Linked Lists and File Handling in C#
Introduction
Table of Contents
Linked lists and file handling are essential concepts in C# programming, widely used in data management and storage applications. A linked list is a dynamic data structure that allows efficient insertion and deletion of elements, making it ideal for scenarios where data size is unpredictable. On the other hand, file handling enables reading from and writing to files, ensuring data persistence beyond program execution.
In this lesson, you’ll learn how to implement linked lists in C# and perform file operations such as reading, writing, and appending data. Whether you’re managing real-time data structures or working with external files, mastering these concepts will enhance your ability to build efficient and scalable applications. Let’s get started!
Objectives
In this lesson, we aim to provide you with a clear and structured path to understanding two essential programming concepts in C#: Linked Lists and File Handling. By breaking down the learning process into four key objectives—Understand, Learn, Practice, and Apply—we ensure you gain both theoretical knowledge and hands-on experience. Whether you’re a beginner or looking to refine your skills, these objectives will guide you in building a strong foundation and applying these concepts to real-world scenarios. Let’s explore what each objective entails:
- Understand: Grasp the core concepts of linked lists and file handling, including their purpose, structure, and use cases in C#.
- Learn: Dive into the syntax and methods required to implement linked lists and perform file operations like reading, writing, and appending data.
- Practice: Reinforce your understanding through coding exercises and examples that simulate real-world problems.
- Apply: Use your newfound skills to create a simple project that combines linked lists and file handling, showcasing your ability to integrate these concepts effectively.
By the end of this lesson, you’ll not only understand the theory but also feel confident in applying these concepts to your own C# projects. Let’s get started!
Source code example
using System; using System.Collections.Generic; using System.IO; namespace LinkedListAndFiles { class Program { // Define a Node class for the linked list class Node { public string Data; // Data stored in the node public Node Next; // Reference to the next node public Node(string data) { Data = data; Next = null; } } // Define a LinkedList class to manage nodes class LinkedList { public Node Head; // Starting point of the linked list // Add a new node to the end of the linked list public void AddNode(string data) { Node newNode = new Node(data); if (Head == null) { Head = newNode; // If the list is empty, set the new node as the head } else { Node current = Head; while (current.Next != null) { current = current.Next; // Traverse to the end of the