Feedback Platform with Sentiment Analysis Database Design
In this tutorial, we will guide you through building a feedback platform with sentiment analysis. By the end of this tutorial, you will have a clear understanding of how to design a database for collecting feedback and how to implement sentiment analysis to gain deeper insights from the feedback data.
Introduction
Table of Contents
The main goal of this tutorial is to help you create a robust feedback platform that can gather, store, and analyze feedback from users. We will walk you through the process of designing a database schema that supports feedback collection and integrates sentiment analysis. You will also learn how to implement features for feedback submission, perform sentiment analysis, and generate insightful reports based on user feedback.
Feedback platforms play a critical role in many industries, including education, business, and customer service. In educational settings, feedback from students can help improve teaching methods and course content. In the business world, customer feedback provides valuable insights into product performance and customer satisfaction. By using feedback platforms, organizations can gather input from their audience, understand their needs, and make data-driven decisions to enhance their services or products.
Sentiment analysis is a powerful tool that helps to automatically identify and extract the sentiment expressed in user feedback. By analyzing the tone of comments—whether positive, negative, or neutral—organizations can quickly understand the overall mood and opinions of their users. This analysis enables businesses and institutions to respond effectively to customer concerns, celebrate successes, and continuously improve based on real-time feedback. Integrating sentiment analysis into your feedback platform adds a valuable layer of understanding that goes beyond basic data collection, providing actionable insights for strategic decision-making.
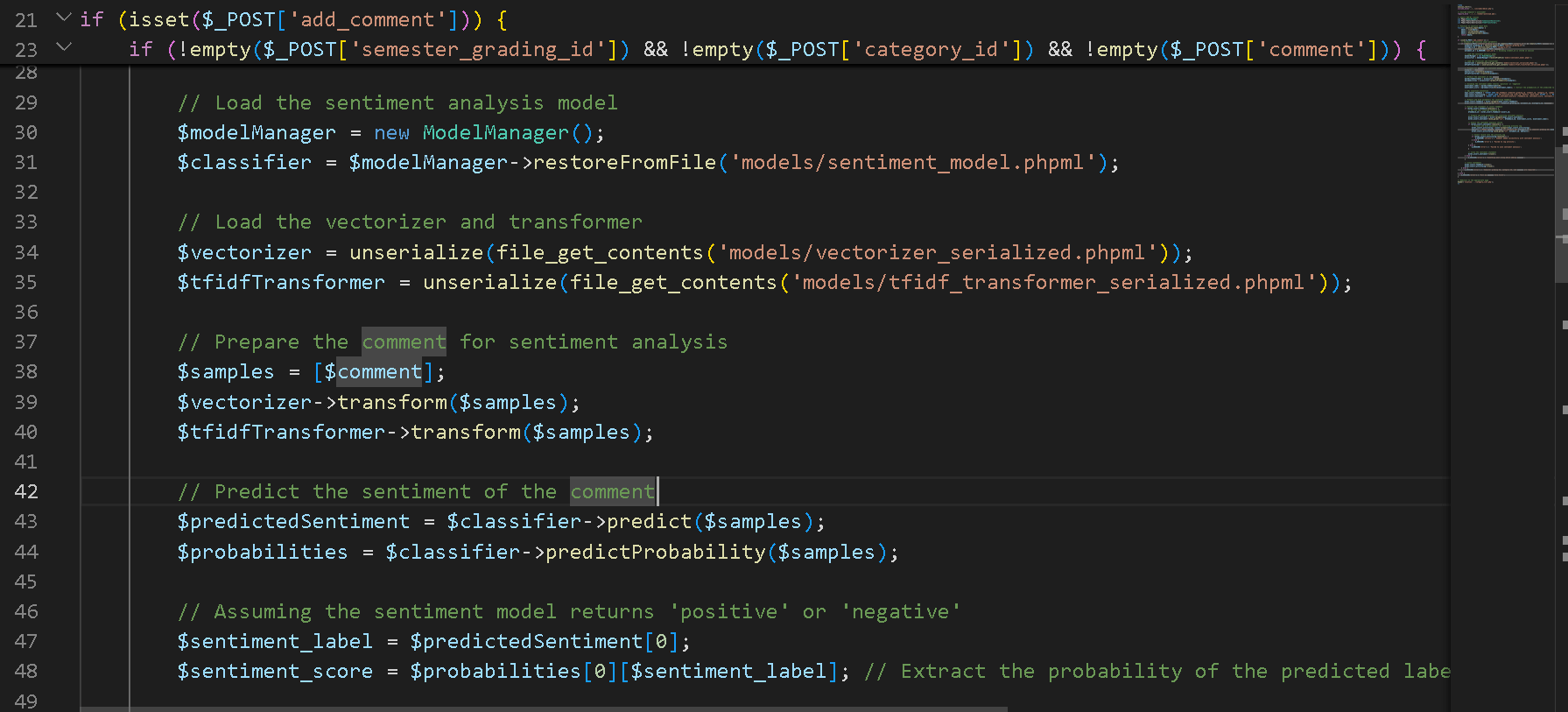
Understanding the Requirements
Defining the Scope
Before diving into the design and development of the feedback platform, it’s crucial to clearly define its scope. This involves identifying the specific goals and objectives of the platform, as well as the target audience and the types of feedback it will collect.
