C# Variable
Introduction to Variables
Table of Contents
In programming, variables are like containers that hold data. They allow us to store, modify, and access information throughout our code. Think of a variable as a box labeled with a name where you can put a value, such as a number, a word, or even a more complex object.
Definition of Variables
A variable is a named storage location in the computer’s memory. It has a specific type, which defines the kind of data it can hold (like a number, text, or true/false value). Variables make our programs flexible because we can use them to represent changing data, like the score in a game, the temperature in a weather app, or the name of a user.
Importance of Variables in Programming
Variables are essential in programming because they help us:
- Store Data: We use variables to keep information that our program needs to run, such as user input or calculated results.
- Improve Readability: Using meaningful variable names makes our code easier to understand. For example, using userAge is more understandable than just using x.
- Reuse and Update Values: We can update a variable’s value as needed. This means we don’t have to hard-code values, which makes our code more adaptable and less error-prone.
- Perform Calculations and Operations: Variables allow us to do mathematical calculations, store results, and use them in different parts of our program.
How Variables Store Data
When we declare a variable in C#, we tell the computer to set aside some memory to store a value. Here’s how it works:
- Declaration: We start by giving our variable a name and a type. The type tells the computer what kind of data the variable will hold. For example:
int age; // Declares a variable named 'age' that will hold an integer value.
- Assignment: We then assign a value to the variable:
age = 25; // Now, 'age' holds the value 25.
- Usage: Once a variable has a value, we can use it throughout our program:
Console.WriteLine("Your age is " + age); // Outputs: Your age is 25
This process helps us manage data efficiently, making our programs dynamic and interactive. In the example above, age is a variable that stores an integer value representing a person’s age. If the person’s age changes, we can easily update the age variable without changing other parts of the code.
Syntax and Declaration
In C#, declaring a variable means creating it and assigning it a type. This process is straightforward but crucial, as it tells the program what kind of data the variable will hold.
Basic Syntax for Declaring Variables in C#
The basic syntax for declaring a variable in C# is:
dataType variableName = value;
- dataType: Specifies the kind of data the variable will hold, such as int for integers or string for text.
- variableName: A unique name you choose for the variable.
- value (optional): The initial value assigned to the variable.
Example 1: Declaring an integer variable named age and assigning it a value of 25:
int age = 25;
Example 2: Declaring a string variable named greeting with the value “Hello, World!”:
string greeting = "Hello, World!";
Example 3: Declaring a string variable named temperature with the value of 36.1:
double temperature = 36.1;
Naming Conventions and Best Practices
Choosing good names for variables makes your code easier to read and maintain. Here are some best practices:
- Use Descriptive Names: Choose names that clearly describe the data the variable holds. For example, use userAge instead of ua.
- Camel Case for Local Variables: Start the variable name with a lowercase letter and capitalize the first letter of each subsequent word. For example, studentName or totalAmount.
- Avoid Single-Letter Names: Unless used in a very short and simple context, avoid single-letter names like x or y. They don’t provide meaningful information about the data.
- No Spaces or Special Characters: Variable names cannot contain spaces or special characters (except underscore _). For example, use user_name instead of user name.
- Avoid Reserved Keywords: You cannot use C# keywords like int, string, or class as variable names. If necessary, use an underscore or add a prefix to avoid conflicts.
Type Conversion and Casting
Type conversion and casting allow us to change the type of a variable in C#. This is important when we need to perform operations between different types of data or when we need to store data in a format that is different from its original type.
Implicit and Explicit Type Conversion
- Implicit Type Conversion (Automatic Conversion) Implicit conversion happens automatically when the compiler can safely convert one type to another without losing data or causing errors. This usually occurs when converting from a smaller type to a larger type, such as int to double.
Example:
int number = 10; double decimalNumber = number; // Implicit conversion from int to double Console.WriteLine(decimalNumber); // Output: 10.0
In this example, the integer number is automatically converted to a double because all integers can be represented as doubles without losing information.
- Explicit Type Conversion (Casting) Explicit conversion is required when there is a possibility of data loss or the conversion may not always succeed. This is done using a cast operator (type).
Example:
double decimalNumber = 9.8; int wholeNumber = (int)decimalNumber; // Explicit conversion from double to int Console.WriteLine(wholeNumber); // Output: 9 (fractional part is discarded)
In this example, the decimalNumber is explicitly cast to an integer. The fractional part is lost, and only the whole number is stored.
Using the Convert Class and Parse Method
- Convert Class The Convert class provides methods to convert from one type to another, such as from a string to a number. It handles common conversions and provides error handling if the conversion is not possible.
