Calculate Fare Based on Distance Traveled
Introduction
In transportation systems, such as taxis, buses, and ride-sharing services, fares are often calculated based on the distance traveled. This ensures fair pricing for customers and helps service providers manage costs effectively. Understanding how to calculate fares based on distance is crucial for developing transportation apps or systems.
In this tutorial, we will guide you through creating a simple C# program that calculates fare based on the distance a passenger travels. The program will use a basic fare structure, including a base fare for a minimum distance and additional charges per kilometer beyond that. This is an excellent project for beginners to practice using conditional statements and arithmetic operations in C#.
Objectives
In this tutorial, we aim to help you develop a solid understanding of how fare calculations work in transportation systems, such as taxis and ride-sharing services, by building a simple C# program. Through this hands-on learning experience, you will not only understand the key concepts but also practice writing code that calculates fare based on distance traveled. By the end of this lesson, you will be able to apply these skills to real-world transportation and pricing systems.
- Understand the concept of fare calculation based on distance traveled.
- Grasp how transportation services like taxis and buses calculate fares using a base fare and additional cost per kilometer.
- Learn how to implement conditional logic and arithmetic operations in C#.
- Discover how to write a C# program that calculates fare based on distance input from the user.
- Practice writing and modifying code to handle different fare calculation scenarios.
- Work through examples that calculate fares for short and long distances, incorporating user input and conditional statements.
- Apply your knowledge to create a functional fare calculator in C#.
- Build a program that calculates fare based on distance traveled, with the flexibility to adapt it for various fare structures in transportation systems.
These objectives ensure that you gain a complete understanding of fare calculation, learn the necessary coding skills, practice using conditional logic, and apply this knowledge in real-world applications.
Source code example
Below are two versions of the fare calculator: one using if-else statements and another using a switch statement.
Version 1: Using if-else Statements
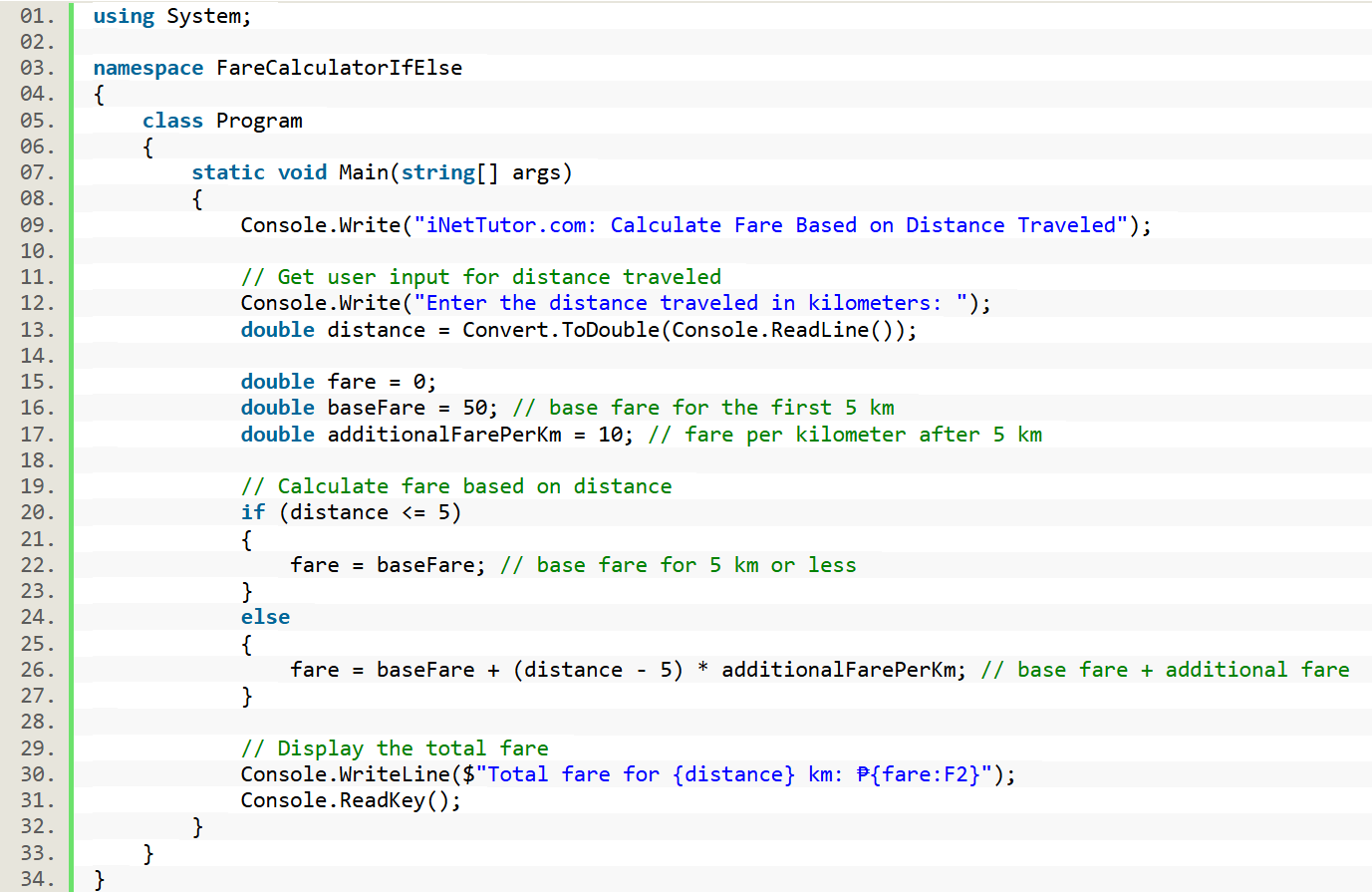
Explanation:
- The program asks the user for the distance traveled in kilometers.
- If the distance is 5 km or less, the base fare (₱50) is applied.
- If the distance is more than 5 km, the fare is calculated by adding an additional ₱10 per km for the extra distance.
- The total fare is then displayed.
Version 2: Using switch Statement
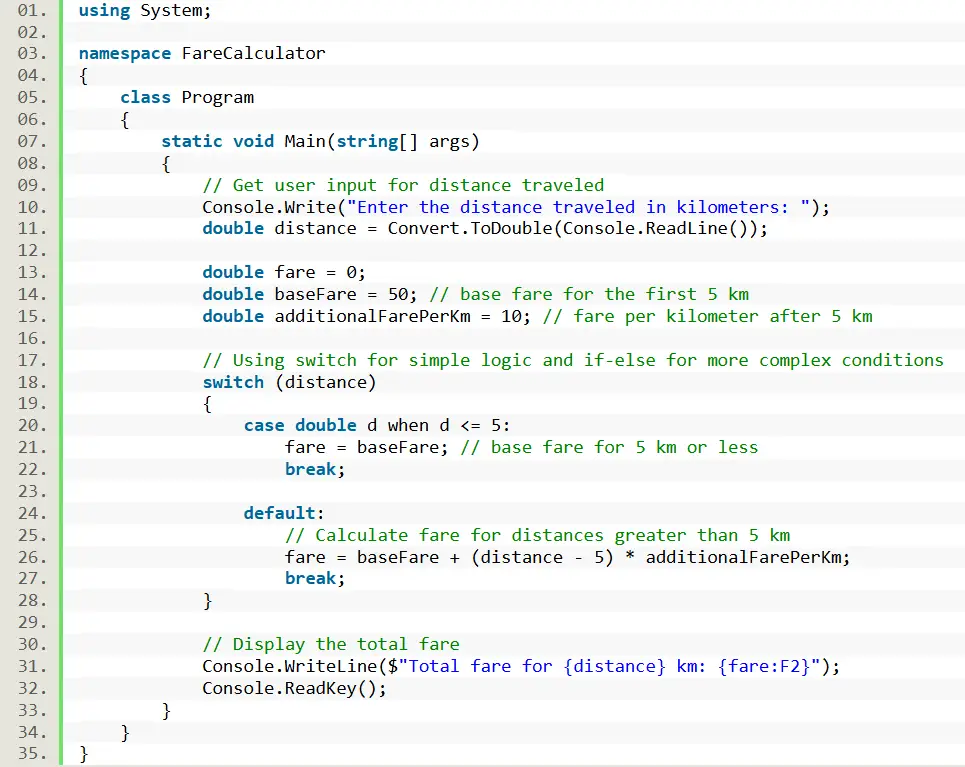
Explanation:
- case double d when d <= 5: This approach uses a guard clause with a when condition to handle distances less than or equal to 5 km in the switch statement. This is compatible with C# 7.3.
