Arithmetic Operators in C#
Introduction to Arithmetic Operators
Table of Contents
Arithmetic operators in C# are essential tools that allow you to perform basic mathematical operations like addition, subtraction, multiplication, division, and modulus. These operators are widely used to manipulate numerical data in programming and are fundamental for any developer.
What are Arithmetic Operators?
Arithmetic operators are symbols used in C# to perform calculations between variables or values. These include:
- Addition (+): Adds two numbers.
- Subtraction (-): Subtracts one number from another.
- Multiplication (*): Multiplies two numbers.
- Division (/): Divides one number by another.
- Modulus (%): Returns the remainder when one number is divided by another.
Importance of Arithmetic Operations in Programming
Arithmetic operations are crucial in programming because they enable developers to perform essential calculations that are required for tasks like:
- Performing real-time calculations (e.g., in finance or scientific applications).
- Handling user input and generating dynamic results.
- Building interactive applications like games, where scores or health points change.
Where Arithmetic Operations are Used in Everyday Applications
Arithmetic operations are a part of our daily lives, powering applications such as:
- Finance: Calculating interest rates, discounts, and total amounts.
- Gaming: Managing scores, levels, and in-game currency.
- Data Analysis: Summing up totals, finding averages, and analyzing datasets.
By mastering arithmetic operators, you’ll be able to create more dynamic, interactive, and data-driven applications in C#.
How Each Operator Works
In C#, arithmetic operators allow you to perform calculations on numbers. Let’s dive into how each operator works, along with code examples and practical usage scenarios.
- Addition (+)
The addition operator adds two numbers together. It’s commonly used to sum values in various contexts.
int total = 50 + 30; // total is 80
Practical Usage Example:
Calculating the total cost in shopping:
int item1 = 20; int item2 = 30; int totalCost = item1 + item2; // totalCost is 50
- Subtraction (-)
The subtraction operator subtracts one number from another.
int difference = 100 - 45; // difference is 55
Practical Usage Example:
Deducting expenses from a budget:
int budget = 500; int expenses = 200; int remainingBudget = budget - expenses; // remainingBudget is 300
- Multiplication (*)
The multiplication operator multiplies two numbers together.
int product = 8 * 7; // product is 56
Practical Usage Example:
Calculating interest over time or scaling up quantities in recipes:
double principal = 1000; double rate = 0.05; double interest = principal * rate; // interest is 50 (5% of 1000)
- Division (/)
The division operator divides one number by another. In C#, when both numbers are integers, the result is also an integer, discarding any remainder.
int result = 10 / 3; // result is 3 (integer division)
Handling Integer Division: If you need an accurate result with decimals, use double or float types.