User Roles
Consider the various roles that will interact with the feedback platform. For instance, you might have:
- Administrators: Responsible for managing the platform, creating feedback forms, and analyzing data.
- Students: Users who submit feedback and view reports.
- Faculty: Users who may create feedback forms or analyze student feedback.
Key Features
The following features are essential for a comprehensive feedback platform:
- Feedback Submission: A user-friendly interface for submitting feedback, including options for rating or scoring, and providing detailed comments.
- Sentiment Analysis: Integration of a sentiment analysis tool or library to automatically classify feedback as positive, negative, or neutral.
- Report Generation: The ability to generate various types of reports, such as summary reports, trend analysis, and detailed breakdowns of feedback categories.
- Data Visualization: Tools for visualizing feedback data, such as charts, graphs, and dashboards, to facilitate understanding and communication.
- User Management: If applicable, a system for managing user accounts, permissions, and access controls.
By carefully considering these factors, you can ensure that the feedback platform aligns with the specific needs and goals of your organization.
Database Design Concepts
Designing a robust and efficient database is a critical step in developing a feedback platform. A well-structured database ensures that data is stored efficiently, relationships are maintained, and the platform can scale effectively. Here, we will cover essential database design concepts that are relevant to building a feedback platform with sentiment analysis.
Relational Databases and Their Components
A relational database organizes data into tables, which consist of rows and columns. It is called “relational” because it can define relationships between different tables, allowing for efficient data management and retrieval. The primary components of a relational database include:
- Tables: A table is a collection of related data entries. Each table represents a specific entity, such as students, feedback, or categories. In a feedback platform, different tables might store user information, feedback data, and sentiment analysis results.
- Columns: Columns, also known as fields or attributes, define the structure of a table by specifying the type of data each entry in the table will hold. For example, a students table might have columns like student_id, student_name, email, and course.
- Rows: Rows, also known as records, contain the actual data entries for each column. Each row represents a unique instance of the entity defined by the table.
- Relationships: Relationships define how tables are connected. Common relationships include one-to-one, one-to-many, and many-to-many. In a feedback platform, there might be a one-to-many relationship between students and feedback, where each student can submit multiple feedback entries.
Data Types and Their Usage in Feedback Platforms
Data types specify the kind of data that can be stored in each column of a table. Choosing the appropriate data type is essential for optimizing storage and ensuring data integrity. Common data types used in feedback platforms include:
- Integer: Used for numerical data without decimals, such as IDs (student_id, feedback_id) or counters (e.g., number of feedback entries).
- Varchar: Used for variable-length strings, such as names (student_name), email addresses, and comments. It allows flexibility in the length of text data.
- Text: Used for longer text fields, such as detailed comments or feedback. It is suitable for storing large amounts of textual data.
- DateTime: Used to store date and time information, such as the date a feedback entry was submitted (date_time_recorded).
- Float/Double: Used for numerical data with decimal points, such as sentiment scores that might range from -1.0 to 1.0.
- Boolean: Used for true/false values, such as flags to indicate active/inactive status (is_active).
Normalization and Its Importance
Normalization is the process of organizing data in a database to reduce redundancy and improve data integrity. The goal is to ensure that each piece of data is stored in one place, avoiding duplication and inconsistencies. The main benefits of normalization include:
- Eliminating Redundancy: By organizing data into separate tables based on entities and relationships, normalization prevents the same data from being stored in multiple places. This reduces storage requirements and minimizes the risk of inconsistent data.
- Improving Data Integrity: Normalization enforces rules that maintain the accuracy and consistency of data. For example, changes to a student’s information are made in one place, ensuring that all related feedback entries automatically reflect the update.
- Facilitating Maintenance: A normalized database structure is easier to maintain and update, as changes to the schema or data can be made with minimal impact on the overall system.
Common normalization forms include:
- First Normal Form (1NF): Ensures that each column contains atomic values and each entry is unique.
