Calculate Shipping Cost Based on Weight in C#
Introduction
Table of Contents
In e-commerce and logistics, calculating shipping costs is a crucial part of delivering products to customers efficiently. Shipping costs are often determined based on factors like weight, distance, and the carrier used for delivery. Understanding how these costs are calculated helps businesses manage expenses and offer competitive pricing.
The primary factor affecting shipping cost is the package weight. Heavier packages cost more to ship due to higher handling and transportation fees. In this tutorial, we will build a C# program that calculates the shipping cost based on package weight.
Understanding Shipping Cost Calculation
Shipping costs are generally calculated using several variables. The most common structure involves a base cost for a minimum weight and additional costs for each kilogram beyond the base limit.
Sample Shipping Cost Structure:
- Base Cost: ₱50 for packages up to 1 kg.
- Additional Cost: ₱10 per kilogram for packages over 1 kg.
- Flat Rate: For packages above a certain weight threshold, a flat rate can be applied (e.g., ₱200 for packages over 20 kg).
By understanding this structure, you can build a program that dynamically calculates shipping costs based on the package weight, providing an efficient solution for e-commerce businesses.
In this tutorial, we’ll walk you through creating a C# program that implements this logic and gives the correct shipping cost based on user input.
Objectives
This tutorial will guide you through creating a C# program to calculate shipping costs based on package weight, a key concept in e-commerce and logistics. By the end of this lesson, you will not only understand how shipping costs are calculated but also learn how to implement these calculations in a C# program. Through hands-on practice, you will gain confidence in applying conditional logic and arithmetic operations to real-world scenarios.
- Understand how shipping costs are calculated based on package weight.
- Grasp the concept of base cost and additional costs per kilogram in determining shipping charges.
- Learn how to implement conditional logic and arithmetic calculations in C#.
- Discover how to write a program that calculates shipping costs dynamically based on weight input from the user.
- Practice coding and modifying the shipping cost calculator for different scenarios.
- Work through examples that calculate costs for light, heavy, and flat-rate packages.
- Apply the knowledge to create a flexible shipping cost calculator in C#.
- Build a program that can be adapted to various shipping cost structures used in e-commerce and logistics businesses.
These objectives will ensure that you not only understand the core concepts but also practice writing and applying code to solve real-world shipping cost problems.
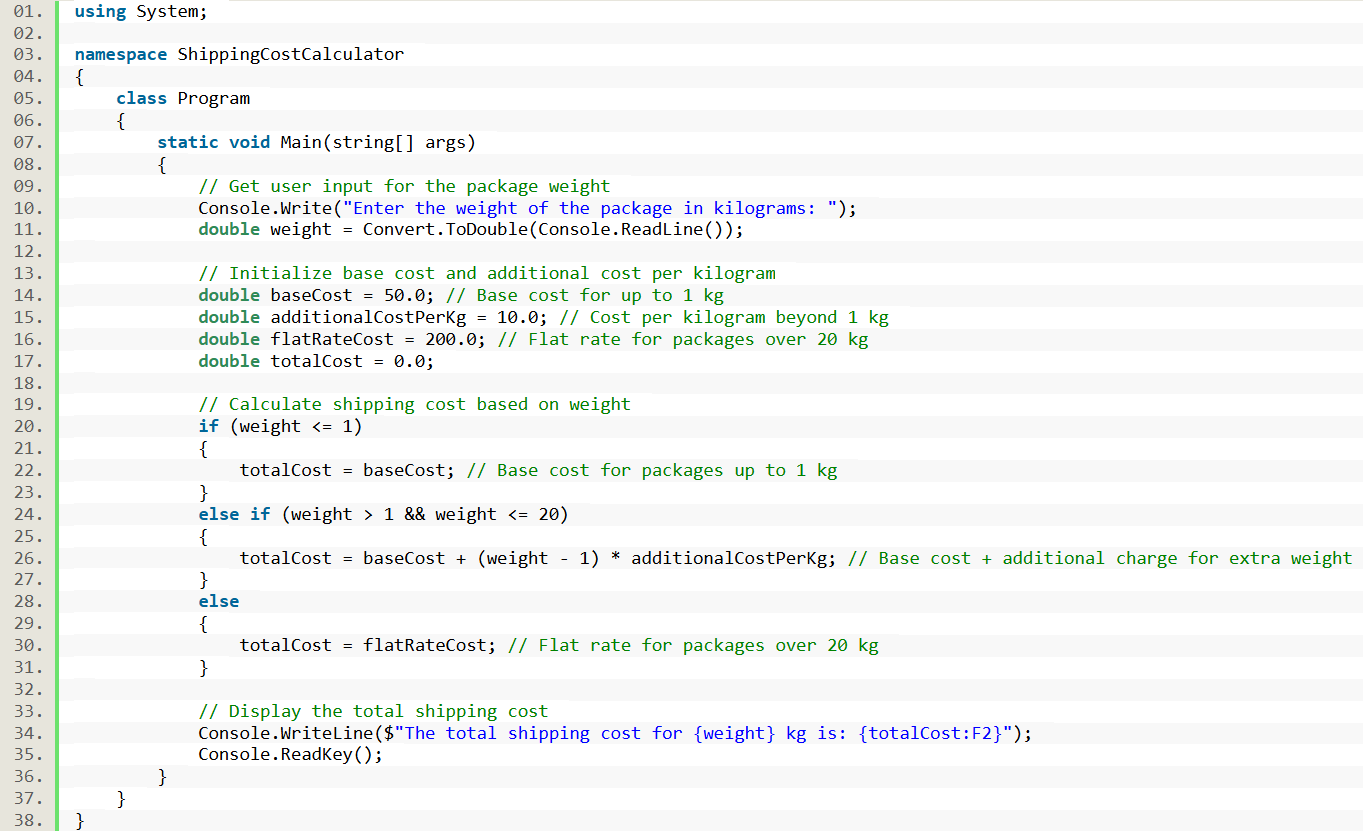
Source code example
using System; namespace ShippingCostCalculator { class Program { static void Main(string[] args) { // Get user input for the package weight Console.Write("Enter the weight of the package in kilograms: "); double weight = Convert.ToDouble(Console.ReadLine()); // Initialize base cost and additional cost per kilogram double baseCost = 50.0; // Base cost for up to 1 kg double additionalCostPerKg = 10.0; // Cost per kilogram beyond 1 kg double flatRateCost = 200.0; // Flat rate for packages over 20 kg double totalCost = 0.0; // Calculate shipping cost based on weight if (weight <= 1) { totalCost = baseCost; // Base cost for packages up to 1 kg } else if (weight > 1 && weight <= 20) { totalCost = baseCost + (weight - 1) * additionalCostPerKg; // Base cost + additional charge for extra weight } else { totalCost = flatRateCost; // Flat rate for packages over 20 kg } // Display the total shipping cost Console.WriteLine($"The total shipping cost for {weight} kg is: {totalCost:F2}"); Console.ReadKey(); } } }
Here’s a beginner-friendly C# source code that calculates the shipping cost based on package weight. This program uses simple conditional logic to determine the base cost and additional charges for packages over a certain weight.
Explanation
- User Input:
- The program prompts the user to input the weight of the package in kilograms, using Console.ReadLine() to capture the input and Convert.ToDouble() to convert it into a numerical value.
