Electricity Bill Calculator in C#
Introduction
Table of Contents
Electricity billing is the process of calculating how much energy you consume over a billing period, typically measured in kilowatt-hours (kWh). Knowing how to calculate your electricity bill is crucial for budgeting and understanding your energy usage, whether it’s for a household or business.
In real-world applications, calculating electricity consumption allows users to monitor their energy efficiency and avoid unexpected expenses. Utility companies often use tiered billing systems where rates increase as usage goes up, making it important to understand how your bill is calculated.
In this tutorial, we’ll guide you through creating a simple C# program that calculates an electricity bill based on user input and usage. This program will consider various rate slabs, making it a useful tool for learning about conditional statements and mathematical operations in programming.
Objectives
In this tutorial, we aim to provide you with a comprehensive understanding of how electricity billing works and how to implement it using C#. Through a step-by-step approach, you will learn the fundamentals of programming concepts such as user input, conditional statements, and calculations. By the end of this tutorial, you’ll have the opportunity to practice these skills and apply them to create a functional electricity bill calculator. The objectives below will guide you through the key focus areas of this tutorial.
Objectives:
- Understand the basic concept of electricity billing and rate slabs.
- Grasp the importance of calculating electricity consumption and how billing systems typically work.
- Learn how to implement user input and conditional logic in C#.
- Discover how to collect electricity usage from the user and apply different rates based on usage using if-else statements.
- Practice writing a C# program that computes an electricity bill.
- Follow along with examples to practice using arithmetic operations, conditional statements, and input/output methods.
- Apply your knowledge to build a real-world C# application.
- Create a fully functional electricity bill calculator and adapt it for various scenarios, such as different rate structures or additional charges.
These objectives focus on helping you develop a deeper understanding of key programming concepts, allowing you to practice and apply them in real-world scenarios.
Source code example
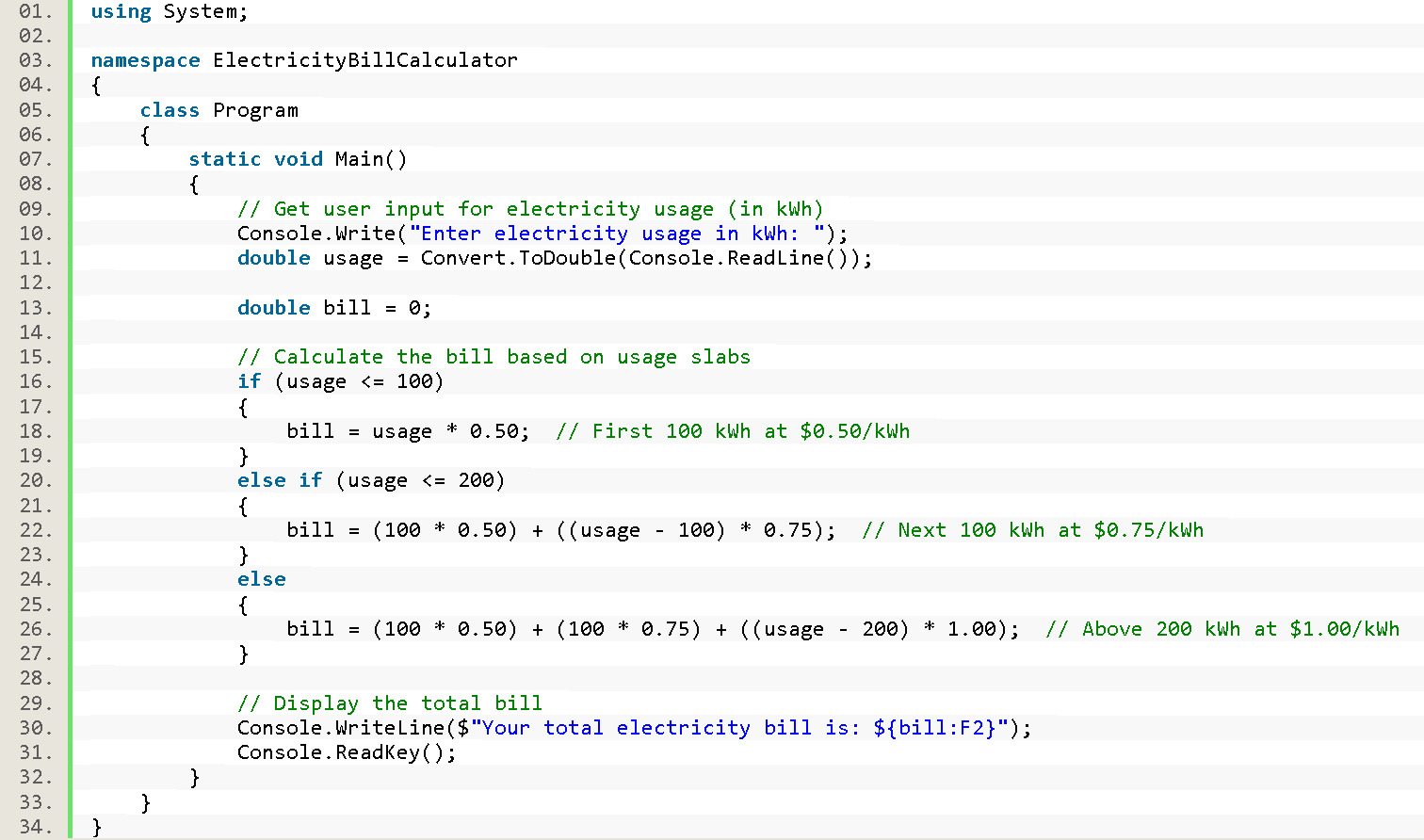
Here’s a simple, beginner-friendly C# source code for calculating an electricity bill based on usage. This program asks the user for their electricity consumption (in kilowatt-hours or kWh) and applies different rates based on usage. The program uses conditional statements (if-else) to handle rate slabs.
