Introduction to Data Structures in Computer Programming
Introduction
Table of Contents
What Are Data Structures?
Data structures are specialized formats for organizing, managing, and storing data in a computer system. They allow efficient data access and modification, making it easier to perform various operations like searching, sorting, and updating information. Common examples include arrays, linked lists, stacks, queues, trees, and graphs.
Why Are Data Structures Important in Computer Programming?
Data structures play a crucial role in software development by enhancing data organization and efficiency. Here’s why they matter:
- Efficient Data Handling: Proper data structures improve data storage and retrieval, reducing execution time.
- Optimized Performance: Algorithms rely on data structures for faster processing and minimal resource consumption.
- Better Problem-Solving: Choosing the right data structure simplifies complex programming challenges.
- Memory Management: Data structures help in optimizing memory usage, preventing wastage.
How Data Structures Improve Efficiency
Using the right data structure can significantly impact a program’s performance. Here’s how:
- Faster Search and Retrieval: Hash tables and trees allow quick lookups, enhancing system speed.
- Reduced Computational Complexity: Sorting and searching algorithms work efficiently with well-structured data.
- Scalability: Proper data structures handle large datasets efficiently, making applications scalable.
Understanding data structures is essential for any programmer, as they form the foundation for writing optimized and scalable code.
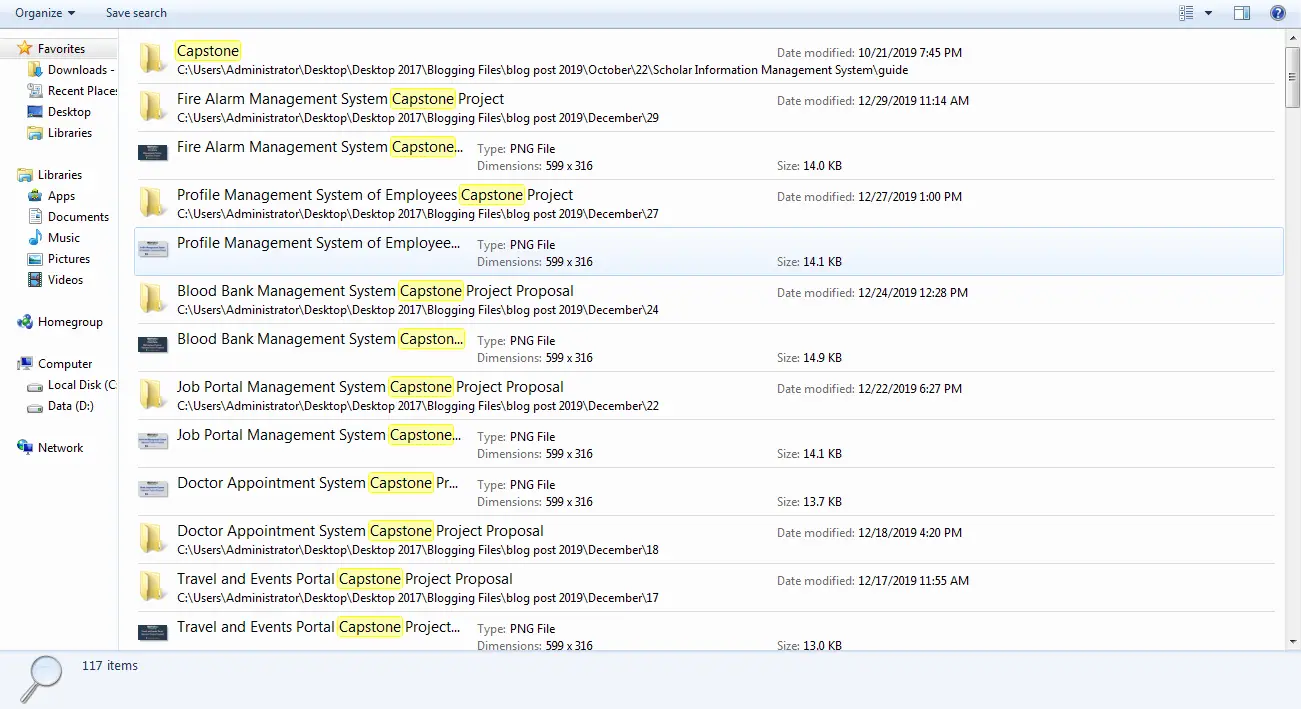
Fundamental Concepts of Data Structures
Understanding the core concepts of data structures is essential for any aspiring programmer. Let’s break down the fundamental elements that underpin how we organize and manipulate data.