- Shipping Cost Calculation:
- If the weight is 1 kg or less, the base cost of ₱50 is applied.
- If the weight is between 1 kg and 20 kg, the total cost is the base cost plus an additional ₱10 per kilogram for the weight above 1 kg.
- If the weight exceeds 20 kg, a flat rate of ₱200 is applied.
- Output:
- The program then displays the calculated total shipping cost using Console.WriteLine().
Example Output
Enter the weight of the package in kilograms: 3.5 The total shipping cost for 3.5 kg is: ₱70.00
This code provides a simple, easy-to-understand solution for calculating shipping costs based on weight, using conditional logic to handle different pricing tiers. It’s a perfect example for beginners learning how to apply if-else statements and perform basic arithmetic operations in C#.
Summary
In this lesson, we learned how to create a C# program that calculates shipping costs based on package weight, a crucial aspect in logistics and e-commerce. The program applies conditional logic to determine the total cost based on weight ranges, using a base cost for packages up to 1 kg, an additional charge per kilogram for packages between 1 kg and 20 kg, and a flat rate for packages over 20 kg. By implementing user input, conditional statements, and arithmetic operations, this tutorial provides a beginner-friendly approach to solving real-world problems in shipping and logistics.
Quiz
- Knowledge (Remembering)
What is the base cost for packages up to 1 kg in the shipping cost program?
- A) ₱100
- B) ₱50
- C) ₱200
- D) ₱10
- Comprehension (Understanding)
Why is there an additional cost per kilogram after 1 kg in the shipping cost calculation?
- A) To reduce shipping cost for lighter packages.
- B) To account for increased transportation costs for heavier packages.
- C) To increase the overall base cost.
- D) To provide free shipping for heavier packages.
- Application (Applying)
Which C# structure is used to calculate the shipping cost based on different weight ranges?
- A) for loop
- B) switch statement
- C) if-else statements
- D) while loop
- Analysis (Analyzing)
If the weight of a package is 15 kg, which condition will the shipping cost program execute?
- A) Base cost only
- B) Flat rate for packages over 20 kg
- C) Base cost + additional cost for weight above 1 kg
- D) Free shipping
- Evaluation (Evaluating)
Which enhancement would be most useful for improving the current shipping cost program?
- A) Adding a time delay to the output
- B) Including options for different shipping speeds (Standard, Express)
- C) Removing conditional logic
- D) Reducing the base cost to ₱20
Exercises and Assessment
To further enhance your understanding of shipping cost calculations in C# and improve the existing code, it is important to apply new features and refine the logic. The following exercises, assessments, and lab exam are designed to challenge your skills and add more functionality to the program. By incorporating options like shipping speed, discounts, and handling multiple packages, you will strengthen your programming abilities and make the shipping cost calculator more adaptable for real-world scenarios.
Exercises:
- Exercise 1: Add Express Shipping Option
- Modify the program to include an option for express shipping, which charges 50% more than the base and additional rates. Ask the user to choose between standard or express shipping.
- Exercise 2: Discount for Heavy Packages
- Extend the program to apply a 10% discount for packages weighing over 10 kg. Calculate the total cost after applying the discount.
- Exercise 3: Free Shipping for Orders Over ₱500
- Update the program to offer free shipping for total costs that exceed ₱500. If the calculated shipping cost is over this amount, display a message indicating free shipping.
- Exercise 4: Handle Multiple Packages
- Allow the user to input the weight of multiple packages in one session. Calculate the total shipping cost for all packages combined, and display the individual cost for each package.
- Exercise 5: Validate User Input
- Add validation to ensure that the user inputs a valid weight. If the user enters a non-numeric or negative value, prompt them to re-enter the weight.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.