using System; namespace ElectricityBillCalculator { class Program { static void Main() { // Get user input for electricity usage (in kWh) Console.Write("Enter electricity usage in kWh: "); double usage = Convert.ToDouble(Console.ReadLine()); double bill = 0; // Calculate the bill based on usage slabs if (usage <= 100) { bill = usage * 0.50; // First 100 kWh at $0.50/kWh } else if (usage <= 200) { bill = (100 * 0.50) + ((usage - 100) * 0.75); // Next 100 kWh at $0.75/kWh } else { bill = (100 * 0.50) + (100 * 0.75) + ((usage - 200) * 1.00); // Above 200 kWh at $1.00/kWh } // Display the total bill Console.WriteLine($"Your total electricity bill is: ${bill:F2}"); Console.ReadKey(); } } }
Explanation:
- User Input:
The program starts by asking the user to enter their electricity usage in kilowatt-hours (kWh). The Console.ReadLine() method is used to get the input, and Convert.ToDouble() is used to convert it to a double for calculation purposes. - Conditional Logic:
The if-else statements apply different rates based on the user’s usage:- If usage is less than or equal to 100 kWh, a rate of $0.50 per kWh is applied.
- If usage is between 101 and 200 kWh, the first 100 kWh are billed at $0.50, and the remaining usage is billed at $0.75 per kWh.
- For usage above 200 kWh, the first 100 kWh are billed at $0.50, the next 100 kWh at $0.75, and any usage above 200 kWh at $1.00 per kWh.
- Output:
After calculating the bill, the total amount is displayed using Console.WriteLine(), formatted to two decimal places (${bill:F2}).
Example Output
Enter electricity usage in kWh: 250 Your total electricity bill is: $162.50
Summary
In this lesson, we explored how to calculate an electricity bill based on usage using C# programming. By gathering user input for electricity consumption in kilowatt-hours (kWh) and applying tiered rates through conditional statements (if-else), we created a program that computes the total bill. The program demonstrates key programming concepts such as input handling, arithmetic operations, and conditional logic. Additionally, we incorporated real-world application by displaying the final bill. This hands-on exercise enhances understanding of how programming can be used to solve practical problems like calculating utility bills.
Exercises and Assessment
To solidify your understanding of how conditional statements and arithmetic operations work in C#, it’s important to practice and enhance the electricity bill calculator by applying real-world scenarios. The following exercises, assessment, and lab exam are designed to help you improve the source code. These tasks will challenge you to expand the program’s functionality by incorporating more advanced features such as taxes, additional charges, and error handling, further enhancing your problem-solving skills in programming.
- Calculate and Display Tax
- Add a 12% VAT (Value Added Tax) to the electricity bill.
- Calculate the total bill including VAT, and display both the bill before tax and the total bill after tax.
- Exercise 4: Handling Invalid Input
- Enhance the program to check for invalid input (e.g., negative usage values or non-numeric input).
- Display an error message and prompt the user to enter valid input.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.