Check If a String is a Palindrome in C#
Introduction
Table of Contents
A palindrome is a word, phrase, number, or sequence of characters that reads the same backward as it does forward. Common examples of palindromes include words like “madam” and “racecar,” or phrases like “A man, a plan, a canal, Panama.”
Checking if a string is a palindrome is a common task in programming, especially in areas like word games, puzzles, and string manipulations. Palindromes are used in algorithms, data validation, and even in coding challenges.
A key part of determining if a string is a palindrome involves ensuring that the check is case insensitive and ignores spaces or punctuation. For example, both “Racecar” and “A man, a plan, a canal, Panama” should be considered palindromes despite differences in case or punctuation.
In this tutorial, our goal is to build a C# program that checks if a given string is a palindrome. We will walk through taking user input, normalizing the string, reversing it, and then comparing the two versions to determine if they are the same.
Objectives
In this tutorial, we will focus on developing a deeper understanding of how to check if a string is a palindrome using C#. By the end of this lesson, you will have learned how to manipulate strings, normalize input, and implement logic to reverse and compare strings. This hands-on experience will help you practice coding techniques that can be applied to various real-world scenarios, such as word games or data validation.
Objectives:
- Understand the concept of palindromes and the process of checking them.
- Grasp the meaning of a palindrome and the importance of case insensitivity and ignoring spaces/punctuation when performing checks.
- Learn how to implement string manipulation in C#.
- Discover how to write a C# program that can take user input, normalize it (convert to lowercase, remove spaces), and reverse the string.
- Practice coding logic to identify palindromes.
- Work through examples to build a solution that compares a string with its reversed version, allowing you to identify palindromes efficiently.
- Apply the knowledge to real-world scenarios.
- Create a flexible palindrome checker in C# that can be adapted to handle words, phrases, and numbers, ignoring punctuation and spaces.
These objectives will guide you through the process of understanding palindrome checking, learning essential string manipulation skills, practicing coding logic, and applying this knowledge in practical applications.
Source code example
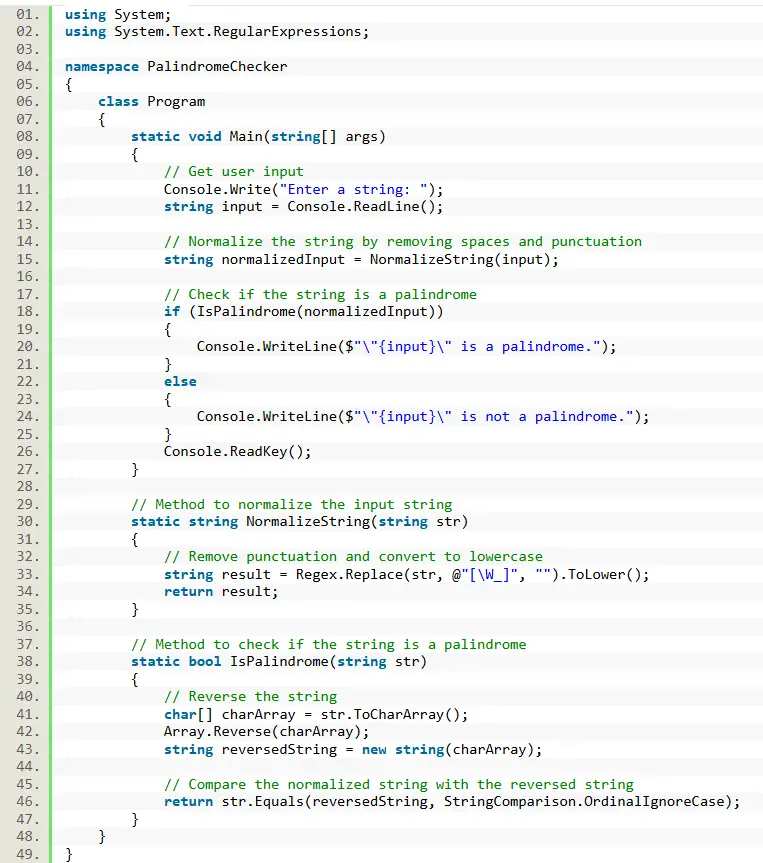
Explanation:
- User Input:
- The program prompts the user to enter a string. It reads the input using Console.ReadLine().