- Second Normal Form (2NF): Ensures that all non-key attributes are fully functional dependent on the primary key.
- Third Normal Form (3NF): Ensures that no transitive dependencies exist, meaning non-key attributes do not depend on other non-key attributes.
Indexing and Its Benefits
Indexing is a technique used to speed up the retrieval of data from a database. An index creates a data structure that allows the database engine to find records more quickly, similar to an index in a book. Key benefits of indexing include:
- Faster Query Performance: Indexes significantly reduce the amount of data the database engine needs to scan to find relevant records. For example, indexing columns frequently used in search queries, such as student_id or category_id, can speed up the retrieval process.
- Efficient Data Sorting: Indexes can help sort data more efficiently, making operations like ordering and grouping faster.
- Improved Join Operations: In relational databases, join operations are common when querying related tables. Indexing the columns used in joins can enhance the speed and efficiency of these operations.
While indexing improves query performance, it is essential to use it judiciously. Over-indexing can lead to increased storage requirements and slower write operations (inserts, updates, deletes). Therefore, it is important to index only those columns that are frequently used in queries and where performance improvements are necessary.
By understanding these database design concepts—relational databases, data types, normalization, and indexing—you can create a well-structured, efficient, and scalable database for your feedback platform, ensuring it can handle large volumes of data while maintaining high performance.
Database Design
In designing the feedback platform with sentiment analysis, each database table plays a specific role in managing and organizing the data efficiently. Here is a detailed discussion of each table and its significance within the system:
- tbl_course_grade
- Purpose: This table is designed to manage different course grades applicable to both high school and college levels. By having a separate table for course grades, the system can flexibly accommodate different academic structures.
- Columns:
- course_grade_id: A unique identifier for each course grade, ensuring that each entry can be distinctly referenced.
- course_grade_code: A short code representing the course grade (e.g., “HS1” for High School Grade 1).
- course_grade_name: A descriptive name for the course grade (e.g., “High School Grade 1”, “College Freshman”).
- Usage: The tbl_course_grade table helps categorize students and feedback based on their educational level, allowing for more tailored analysis and reporting.
- tbl_semester_grading
- Purpose: This table tracks different semesters or grading periods, which can vary between institutions. It is crucial for organizing feedback data chronologically.
- Columns:
- semester_grading_id: A unique identifier for each semester or grading period.
- semester_grading_code: A code that uniquely represents a specific semester (e.g., “2024S1” for Spring 2024).
- semester_grading_description: A more detailed description of the semester (e.g., “Spring Semester 2024”).
- is_active: A boolean field indicating whether the semester is currently active. This helps in filtering relevant data and reports.
- Usage: By using tbl_semester_grading, the platform can differentiate feedback entries based on the semester, which is essential for tracking changes over time and for academic performance reviews.
- tbl_student
- Purpose: This table stores detailed information about each student in the system, including their personal details and academic affiliation.
- Columns:
- student_id: A unique identifier for each student.
- student_complete_name: The full name of the student.
- student_email_address: The student’s email address, ensuring unique identification and communication.
- student_contact_number: The contact number for direct communication if needed.
- student_profile_image: A link or path to the student’s profile image, enhancing the user interface.
- s_username: A username for the student’s login.
- s_password: A hashed password for security purposes.
- course_grade_id: A foreign key linking to tbl_course_grade, indicating the student’s current grade level.
- Usage: The tbl_student table is central to linking personal and academic data with feedback entries, ensuring that each piece of feedback can be traced back to the specific student who provided it.
- tbl_category
- Purpose: To classify feedback into various categories, making it easier to analyze and understand specific areas of concern or excellence.
- Columns:
- category_id: A unique identifier for each feedback category.
- category_name: The name of the category (e.g., “Teaching Style”, “Library Resources”).
- description: A detailed description of what the category covers.
- type: A broader classification (e.g., “Academics”, “Facilities”, “Services”).
- Usage: tbl_category allows the system to organize feedback into meaningful groups, aiding in targeted analysis and actionable insights.
- tbl_feedback
- Purpose: This table captures all the feedback entries from students, providing a structured way to store, manage, and analyze feedback.
- Columns:
- feedback_id: A unique identifier for each feedback entry.
- semester_grading_id: A foreign key linking to tbl_semester_grading, associating feedback with a specific semester.
- student_id: A foreign key linking to tbl_student, identifying which student gave the feedback.
- category_id: A foreign key linking to tbl_category, categorizing the feedback.
- comments: The actual feedback text provided by the student.
- date_time_recorded: The date and time when the feedback was recorded.