list } current.Next = newNode; // Add the new node at the end } } // Print all nodes in the linked list public void PrintList() { Node current = Head; while (current != null) { Console.WriteLine(current.Data); current = current.Next; } } } static void Main(string[] args) { // Step 1: Create a linked list and add some data LinkedList myList = new LinkedList(); myList.AddNode("Apple"); myList.AddNode("Banana"); myList.AddNode("Cherry"); Console.WriteLine("Linked List Contents:"); myList.PrintList(); // Step 2: Save the linked list data to a file string filePath = "linkedlist_data.txt"; SaveLinkedListToFile(myList, filePath); Console.WriteLine($"\nData saved to {filePath}"); // Step 3: Read the data from the file and rebuild the linked list LinkedList newList = ReadLinkedListFromFile(filePath); Console.WriteLine("\nRebuilt Linked List Contents:"); newList.PrintList(); Console.ReadLine(); } // Save the linked list data to a file static void SaveLinkedListToFile(LinkedList list, string filePath) { using (StreamWriter writer = new StreamWriter(filePath)) { Node current = list.Head; while (current != null) { writer.WriteLine(current.Data); // Write each node's data to the file current = current.Next; } } } // Read the data from the file and rebuild the linked list static LinkedList ReadLinkedListFromFile(string filePath) { LinkedList list = new LinkedList(); using (StreamReader reader = new StreamReader(filePath)) { string line; while ((line = reader.ReadLine()) != null) { list.AddNode(line); // Add each line from the file as a new node } } return list; } } }
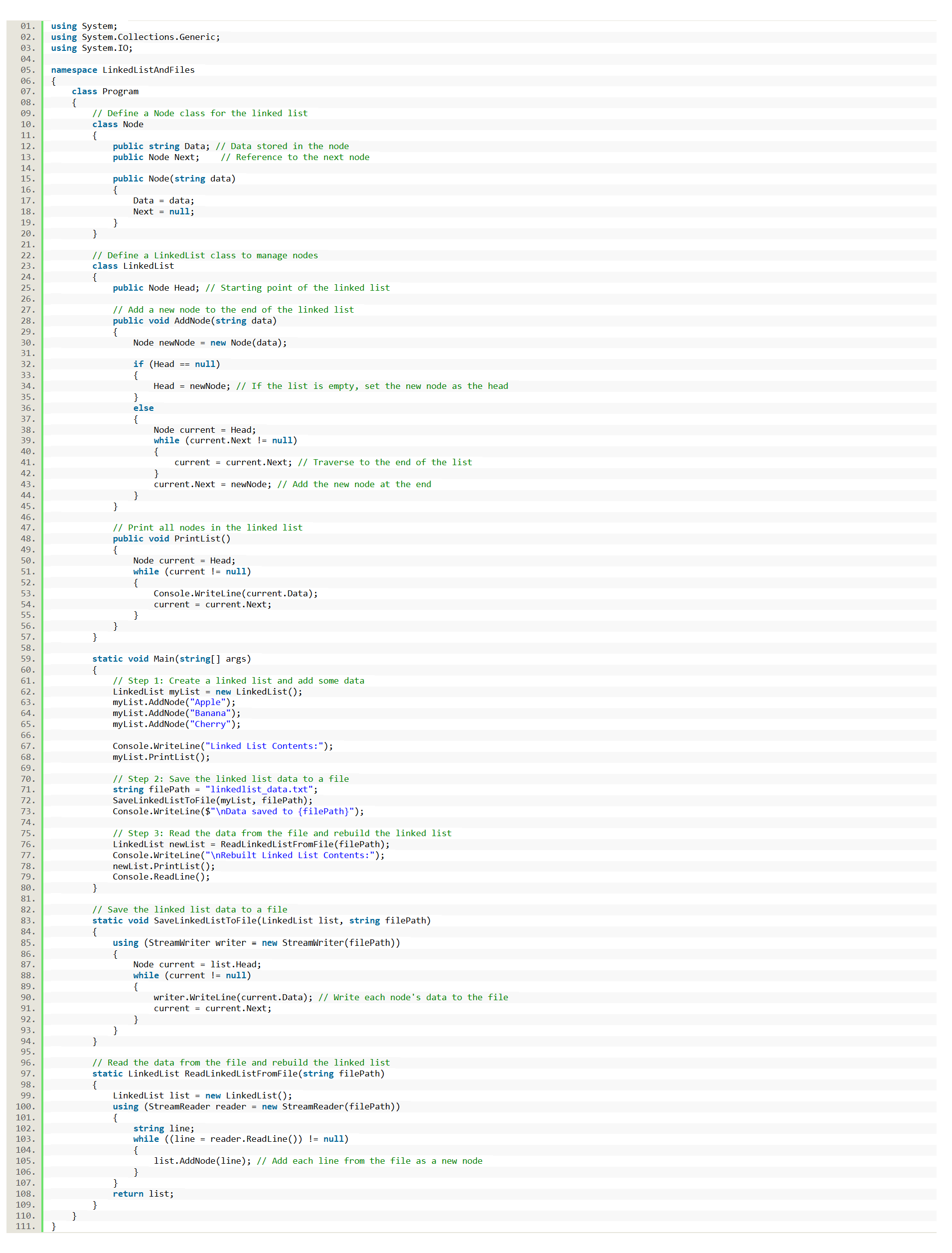
Explanation
- Node Class:
- Represents a single node in the linked list.
- Contains Data (a string) and a reference to the Next node.
- LinkedList Class:
- Manages the linked list.
- Includes methods to add nodes (AddNode) and print the list (PrintList).
- File Handling:
- SaveLinkedListToFile: Writes the linked list data to a text file.
- ReadLinkedListFromFile: Reads the data from the file and rebuilds the linked list.
- Main Method:
- Demonstrates creating a linked list, saving it to a file, and rebuilding it from the file.
Example Output
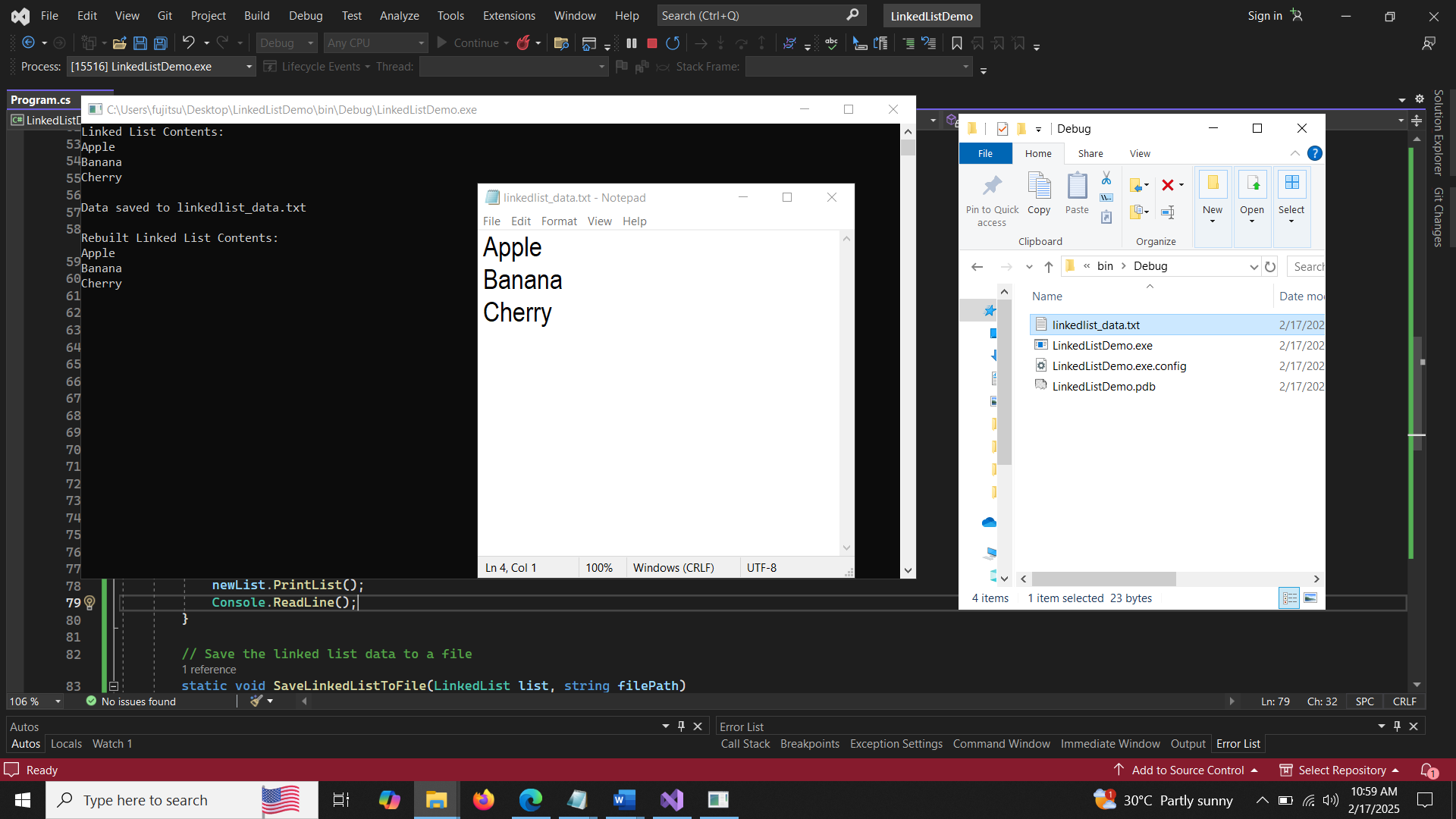
Why This Code is Beginner-Friendly
- Simple Structure: The code is modular, with clear separation of concerns (e.g., Node, LinkedList, file handling).
- Comments: Each step is explained with comments to help beginners follow along.
- Real-World Application: Combines two key concepts (linked lists and file handling) in a practical way.
This code is a great starting point for beginners to understand how linked lists and file handling work together in C#!
Summary
