C# Operators
Introduction to Operators
Table of Contents
In programming, operators are special symbols or keywords that perform operations on variables and data. They allow you to manipulate data, perform calculations, and control the flow of your program. Understanding operators is essential for writing effective and efficient code.
Definition of Operators in Programming
Operators can be thought of as tools that perform specific tasks within a program. In C#, operators allow you to carry out various functions such as arithmetic calculations, comparisons, and logical operations. For example, when you want to add two numbers together, you use the addition operator (+).
Importance of Operators in C#
Operators play a vital role in C# for several reasons:
- Data Manipulation: Operators enable you to perform calculations and modify data stored in variables. For example, you can add, subtract, multiply, or divide numerical values using arithmetic operators.
- Conditional Logic: Comparison operators allow you to compare values and make decisions based on certain conditions. This is crucial for controlling the flow of your program through conditional statements (like if statements).
- Boolean Operations: Logical operators help you evaluate multiple conditions at once. This is important for writing complex decision-making logic in your code.
- Efficiency: Using operators effectively can lead to cleaner and more efficient code. Instead of writing complex functions, you can use operators to perform tasks in a straightforward manner.
By mastering operators in C#, you’ll be able to enhance your programming skills, making it easier to build dynamic and interactive applications. Stay tuned as we explore the different types of operators available in C# and how to use them effectively in your projects!
Types of Operators in C#
In C#, operators are categorized based on the operations they perform. Understanding these different types of operators is crucial for effective programming. Here’s an overview of the primary categories of operators you’ll encounter in C#:
Overview of the Different Types of Operators
- Arithmetic Operators
These operators perform basic mathematical operations. They include:- Addition (+): Adds two operands.
- Subtraction (-): Subtracts the second operand from the first.
- Multiplication (*): Multiplies two operands.
- Division (/): Divides the first operand by the second.
- Modulus (%): Returns the remainder of a division operation.
- Assignment Operators
Assignment operators are used to assign values to variables. The most common operator is:- Assignment (=): Assigns the right-hand operand to the left-hand variable.
- Additionally, there are compound assignment operators like +=, -=, *=, and /= that combine arithmetic operations with assignment.
- Comparison Operators
Comparison operators are used to compare two values. They return a boolean value (true or false). These include:- Equal to (==): Checks if two operands are equal.
- Not equal to (!=): Checks if two operands are not equal.
- Greater than (>): Checks if the left operand is greater than the right.
- Less than (<): Checks if the left operand is less than the right.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right.
- Logical Operators
Logical operators are used to combine multiple boolean expressions. They include:- Logical AND (&&): Returns true if both operands are true.
- Logical OR (||): Returns true if at least one operand is true.
- Logical NOT (!): Reverses the boolean value of the operand.
- Bitwise Operators
Bitwise operators perform operations on binary representations of integers. They include:- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Left Shift (<<)
- Right Shift (>>)
- Unary Operators
Unary operators operate on a single operand. They include:- Increment (++): Increases a variable’s value by 1.
- Decrement (–): Decreases a variable’s value by 1.
- Ternary Operator
The ternary operator (? :) is a shorthand for an if-else statement. It evaluates a condition and returns one of two values based on the evaluation. - Null Coalescing Operator
The null coalescing operator (??) returns the left operand if it is not null; otherwise, it returns the right operand.
Brief Explanation of Operator Categories
Each category of operators serves a specific purpose and is used in different scenarios within your C# code. By understanding the types of operators and their functionalities, you can write more efficient and effective programs. In the upcoming sections, we will explore each type of operator in detail, providing examples to illustrate how they work in practice.
Source code example
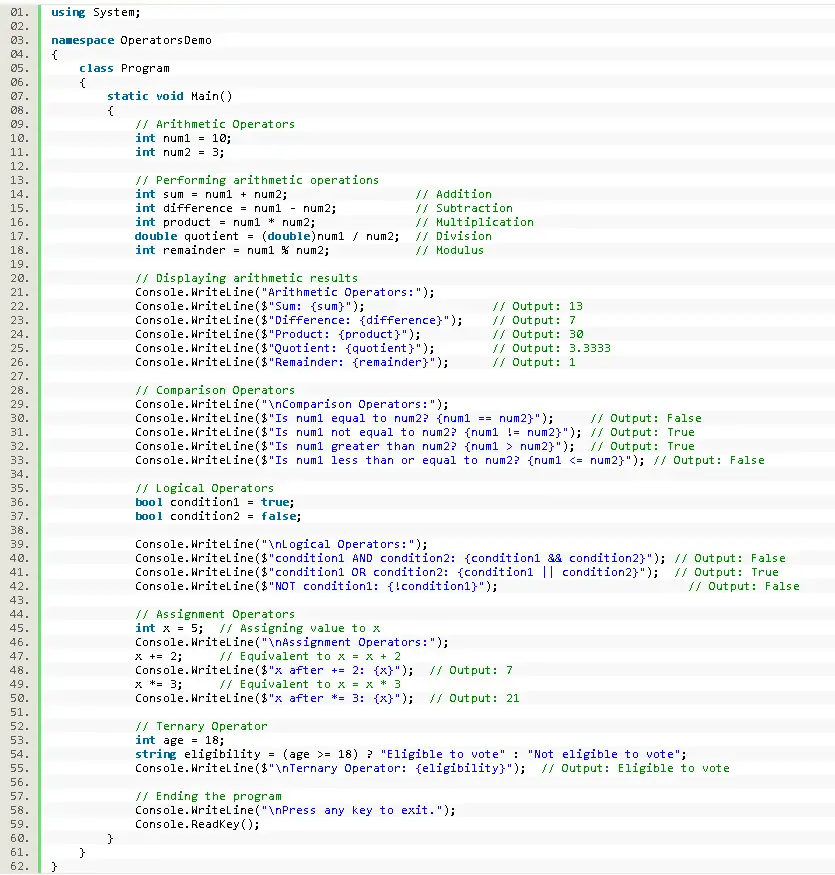
Here’s a beginner-friendly C# source code that demonstrates the use of various operators, including arithmetic, comparison, logical, and assignment operators. This code is structured to be simple and easy to understand for beginners.