- Usage: The tbl_feedback table is critical for storing the actual feedback data. It links directly to students, semesters, and categories, enabling a multi-dimensional view of feedback.
- tbl_sentiment_analysis
- Purpose: This table stores the results of sentiment analysis performed on the feedback entries. It helps in understanding the overall mood and sentiment of the feedback provided by students.
- Columns:
- sentiment_analysis_id: A unique identifier for each sentiment analysis entry.
- feedback_id: A foreign key linking to tbl_feedback, showing which feedback entry the sentiment analysis refers to.
- sentiment_score: A numerical score indicating the sentiment’s intensity (e.g., ranging from -1.0 to 1.0, where negative values indicate negative sentiment, and positive values indicate positive sentiment).
- sentiment_label: A textual label indicating the sentiment (e.g., “Positive”, “Negative”, “Neutral”).
- date_time_recorded: The date and time when the sentiment analysis was recorded.
- Usage: tbl_sentiment_analysis provides insights into the emotional tone of feedback, which is valuable for understanding student satisfaction and identifying areas that need improvement.
These tables collectively form the backbone of the feedback platform, enabling the collection, categorization, analysis, and reporting of feedback data. Each table has been carefully designed to ensure data integrity, efficient storage, and meaningful relationships. By structuring the database in this manner, the platform can handle large volumes of data while providing insightful analysis and reports, thereby enhancing decision-making processes based on student feedback.
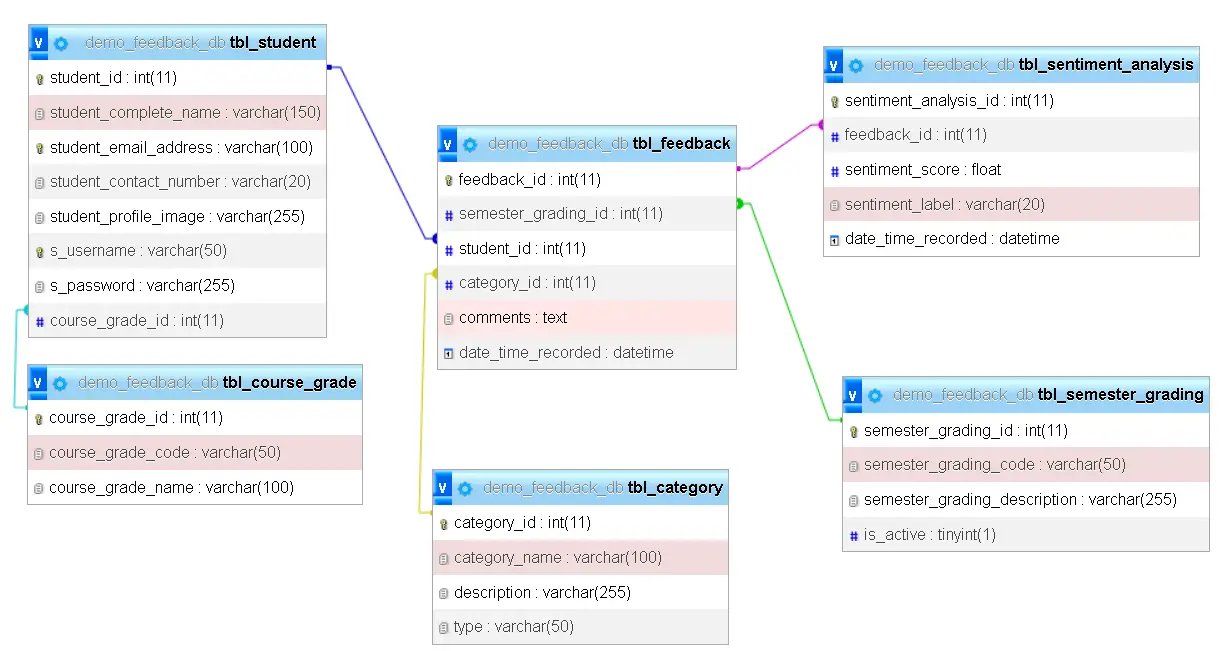
SQL Statements
-- Table: tbl_course_grade CREATE TABLE tbl_course_grade ( course_grade_id INT AUTO_INCREMENT PRIMARY KEY, course_grade_code VARCHAR(50) NOT NULL, course_grade_name VARCHAR(100) NOT NULL ); -- Table: tbl_semester_grading CREATE TABLE tbl_semester_grading ( semester_grading_id INT AUTO_INCREMENT PRIMARY KEY, semester_grading_code VARCHAR(50) NOT NULL, semester_grading_description VARCHAR(255) NOT NULL, is_active BOOLEAN NOT NULL DEFAULT 0 ); -- Table: tbl_student CREATE TABLE tbl_student ( student_id INT AUTO_INCREMENT PRIMARY KEY, student_complete_name VARCHAR(150) NOT NULL, student_email_address VARCHAR(100) NOT NULL UNIQUE, student_contact_number VARCHAR(20) NOT NULL, student_profile_image VARCHAR(255), s_username VARCHAR(50) NOT NULL UNIQUE, s_password VARCHAR(255) NOT NULL, course_grade_id INT, FOREIGN KEY (course_grade_id) REFERENCES tbl_course_grade(course_grade_id) ); -- Table: tbl_category CREATE TABLE tbl_category ( category_id INT AUTO_INCREMENT PRIMARY KEY, category_name VARCHAR(100) NOT NULL, description VARCHAR(255), type VARCHAR(50) NOT NULL ); -- Table: tbl_feedback CREATE TABLE tbl_feedback ( feedback_id INT AUTO_INCREMENT PRIMARY KEY, semester_grading_id INT, student_id INT, category_id INT, comments TEXT, date_time_recorded DATETIME DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (semester_grading_id) REFERENCES tbl_semester_grading(semester_grading_id), FOREIGN KEY (student_id) REFERENCES tbl_student(student_id), FOREIGN KEY (category_id) REFERENCES tbl_category(category_id) ); -- Table: tbl_sentiment_analysis CREATE TABLE tbl_sentiment_analysis ( sentiment_analysis_id INT AUTO_INCREMENT PRIMARY KEY, feedback_id INT, sentiment_score FLOAT, sentiment_label VARCHAR(20), date_time_recorded DATETIME DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (feedback_id) REFERENCES tbl_feedback(feedback_id) );