In this lesson, you learned the fundamentals of Linked Lists and File Handling in C#. The linked list was introduced as a dynamic data structure that allows efficient insertion and deletion of elements, with a simple implementation that includes adding and displaying data. You also explored file handling techniques, including writing linked list data to a file and reading it back for display. Through practical coding examples, you gained hands-on experience in managing data with linked lists and saving and retrieving it using file operations, forming a strong foundation for building more advanced data-driven applications.
Lab Exam: Improving the Source Code
In this lab exam, you’ll have the opportunity to apply what you’ve learned in the previous lesson by improving the source code for linked lists and file handling in C#. This exercise will help you enhance your skills in structuring code, implementing additional features, and applying best practices in coding. You’ll work on optimizing the linked list implementation, adding more file handling features, and refining the program to handle edge cases. The objective is to build a more robust, efficient, and user-friendly program.
Exercises:
- Linked List Enhancement:
- Modify the LinkedList class to include a method that removes a specific element from the list. If the element does not exist, display an appropriate message.
- Add a method to search for an element in the list and return its position (index). If the element is not found, return a message indicating so.
- File Handling Improvement:
- Implement error handling for file operations. If the program cannot open or read/write to the file, display a helpful error message to the user.
- Add a feature to append new linked list data to the file instead of overwriting the existing data each time the program runs.
- Validation and User Input:
- Add validation for user input when adding elements to the linked list. Ensure that the user inputs only valid integers. If an invalid input is provided, ask the user to enter a valid integer.
- Implement a menu-driven interface that allows the user to choose actions (e.g., Add Element, Remove Element, Search, Display List, Save to File, Load from File).
- File Loading Feature:
- Implement a method to load the linked list from the file, allowing the program to reconstruct the list when it starts. The file should contain the previously saved data, and the program should read the data to populate the linked list.
Submission:
Submit your improved source code after making the changes. Ensure that your program compiles and runs as expected, with the following features:
- User-friendly input and output
- Error handling for file operations
- Enhanced linked list operations (add, remove, search)
- File reading and writing functionality
By completing this lab exam, you’ll gain valuable experience in working with more advanced concepts like dynamic data structures, file handling, and user interaction in C#, allowing you to build more efficient and maintainable applications.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.