Mathematical Operations using Variables in C#
Introduction
In this tutorial, we’ll explore how to perform mathematical operations with variables in C#. Understanding how to manipulate data through basic arithmetic is essential for building functional programs. We’ll cover addition, subtraction, multiplication, division, and modulus, using variables to store and compute values. By the end of this lesson, you’ll know how to apply these operations in C# to create more interactive and dynamic applications. Perfect for beginners looking to strengthen their coding skills.
Objectives
In this lesson, you will learn the fundamental concepts of mathematical operations with variables in C#. By focusing on both theoretical understanding and hands-on practice, this tutorial will help you gain practical skills to apply these concepts in real-world coding scenarios. The objectives of this tutorial are structured around key learning outcomes to ensure a comprehensive grasp of arithmetic operations in C# programming.
Objective 1: Understand Mathematical Operations in C#
- Grasp the core mathematical operations (addition, subtraction, multiplication, division, modulus) and how they interact with variables.
Objective 2: Learn to Work with Numeric Data Types
- Recognize how different data types (int, double, float) handle arithmetic operations and when to use each.
Objective 3: Practice Using Operators with Variables
- Perform various mathematical operations by writing code that manipulates variables and produces accurate results through calculations.
Objective 4: Apply Mathematical Operations in Programs
- Implement mathematical operations in real-world programming examples, such as building a simple calculator or solving arithmetic problems.
By focusing on these objectives, you will build a solid foundation in working with mathematical operations in C# and be ready to tackle more complex coding challenges.
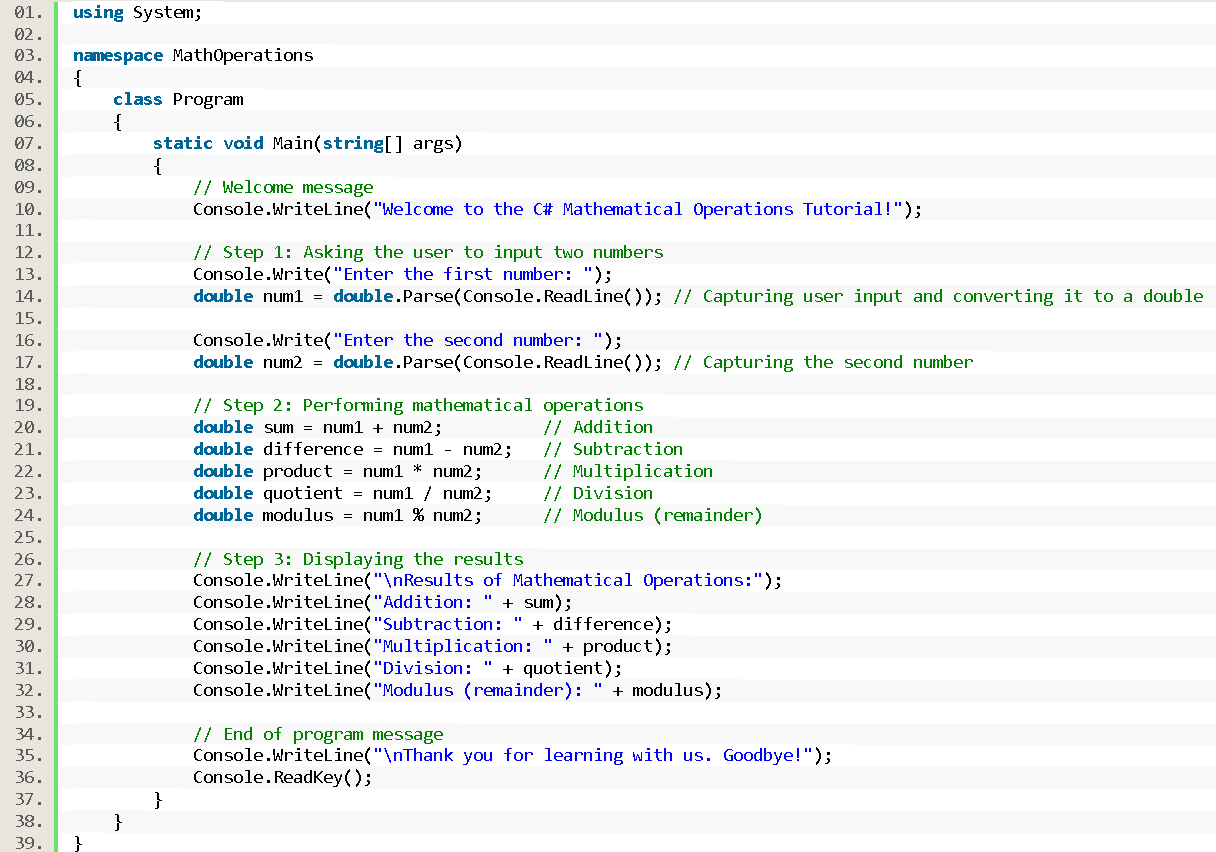
Source code example
using System; namespace MathOperations { class Program { static void Main(string[] args) { // Welcome message Console.WriteLine("Welcome to the C# Mathematical Operations Tutorial!"); // Step 1: Asking the user to input two numbers Console.Write("Enter the first number: "); double num1 = double.Parse(Console.ReadLine()); // Capturing user input and converting it to a double Console.Write("Enter the second number: "); double num2 = double.Parse(Console.ReadLine()); // Capturing the second number // Step 2: Performing mathematical operations double sum = num1 + num2; // Addition double difference = num1 - num2; // Subtraction double product = num1 * num2; // Multiplication double quotient = num1 / num2; // Division double modulus = num1 % num2; // Modulus (remainder) // Step 3: Displaying the results Console.WriteLine("\nResults of Mathematical Operations:"); Console.WriteLine("Addition: " + sum); Console.WriteLine("Subtraction: " + difference); Console.WriteLine("Multiplication: " + product); Console.WriteLine("Division: " + quotient); Console.WriteLine("Modulus (remainder): " + modulus); // End of program message Console.WriteLine("\nThank you for learning with us. Goodbye!"); Console.ReadKey(); } } }
Explanation
This program takes two numbers as input from the user, performs basic mathematical operations (addition, subtraction, multiplication, division, modulus), and then displays the results. It’s a simple introduction to working with user input, variables, and basic arithmetic in C#.