- Normalization:
- The NormalizeString method removes any non-alphanumeric characters (including spaces and punctuation) and converts the string to lowercase. It uses Regular Expressions (Regex) to handle this process efficiently.
- Palindrome Check:
- The IsPalindrome method checks if the normalized string reads the same backward. It reverses the string using an array and compares it with the original normalized string.
- Output:
- The program displays a message indicating whether the entered string is a palindrome or not.
Example Output
Enter a string: A man, a plan, a canal, Panama "A man, a plan, a canal, Panama" is a palindrome.
This code provides a straightforward implementation of a palindrome checker in C#, focusing on string manipulation and conditional logic, making it accessible for beginners.
Summary
In this lesson, we explored how to determine if a string is a palindrome using C#. We began by understanding the definition of a palindrome—words or phrases that read the same backward as forward—while also considering the importance of ignoring spaces, punctuation, and case differences. We developed a simple program that collects user input, normalizes the string by removing unwanted characters, and checks for palindromic properties through string reversal. By implementing the normalization and comparison logic, this tutorial provided valuable insights into string manipulation techniques in C#, reinforcing essential programming concepts for beginners.
Quiz
- Knowledge (Remembering)
What is a palindrome?
- A) A word that is spelled differently backward
- B) A word, phrase, or sequence that reads the same backward as forward
- C) A type of string manipulation
- D) A number that is the same when reversed
- Comprehension (Understanding)
Why is it important to normalize a string before checking for palindromes?
- A) To increase processing speed
- B) To ensure case sensitivity
- C) To ignore spaces and punctuation
- D) To make the string longer
- Application (Applying)
Which C# method would you use to reverse a string?
- A) string.Reverse()
- B) Array.Reverse()
- C) string.ToLower()
- D) string.Normalize()
- Analysis (Analyzing)
If the input string is “No ‘x’ in Nixon”, what would be the normalized string used for the palindrome check?
- A) “nixon”
- B) “noxinnixon”
- C) “noxinixon”
- D) “noxinixon”
- Evaluation (Evaluating)
Which approach is best for checking if a string is a palindrome in terms of performance and accuracy?
- A) Checking the string length first
- B) Comparing the original string to its reversed version after normalization
- C) Checking character by character without normalization
- D) Converting the string to an array and checking its length
Exercises and Assessment
To enhance your understanding of palindrome checking in C# and improve the existing source code, it’s important to practice and extend the program’s functionality. The following exercises, assessments, and lab exam are designed to challenge your skills by introducing additional features such as enhanced input validation, string manipulation, and more advanced palindrome checks. These tasks will help solidify your programming skills and prepare you for more complex scenarios involving string processing and data validation.
Exercises:
- Exercise 1: Case Sensitivity Check
- Modify the palindrome checker to be case-sensitive. Instead of ignoring case, display a message indicating whether the palindrome check is case-sensitive or not. For example, “Racecar” and “racecar” should not be considered the same.
- Exercise 2: Ignore Digits in Palindrome Check
- Update the program to ignore numeric digits while checking for palindromes. For instance, the input “12321” should be considered a palindrome, but “A12321A” should also be valid after removing digits.
- Exercise 3: Palindrome Substring Check
- Extend the program to find palindromic substrings within a given string. Ask the user for a string input and display all palindromic substrings found in that input.
- Exercise 4: Multiple Inputs in One Session
- Allow the user to check multiple strings for palindromic properties in one session. After each check, ask if they want to check another string and repeat the process until the user decides to exit.
- Exercise 5: Anagram Palindrome Check
- Create a function that checks if a given string can be rearranged to form a palindrome (e.g., “civic” and “ivicc”). Prompt the user for a string and display whether it can be rearranged to form a palindrome.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.