using System; namespace OperatorsDemo { class Program { static void Main() { // Arithmetic Operators int num1 = 10; int num2 = 3; // Performing arithmetic operations int sum = num1 + num2; // Addition int difference = num1 - num2; // Subtraction int product = num1 * num2; // Multiplication double quotient = (double)num1 / num2; // Division int remainder = num1 % num2; // Modulus // Displaying arithmetic results Console.WriteLine("Arithmetic Operators:"); Console.WriteLine($"Sum: {sum}"); // Output: 13 Console.WriteLine($"Difference: {difference}"); // Output: 7 Console.WriteLine($"Product: {product}"); // Output: 30 Console.WriteLine($"Quotient: {quotient}"); // Output: 3.3333 Console.WriteLine($"Remainder: {remainder}"); // Output: 1 // Comparison Operators Console.WriteLine("\nComparison Operators:"); Console.WriteLine($"Is num1 equal to num2? {num1 == num2}"); // Output: False Console.WriteLine($"Is num1 not equal to num2? {num1 != num2}"); // Output: True Console.WriteLine($"Is num1 greater than num2? {num1 > num2}"); // Output: True Console.WriteLine($"Is num1 less than or equal to num2? {num1 <= num2}"); // Output: False // Logical Operators bool condition1 = true; bool condition2 = false; Console.WriteLine("\nLogical Operators:"); Console.WriteLine($"condition1 AND condition2: {condition1 && condition2}"); // Output: False Console.WriteLine($"condition1 OR condition2: {condition1 || condition2}"); // Output: True Console.WriteLine($"NOT condition1: {!condition1}"); // Output: False // Assignment Operators int x = 5; // Assigning value to x Console.WriteLine("\nAssignment Operators:"); x += 2; // Equivalent to x = x + 2 Console.WriteLine($"x after += 2: {x}"); // Output: 7 x *= 3; // Equivalent to x = x * 3 Console.WriteLine($"x after *= 3: {x}"); // Output: 21 // Ternary Operator int age = 18; string eligibility = (age >= 18) ? "Eligible to vote" : "Not eligible to vote"; Console.WriteLine($"\nTernary Operator: {eligibility}"); // Output: Eligible to vote // Ending the program Console.WriteLine("\nPress any key to exit."); Console.ReadKey(); } } }
Explanation of the Code:
- Arithmetic Operators: The program demonstrates addition, subtraction, multiplication, division, and modulus operations with two integer variables, num1 and num2.
- Comparison Operators: It compares num1 and num2 using various comparison operators and outputs the results as boolean values.
- Logical Operators: The program showcases how logical operators work with two boolean conditions, condition1 and condition2.
- Assignment Operators: It illustrates the use of assignment operators by modifying the value of x using the += and *= operators.
- Ternary Operator: This is a shorthand for an if-else statement that checks if age is greater than or equal to 18 to determine voting eligibility.
- Console Output: Each section prints the results of operations to the console, making it easy to see the effects of each operator.
This code provides a comprehensive introduction to operators in C# and is suitable for beginners to understand their usage.
Output
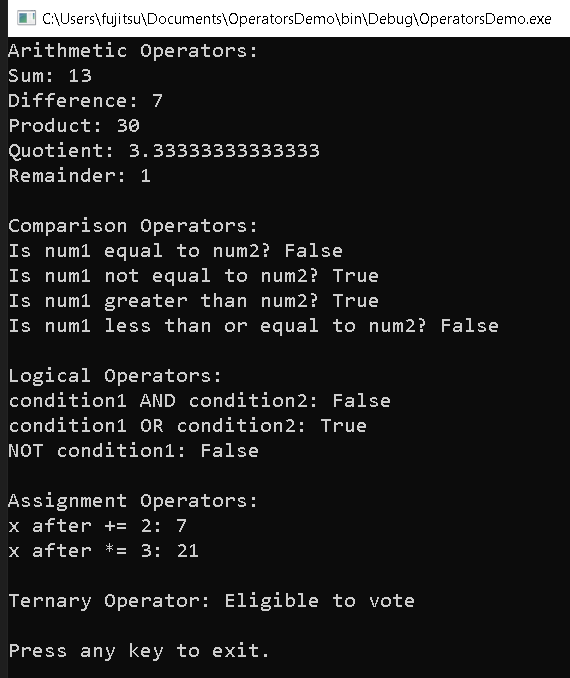
Conclusion
In this tutorial, we explored the essential concepts of arithmetic operators in C#. We learned that these operators are fundamental for performing mathematical calculations, including addition, subtraction, multiplication, division, and modulus. Here’s a recap of the key points we covered:
- Types of Arithmetic Operators: We discussed the basic operations and their syntax, along with practical examples demonstrating how to use them in C#.
- Operator Precedence: Understanding the order of operations is crucial for accurately calculating expressions in your code.
Practicing with these operators will enhance your ability to perform calculations and manipulate data effectively in your programs. We encourage you to experiment with different arithmetic operations and create your own examples to solidify your understanding.
As you continue your journey in C# programming, feel free to explore further topics such as other types of operators, conditional statements, loops, and data structures. Each of these areas will build upon the skills you’ve gained and open up new possibilities for your coding projects.
Thank you for watching, and happy coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.