- Namespace:
- MathOperations: This defines the namespace where the code resides. It helps organize and avoid naming conflicts with other code.
- Class:
- Program: This is the main class where the execution of the program starts.
- Main Method:
- This is the entry point of the program. It’s where the code logic is executed.
- Welcome Message:
- Console.WriteLine(“Welcome to the C# Mathematical Operations Tutorial!”);: This line prints a welcome message to the console, greeting the user.
- User Input:
- Console.Write(“Enter the first number: “);: This line prompts the user to enter the first number.
- double num1 = double.Parse(Console.ReadLine());: This line reads the user’s input from the console using Console.ReadLine() and converts it to a double data type using double.Parse(). This allows for decimal values.
- Similar steps are followed for the second number.
- Mathematical Operations:
- double sum = num1 + num2;: This line calculates the sum of the two numbers and stores it in the sum variable.
- Similar calculations are performed for subtraction, multiplication, division, and modulus using the appropriate operators.
- Displaying Results:
- Console.WriteLine(“\nResults of Mathematical Operations:”);: This line prints a new line and a heading to separate the results.
- Console.WriteLine(“Addition: ” + sum);: This line prints the result of the addition operation, along with a label.
- Similar lines are used to print the results of other operations.
- End Message:
- Console.WriteLine(“\nThank you for learning with us. Goodbye!”);: This line prints a farewell message to the user.
- Console.ReadKey();: This line pauses the program execution until the user presses a key, allowing the output to remain visible.
Output
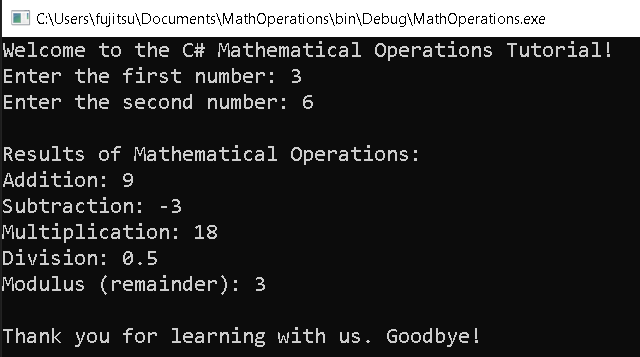
Summary
In this lesson, we explored how to perform basic mathematical operations using variables in C#. By taking user input and applying arithmetic operations such as addition, subtraction, multiplication, division, and modulus, we demonstrated how to manipulate data and display the results in a console application. This hands-on approach provided a clear understanding of how to work with numeric variables and operators in C#, making it an essential foundational skill for beginners in programming.
Exercises and Assessment
To reinforce your understanding of mathematical operations in C# and to enhance your coding skills, we have prepared a series of exercises, an assessment, and a lab exam. These activities will challenge you to apply the concepts learned in this tutorial in various scenarios.
Exercises:
- Temperature Conversion: Write a program that converts temperature from Fahrenheit to Celsius and vice versa. Allow the user to input the temperature and the desired unit of conversion.
- Area and Perimeter Calculator: Create a program that calculates the area and perimeter of different shapes (e.g., rectangle, circle, triangle). Prompt the user to choose a shape and enter the required dimensions.
- Grade Calculator: Develop a program that calculates a student’s average grade based on multiple assignments. Allow the user to input the scores for each assignment and determine the final grade.
Assessment:
Implement a program that calculates the compound interest on a given principal amount, interest rate, and time period. The program should provide options for different compounding frequencies (e.g., annually, semi-annually, quarterly, monthly).
Lab Exam:
Design and code a program that simulates a simple calculator. The calculator should allow users to perform basic arithmetic operations (addition, subtraction, multiplication, division) and calculate the square root of a number. Implement error handling to prevent invalid inputs.
Tips for Success:
- Break down complex problems into smaller, manageable steps.
- Use meaningful variable names to improve code readability.
- Test your code thoroughly with different input values.
- Refer to the tutorial and provided code examples for guidance.
- Seek assistance from your instructor or classmates if needed.
By completing these exercises and assessments, you will gain practical experience and solidify your understanding of mathematical operations in C#.
Quiz
1. What is the correct operator for addition in C#?
A. *
B. –
C. +
D. /
2. Which of the following best describes the modulus operator (%) in C#?
A. It divides two numbers and returns the result.
B. It subtracts the second number from the first number.
C. It multiplies two numbers together.
D. It divides two numbers and returns the remainder.
3. How would you modify the program to handle division by zero without crashing?
A. Remove the division operation from the code.
B. Add an if condition to check if the divisor is zero and display an error message.
C. Use the modulus operator instead of the division operator.
D. Perform the division first, then handle the error afterwards.
4. Question: Given two integer variables num1 and num2, write a C# expression to calculate their average.
A. (num1 + num2) / 2
B. num1 / num2
C. num1 * num2
D. num1 – num2
5. Question: What is the correct operator for division in C#?
A. /
B. //
C. %
D. \
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.