- Data and Information: The Building Blocks
- Difference between Data and Information:
- Data is raw, unprocessed facts and figures. It’s the “what” – the individual pieces of information. Think of it as a collection of numbers, text, or symbols without context. For example, “42,” “John Doe,” and “temperature = 25°C” are all pieces of data.
- Information, on the other hand, is processed, organized, and interpreted data. It’s the “what does it mean?” Data becomes information when it’s given context, meaning, and relevance. For instance, “John Doe is 42 years old, and the temperature is 25°C” is information derived from the raw data.
- In essence, data is the input, and information is the output after processing.
- Representation of Data in Programming:
- Programming languages provide various ways to represent data, depending on its nature.
- This representation involves assigning data to variables and storing it in memory.
- How data is represented directly impacts how efficiently it can be processed.
- For example, a numerical value might be represented as an integer or a floating-point number, depending on whether it has decimal places. Textual data is represented as strings.
- The way data is stored, and the operations that can be performed on it, are defined by the data type.
- Types of Data Structures: Organizing the Elements
- Primitive Data Structures (Fundamental Building Blocks):
- These are the basic data types provided by programming languages.
- They represent single values and are the foundation for more complex structures.
- Examples include:
- Integers (int): Whole numbers (e.g., -10, 0, 5).
- Floats (float): Numbers with decimal points (e.g., 3.14, -0.5).
- Characters (char): Single letters, digits, or symbols (e.g., ‘a’, ‘7’, ‘$’).
- Booleans (bool): Logical values representing true or false.
- Non-Primitive Data Structures (Organized Collections):
- These are derived from primitive data types and allow us to store and organize collections of data.
- They provide ways to structure data for specific purposes, enhancing efficiency and problem-solving.
- Examples include:
- Arrays: Ordered collections of elements of the same data type, stored in contiguous memory locations.
- Lists (Linked Lists): Dynamic collections of elements (nodes) connected by pointers, allowing for flexible insertion and deletion.
- Trees: Hierarchical structures with nodes connected by edges, used for representing relationships and organizing data in a tree-like manner.
- Graphs: Network-like structures with nodes (vertices) and connections (edges), used for modeling relationships between entities.
- Stacks: LIFO(Last in First Out) data structure, used in many implementations for undo buttons, and function calls.
- Queues: FIFO(First in First Out) data structure, used in task scheduling, and buffering.
- Hash Tables: Data structures that use hash functions to map keys to values, enabling fast data retrieval.
By understanding these fundamental concepts, programmers can make informed decisions about how to represent and organize data effectively, leading to more efficient and robust software solutions.
Classification of Data Structures
Data structures, the backbone of efficient programming, can be classified based on how they organize and store data. Here’s a breakdown of the primary classifications:
- Linear Data Structures: Sequential Organization
Linear data structures arrange data elements in a sequential manner, where each element is connected to its preceding and succeeding elements. This creates a linear, one-dimensional arrangement.
- Arrays:
- Arrays are fixed-size, contiguous blocks of memory that store elements of the same data type.
- Elements are accessed using indices, providing fast access to any element.
- They are simple and efficient for storing ordered collections.
- Example: a list of student ID numbers.
- Linked Lists:
- Linked lists are dynamic data structures where elements (nodes) are connected by pointers.
- Each node contains data and a pointer to the next node.
- They offer flexibility for insertion and deletion of elements.
- Example: a playlist of songs.
- Stacks:
- Stacks follow the Last-In, First-Out (LIFO) principle.
- Elements are added and removed from the top of the stack.
- They are used in function calls, expression evaluation, and undo/redo operations.
- Example: The undo feature in a text editor.
- Queues:
- Queues follow the First-In, First-Out (FIFO) principle.
- Elements are added to the rear and removed from the front.
- They are used in task scheduling, print queues, and breadth-first search algorithms.
- Example: a line at a checkout counter.
- Non-Linear Data Structures: Hierarchical and Networked Organization
Non-linear data structures arrange data elements in a hierarchical or network-like manner, where elements can have multiple connections.
- Trees:
- Trees represent hierarchical relationships between elements (nodes).
- They consist of a root node and child nodes.
- Examples include binary trees, search trees, and decision trees.
- Example: a file system directory structure.
