Interest Calculator in C#
Introduction
Table of Contents
Interest calculation is a fundamental concept in finance, widely used in loans, savings, and investments. It helps individuals and businesses understand how much they will earn or owe over time based on the interest rate, principal amount, and time period.
Understanding how interest works is essential for effective financial planning. Whether you’re managing loans, calculating savings returns, or planning investments, knowing how to calculate both simple interest and compound interest can save you time and help you make informed decisions.
In this tutorial, we will guide you through building a C# program that calculates simple interest and compound interest based on user input. You’ll learn how to implement the necessary formulas, handle user input, and display the results. This tutorial is perfect for beginners who want to explore the practical application of interest calculations in programming.
Objectives
This tutorial on interest calculation in C# aims to equip you with the knowledge and practical skills needed to compute both simple and compound interest using programming. By the end of this lesson, you will not only understand how interest works in financial contexts but also be able to write code that performs these calculations. The tutorial is designed to enhance your learning through hands-on practice, ensuring that you can apply the concepts in real-world scenarios.
- Understand the basic concepts of simple and compound interest.
- Grasp the difference between the two types of interest calculations and their applications in loans, savings, and investments.
- Learn how to implement the formulas for simple and compound interest in C#.
- Discover how to write a C# program that takes user input (e.g., principal, rate, time) and performs interest calculations.
- Practice writing and modifying C# code to handle different interest calculation scenarios.
- Work through examples and exercises to compute interest for varying time periods, rates, and compounding frequencies.
- Apply your knowledge to create a fully functional interest calculator in C#.
- Build a real-world application that can be used to calculate simple and compound interest for various financial needs.
These objectives guide you through understanding the core concepts, learning the necessary programming skills, practicing through real-world examples, and applying them in practical scenarios.
Source code example
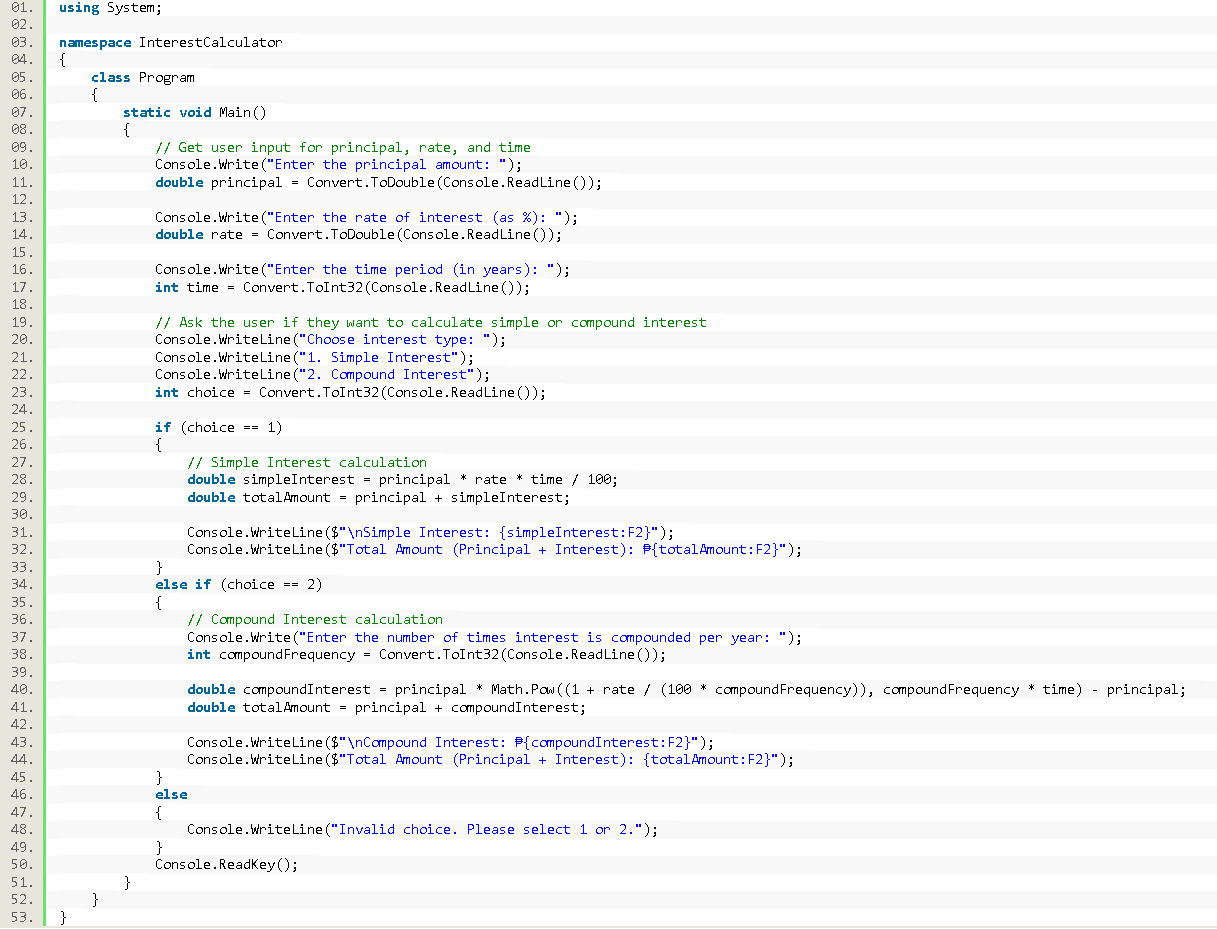
Here’s a beginner-friendly C# source code to calculate both simple interest and compound interest. The program prompts the user for the principal amount, interest rate, and time period, and allows them to choose whether they want to calculate simple or compound interest.
