDAS rule for correct calculations.
Lab Exam:
Design and code a program that calculates the area and perimeter of different shapes (e.g., rectangle, circle, triangle). Use the MDAS rule to ensure accurate calculations and handle potential edge cases.
Tips for Success:
- Break down complex expressions into smaller, manageable parts.
- Use parentheses to clarify the order of operations when necessary.
- Test your code thoroughly with different input values.
- Refer to the tutorial and provided code examples for guidance.
- Seek assistance from your instructor or classmates if needed.
By completing these exercises and assessments, you will gain practical experience and solidify your understanding of the MDAS rule in C#.
Quiz
1. What is the correct order of operations in C# according to the MDAS rule?
A. Addition, Subtraction, Multiplication, Division
B. Multiplication, Division, Addition, Subtraction
C. Addition, Multiplication, Subtraction, Division
D. Multiplication, Addition, Subtraction, Division
2. Which of the following C# expressions will evaluate to 15?
A. 5 * 2 + 3
B. (5 + 2) * 3
C. 10 / 2 – 4 * 3
D. (5 + 2) * (3 – 1)
3. Given the expression (10 – 3) * (2 + 4) / 2, what is the correct result?
A. 21
B. 14
C. 7
D. 35
4. In the following C# code, how does the placement of parentheses affect the result?
int result1 = 5 * 2 + 3; int result2 = (5 * 2) + 3;
A. Both expressions result in the same value.
B. `result1` is greater than `result2`.
C. `result2` is greater than `result1`.
D. The results cannot be determined without knowing the values of `result1` and `result2`.
5. Which of the following expressions correctly follows the MDAS rules?
A. 8 + 4 * 2
B. 8 + (4 * 2)
C. 4 * (8 + 2)
D. (8 + 4) * 2
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.