- Graphs:
- Graphs consist of nodes (vertices) and connections (edges).
- They can represent complex relationships between entities.
- Examples include social networks, road networks, and web pages.
- Example: connections between friends on a social media platform.
- Static vs. Dynamic Data Structures: Memory Management
Data structures can also be classified based on their memory allocation.
- Static Data Structures:
- Static data structures have a fixed size allocated at compile time.
- The size cannot be changed during program execution.
- Arrays are a common example of static data structures.
- Advantage: faster access to elements.
- Disadvantage: size is not flexible.
- Dynamic Data Structures:
- Dynamic data structures can change their size during program execution.
- Memory is allocated and deallocated dynamically.
- Linked lists, stacks, and queues are examples of dynamic data structures.
- Advantage: flexible size.
- Disadvantage: sometimes have slower access to elements.
Understanding these classifications is crucial for choosing the appropriate data structure for a given problem, leading to efficient and effective software development.
Role of Data Structures in Problem-Solving
Data structures are not just theoretical concepts; they are practical tools that empower programmers to solve complex problems efficiently. Here’s a look at their crucial role:
- Enhancing Performance and Optimization:
- Algorithm Efficiency: The choice of data structure directly impacts the efficiency of algorithms. For example, searching for an element in a sorted array using a binary search (enabled by the array’s ordered nature) is significantly faster than searching in an unsorted linked list.
- Time Complexity Reduction: By selecting the right data structure, developers can minimize the time complexity of operations (e.g., searching, sorting, insertion, deletion). This leads to faster execution times, especially for large datasets.
- Optimized Data Retrieval: Data structures like hash tables and trees enable rapid data retrieval, crucial for applications requiring quick access to information.
- Scalability: Efficient data structures allow applications to handle increasing data volumes and user loads without significant performance degradation.
- Memory Management Considerations:
- Memory Efficiency: Data structures help manage memory effectively by minimizing unnecessary memory usage. For example, linked lists allocate memory dynamically, only using the space needed.
- Dynamic vs. Static Allocation: Understanding the trade-offs between static (arrays) and dynamic (linked lists) memory allocation is crucial for optimizing memory usage.
- Garbage Collection: In languages with automatic garbage collection, efficient data structures can reduce the workload on the garbage collector, improving overall performance.
- Reducing Memory Leaks: Proper use of data structures helps prevent memory leaks, which occur when memory is allocated but not released when no longer needed.
- Use Cases in Real-World Applications:
- Databases: Databases rely heavily on data structures like B-trees and hash tables for efficient data storage and retrieval.
- Web Development: Caching mechanisms in web applications use hash tables to store frequently accessed data for faster loading times.
- Operating Systems: Operating systems use queues for task scheduling and memory management.
- Social Networks: Social networks use graphs to represent relationships between users and analyze network connections.
- Search Engines: Search engines utilize inverted indexes (based on hash tables or trees) to quickly find relevant web pages.
- Game Development: Game developers use data structures like quadtrees and octrees for spatial partitioning and collision detection.
- Artificial Intelligence: AI algorithms frequently use trees and graphs to represent knowledge and relationships.
- Networking: Network protocols rely on data structures to manage packets and routing information.
In essence, data structures are indispensable tools for building efficient, scalable, and robust software solutions. They empower programmers to tackle complex challenges and create applications that meet the demands of modern computing.
Conclusion
Data structures are fundamental to efficient programming and problem-solving. They provide organized ways to store, manage, and manipulate data, ensuring that software applications run smoothly and efficiently. Throughout this discussion, we explored the classification of data structures, their role in optimization, memory management, and real-world applications. Understanding these concepts helps programmers make informed decisions when designing algorithms and software systems.
Choosing the right data structure is crucial for performance and resource management. Whether it’s using arrays for fixed-size storage, linked lists for dynamic memory allocation, or graphs for complex relationships, selecting an appropriate structure directly impacts the speed and efficiency of an application. Poor choices can lead to excessive memory consumption, slow execution times, and inefficient data handling. Therefore, a solid understanding of data structures is essential for writing high-quality code.
As technology evolves, new programming challenges arise, requiring continuous learning and adaptation. Mastering data structures opens the door to advanced topics such as algorithm optimization, big data processing, and artificial intelligence. Future programmers should focus on hands-on implementation, experimenting with different data structures to understand their real-world applications. By doing so, they can build scalable, efficient, and high-performance software solutions.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.