Example:
string strNumber = "123"; int number = Convert.ToInt32(strNumber); // Converts string to integer Console.WriteLine(number); // Output: 123
In this example, Convert.ToInt32 converts the string “123” into an integer.
- Parse Method The Parse method converts a string representation of a number into its numeric equivalent. Unlike the Convert class, it throws an exception if the conversion fails.
Example:
string strNumber = "45.67"; double decimalNumber = double.Parse(strNumber); // Converts string to double Console.WriteLine(decimalNumber); // Output: 45.67
In this example, double.Parse converts the string “45.67” into a double.
Note: If the string is not in a valid numeric format, the Parse method will throw a FormatException. To handle such cases gracefully, you can use TryParse:
string strNumber = "abc"; int result; bool success = int.TryParse(strNumber, out result); Console.WriteLine(success); // Output: False Console.WriteLine(result); // Output: 0
Here, TryParse attempts to convert the string “abc” to an integer, fails, and sets success to false without throwing an exception.
Key Points:
- Implicit Conversion happens automatically when there is no risk of data loss.
- Explicit Conversion (casting) is required when data loss is possible.
- The Convert class and Parse method are used to convert between different data types.
Examples and Exercises
In this section, we’ll explore practical examples to demonstrate how variables are used in C#. You’ll also find some exercises to help you practice declaring, initializing, and manipulating variables.
Example 1: Storing and Displaying User Information
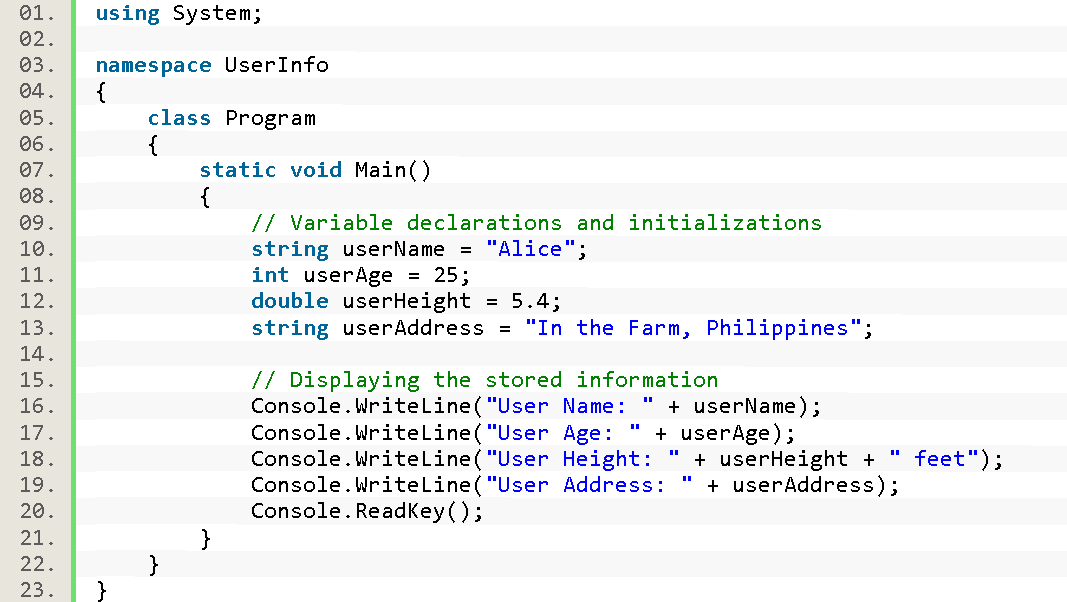
using System; namespace UserInfo { class Program { static void Main() { // Variable declarations and initializations string userName = "Alice"; int userAge = 25; double userHeight = 5.4; string userAddress = "In the Farm, Philippines"; // Displaying the stored information Console.WriteLine("User Name: " + userName); Console.WriteLine("User Age: " + userAge); Console.WriteLine("User Height: " + userHeight + " feet"); Console.WriteLine("User Address: " + userAddress); Console.ReadKey(); } } }
Explanation:
- userName is a string variable that stores the name “Alice”.
- userAge is an int variable that stores the age 25.
- userHeight is a double variable that stores the height 5.6.
- The Console.WriteLine statements display the values of these variables.