- For distances greater than 5 km, the program calculates the fare by adding the base fare plus the additional fare for the extra distance.
- The default case handles distances greater than 5 km, and the total fare is displayed.
The relational patterns feature, such as case <= 5, is available starting from C# 9.0. If you are using an earlier version of C# (such as C# 7.3), you will need to modify the switch statement to avoid using relational patterns. In C# 7.3, you can use a combination of a traditional switch statement and additional if-else logic to achieve similar behavior.
Output
Enter the distance traveled in kilometers: 8 Total fare for 8 km: 80.00
Key Points:
- User Input: The program collects the distance traveled from the user.
- Conditional Logic: It calculates the fare using if-else or switch statements depending on the distance.
- Fare Calculation: The base fare is applied for distances of 5 km or less, and an additional rate is charged for distances beyond that.
Both versions are simple, beginner-friendly, and demonstrate how to use conditional statements to solve real-world problems like fare calculation.
Summary
In this lesson, we learned how to create a fare calculator in C# that determines the total fare based on the distance traveled. By using both if-else and switch statements, we handled different fare structures, such as applying a base fare for the first 5 kilometers and charging an additional rate for distances beyond that. The tutorial walked through user input handling, conditional logic, and basic arithmetic operations, providing a practical example of how C# can be used for real-world applications like transportation fare calculations. This hands-on approach is ideal for beginners looking to apply programming concepts in everyday scenarios.
Exercises and Assessment
To deepen your understanding of fare calculation and improve the source code, it’s important to practice and extend the functionality of the program. The following exercises, assessment, and lab exam are designed to challenge your skills by introducing advanced features like discounts, surcharges, and additional validation. These tasks will enhance your ability to write robust and flexible C# code, preparing you for more complex scenarios.
Exercises:
- Exercise 1: Add a Nighttime Surcharge
- Modify the fare calculator to include a 20% nighttime surcharge for trips taken between 10 PM and 6 AM. Ask the user to enter the time of the trip and adjust the fare accordingly.
- Exercise 2: Apply a Discount for Students and Seniors
- Extend the program to ask the user if they are a student or senior. If yes, apply a 10% discount to the total fare.
- Exercise 3: Round Fare to the Nearest Whole Number
- Update the program to round the final fare to the nearest whole number, ensuring that the fare is always a round amount (e.g., ₱82.75 becomes ₱83).
- Exercise 4: Handle Multiple Trips in One Session
- Modify the program to calculate the fare for multiple trips. Ask the user if they want to calculate another fare and repeat the process until they choose to exit.
- Exercise 5: Calculate Total Fare for a Round Trip
- Add functionality that allows the user to calculate a round-trip fare (twice the one-way fare), with the option to provide different distances for the return trip.
Assessment:
- Assessment 1: Peak Hour Pricing
- Add a feature to handle peak hour pricing. Between 7 AM and 9 AM, and 5 PM to 8 PM, increase the fare by 30%. The user will input the time of the trip, and the program should adjust the fare accordingly.
- Assessment 2: Error Handling for Invalid Input
- Improve the program by adding error handling to prevent invalid input (e.g., non-numeric values, negative distances). If invalid input is detected, the program should display an error message and prompt the user to enter valid values.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.