Explanation:
- tbl_course_grade: Stores information about course grades for both high school and college. Each entry has an ID, a code, and a name.
- tbl_semester_grading: Contains semester information, including a code, description, and an active status to indicate whether it’s the current semester.
- tbl_student: Keeps track of student details. It includes a foreign key (course_grade_id) that links to tbl_course_grade, establishing a relationship between students and their course grades.
- tbl_category: Holds different feedback categories like teaching style, library resources, etc., and categorizes them by type such as academics, facilities, or services.
- tbl_feedback: Collects feedback from students. It uses foreign keys to relate feedback to specific semesters, students, and categories, enabling detailed and structured data analysis.
- tbl_sentiment_analysis: Stores sentiment analysis results for each feedback entry. It includes the sentiment score and label, and links back to the corresponding feedback via a foreign key.
Key Points:
- Primary Keys: Each table has a primary key to uniquely identify each record.
- Foreign Keys: Relationships between tables are established using foreign keys, ensuring referential integrity.
- Data Types: Appropriate data types are chosen for each column, such as VARCHAR for strings, INT for integers, TEXT for longer text fields, DATETIME for date and time, and BOOLEAN for true/false values.
- Default Values: The date_time_recorded fields in tbl_feedback and tbl_sentiment_analysis use the CURRENT_TIMESTAMP to automatically capture the date and time when a new record is inserted.
These SQL statements will help you set up a structured and scalable database for your feedback platform with sentiment analysis.
Conclusion and Next Steps
Throughout this tutorial, we have explored the essential components of database design for feedback platforms. We have discussed the importance of defining the scope, understanding user requirements, and selecting appropriate data types. Additionally, we have delved into the concepts of normalization and indexing to ensure data integrity and optimize performance.
Benefits of Database Design Planning
A well-designed database is crucial for the success of a feedback platform. It provides a solid foundation for data storage, retrieval, and analysis. By carefully planning your database design, you can:
- Improve data integrity: Ensure that data is consistent and accurate.
- Enhance query performance: Optimize database operations for faster data retrieval.
- Facilitate data analysis: Support the generation of meaningful reports and insights.
- Scale effectively: Accommodate future growth and increasing data volumes.
What’s Next?
Once the database design is complete, the next steps typically involve:
- Database implementation: Creating the physical database structure and populating it with data.
- Data integration: Connecting the database to the feedback platform’s frontend and backend components.
- Testing and optimization: Thoroughly testing the database to ensure it functions as expected and making necessary adjustments to improve performance.
- Ongoing maintenance: Regularly monitoring and maintaining the database to address issues, update schema, and ensure data security.
By following these steps, you can successfully implement a robust and efficient database for your feedback platform, enabling you to collect, analyze, and leverage valuable insights from user feedback.
Readers are also interested in:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.