Example 2: Calculating the Sum of Two Numbers
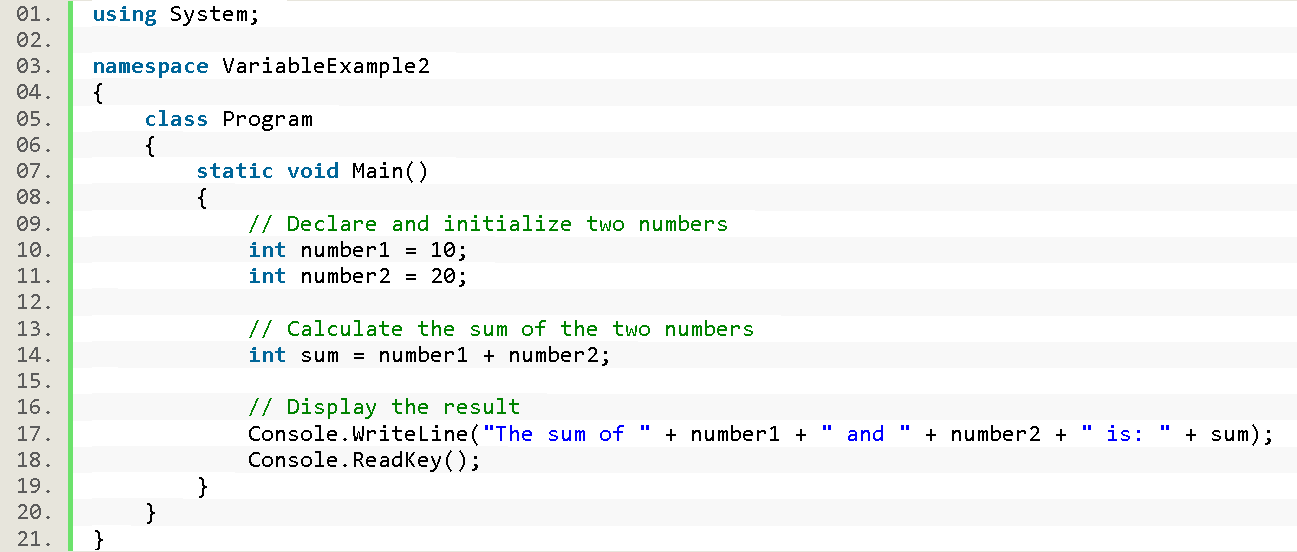
using System; namespace VariableExample2 { class Program { static void Main() { // Declare and initialize two numbers int number1 = 10; int number2 = 20; // Calculate the sum of the two numbers int sum = number1 + number2; // Display the result Console.WriteLine("The sum of " + number1 + " and " + number2 + " is: " + sum); Console.ReadKey(); } } }
Explanation:
- Variable Declaration and Initialization:
- number1 and number2 are integer variables initialized with values 10 and 20 respectively.
- The sum variable is used to store the result of adding number1 and number2.
- Calculation:
- The sum is calculated using the expression number1 + number2 and assigned to the sum variable.
- Output:
- The program displays the sum using Console.WriteLine, combining the text and variable values to create a descriptive message.
Summary and Recap
- Definition: A variable is a named storage location in memory, used to store data that can be modified and accessed throughout a program.
- Importance: Variables help store data, improve code readability, and allow for easy updates and calculations.
- Declaration: Use the syntax dataType variableName = value; to declare and initialize variables. Always use descriptive and meaningful names.
- Best Practices: Use camelCase for naming, avoid single-letter names, and don’t use reserved keywords as variable names.
Exercise 1: Simple Arithmetic Operations
- Create a program that declares two integer variables, a and b, with values 15 and 8.
- Calculate and display the sum, difference, product, and quotient of these two variables.
Expected Output:
Sum: 23 Difference: 7 Product: 120 Quotient: 1
Exercise 2: Average Calculation
- Declare three double variables num1, num2, and num3 with values 5.7, 8.2, and 3.9.
- Calculate and display the average of these three numbers.
Expected Output:
Average: 5.93333333333333
- What is the correct syntax to declare an integer variable in C#?
- A) int age;
- B) integer age;
- C) age int;
- D) declare int age;
- Which of the following data types can store a true/false value?
- A) int
- B) string
- C) bool
- D) char
- What does the following line of code do? double temperature = 36.5;
- A) Declares a variable temperature of type double and assigns it a value of 36.5.
- B) Declares an integer variable named temperature and assigns it a value of 36.5.
- C) Declares a string variable named temperature and assigns it a value of “36.5”.
- D) Declares a character variable named temperature and assigns it a value of ’36’.
- Which of the following best describes the purpose of variables in programming?
- A) They are used to execute loops and conditions.
- B) They are containers for storing data values.
- C) They are used to define classes and methods.
- D) They are used to output data to the console.
- Which of the following is a valid way to change the value of an already declared variable userAge?
- A) userAge = 30;
- B) int userAge = 30;
- C) string userAge = “30”;
- D) bool userAge = true;
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.