using System; namespace InterestCalculator { class Program { static void Main() { // Get user input for principal, rate, and time Console.Write("Enter the principal amount: "); double principal = Convert.ToDouble(Console.ReadLine()); Console.Write("Enter the rate of interest (as %): "); double rate = Convert.ToDouble(Console.ReadLine()); Console.Write("Enter the time period (in years): "); int time = Convert.ToInt32(Console.ReadLine()); // Ask the user if they want to calculate simple or compound interest Console.WriteLine("Choose interest type: "); Console.WriteLine("1. Simple Interest"); Console.WriteLine("2. Compound Interest"); int choice = Convert.ToInt32(Console.ReadLine()); if (choice == 1) { // Simple Interest calculation double simpleInterest = principal * rate * time / 100; double totalAmount = principal + simpleInterest; Console.WriteLine($"\nSimple Interest: {simpleInterest:F2}"); Console.WriteLine($"Total Amount (Principal + Interest): {totalAmount:F2}"); } else if (choice == 2) { // Compound Interest calculation Console.Write("Enter the number of times interest is compounded per year: "); int compoundFrequency = Convert.ToInt32(Console.ReadLine()); double compoundInterest = principal * Math.Pow((1 + rate / (100 * compoundFrequency)), compoundFrequency * time) - principal; double totalAmount = principal + compoundInterest; Console.WriteLine($"\nCompound Interest: {compoundInterest:F2}"); Console.WriteLine($"Total Amount (Principal + Interest): {totalAmount:F2}"); } else { Console.WriteLine("Invalid choice. Please select 1 or 2."); } Console.ReadKey(); } } }
Explanation:
- User Input:
- The program asks the user to enter the principal amount, interest rate (as a percentage), and time period (in years).
- It then asks the user to choose between calculating simple interest or compound interest.
- Simple Interest Calculation:
- If the user chooses Simple Interest, the program uses the formula:
Simple Interest (SI)=Principal×Rate×Time/100
-
- The total amount (principal + interest) is then calculated and displayed.
- Compound Interest Calculation:
- If the user chooses Compound Interest, the program asks for the number of times interest is compounded per year (e.g., annually, semi-annually, etc.).
- The formula for compound interest is:
Compound Interest (CI)=Principal×(1+100×FrequencyRate)Frequency×Time−Principal
-
- The total amount (principal + compound interest) is calculated and displayed.
- Error Handling:
If the user enters an invalid choice (neither 1 nor 2), the program informs them of the invalid selection.
Example Output
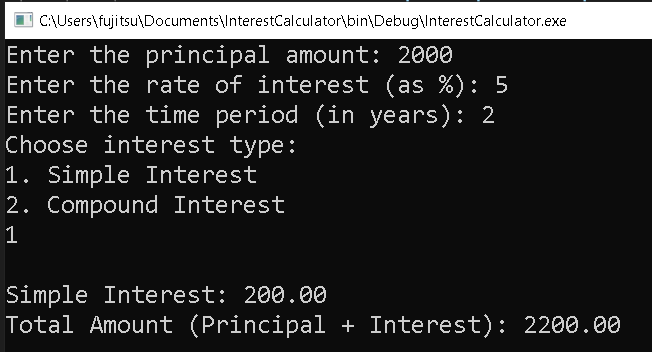
Summary
In this lesson, we explored how to calculate simple and compound interest using C#. We covered key financial concepts like principal, interest rate, and time period, and demonstrated how to implement the respective formulas in a C# program. The tutorial walked through the process of collecting user input, performing the interest calculations based on the user’s choice, and displaying the results. This hands-on approach helps beginners understand basic programming concepts such as user input handling, conditional statements, and mathematical operations, while providing a practical application for financial calculations.
Exercises and Assessment
To further develop your understanding of interest calculations in C# and improve the source code, it’s essential to practice applying the concepts through real-world scenarios. The following exercises, assessments, and lab exam will challenge you to enhance the interest calculator by adding more advanced features such as additional input options, error handling, and advanced financial calculations. These tasks will help solidify your programming skills and expand the functionality of your C# interest calculator.
Exercise 1: Compare Simple and Compound Interest
- Allow the user to calculate both simple and compound interest in the same session and display the difference between the two. This will help users understand the impact of compounding on interest calculations.
Exercise 2: Calculate Monthly Payments
- Modify the program to calculate monthly interest payments. Prompt the user to enter the number of months, and then display the monthly payment along with the total interest.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.