double result = 10.0 / 3.0; // result is 3.3333
Practical Usage Example:
Splitting bills among friends:
int totalBill = 100; int people = 4; int amountPerPerson = totalBill / people; // amountPerPerson is 25
- Modulus (%)
The modulus operator returns the remainder when one number is divided by another.
int remainder = 10 % 3; // remainder is 1
Practical Usage Example:
Determining if a number is odd or even:
int number = 7; bool isEven = number % 2 == 0; // isEven is false (7 is odd)
Mastering these basic arithmetic operators in C# helps you build applications that can handle dynamic calculations and data manipulation.
Examples of Arithmetic Operations in C#
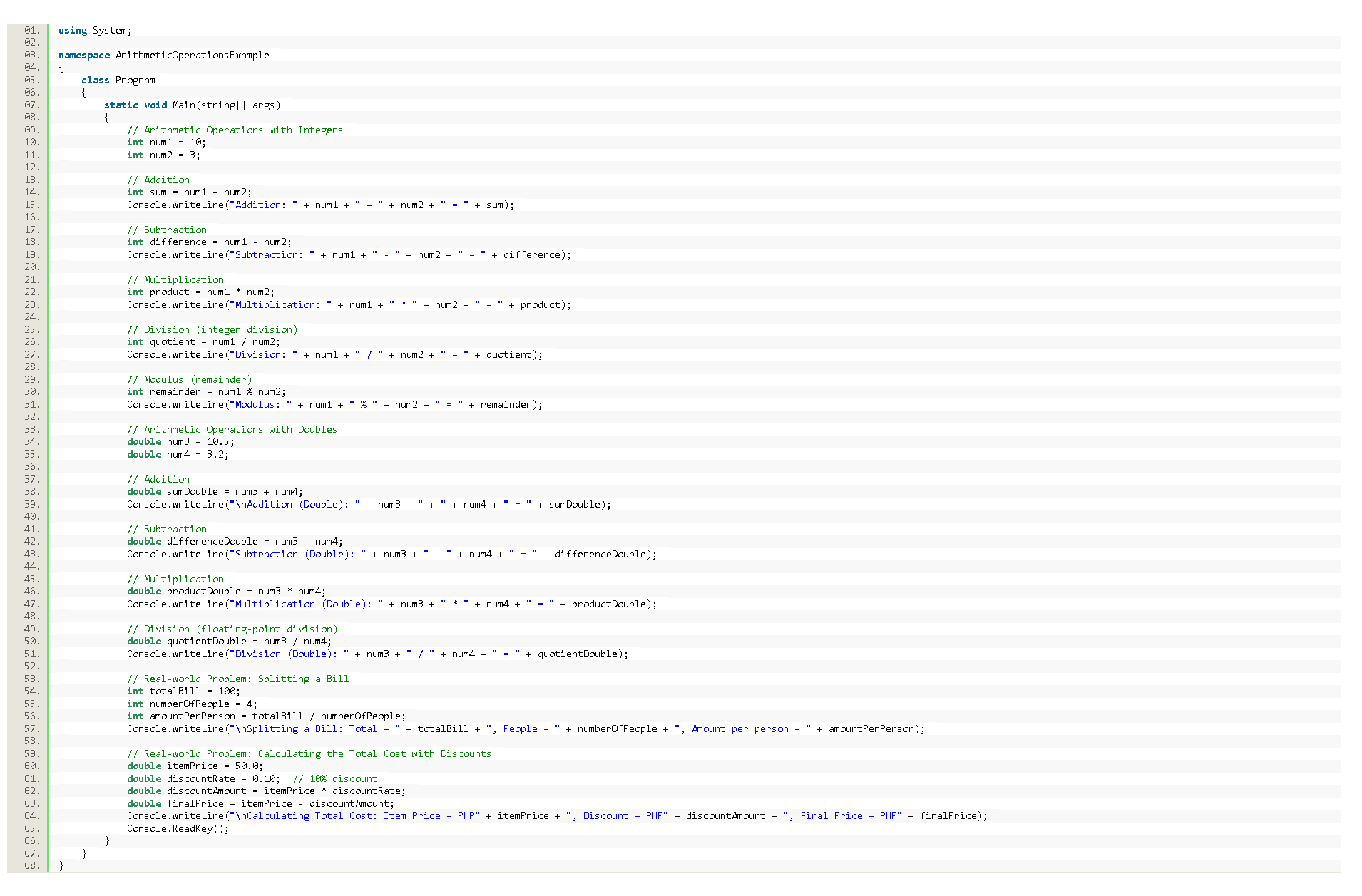
Here’s a simple and beginner-friendly C# source code that demonstrates the use of each arithmetic operator, including sample calculations with different data types, along with real-world problem examples.
using System; namespace ArithmeticOperationsExample { class Program { static void Main(string[] args) { // Arithmetic Operations with Integers int num1 = 10; int num2 = 3; // Addition int sum = num1 + num2; Console.WriteLine("Addition: " + num1 + " + " + num2 + " = " + sum); // Subtraction int difference = num1 - num2; Console.WriteLine("Subtraction: " + num1 + " - " + num2 + " = " + difference); // Multiplication int product = num1 * num2; Console.WriteLine("Multiplication: " + num1 + " * " + num2 + " = " + product); // Division (integer division) int quotient = num1 / num2; Console.WriteLine("Division: " + num1 + " / " + num2 + " = " + quotient); // Modulus (remainder) int remainder = num1 % num2; Console.WriteLine("Modulus: " + num1 + " % " + num2 + " = " + remainder); // Arithmetic Operations with Doubles double num3 = 10.5; double num4 = 3.2; // Addition double sumDouble = num3 + num4; Console.WriteLine("\nAddition (Double): " + num3 + " + " + num4 + " = " + sumDouble); // Subtraction double differenceDouble = num3 - num4; Console.WriteLine("Subtraction (Double): " + num3 + " - " + num4 + " = " + differenceDouble); // Multiplication double productDouble = num3 * num4; Console.WriteLine("Multiplication (Double): " + num3 + " * " + num4 + " = " + productDouble); // Division (floating-point division) double quotientDouble = num3 / num4; Console.WriteLine("Division (Double): " + num3 + " / " + num4 + " = " + quotientDouble); // Real-World Problem: Splitting a Bill int totalBill = 100; int numberOfPeople = 4; int amountPerPerson = totalBill / numberOfPeople; Console.WriteLine("\nSplitting a Bill: Total = " + totalBill + ", People = " + numberOfPeople + ", Amount per person = " + amountPerPerson); // Real-World Problem: Calculating the Total Cost with Discounts double itemPrice = 50.0; double discountRate = 0.10; // 10% discount double discountAmount = itemPrice * discountRate; double finalPrice = itemPrice - discountAmount; Console.WriteLine("\nCalculating Total Cost: Item Price = PHP" + itemPrice + ", Discount = PHP" + discountAmount + ", Final Price = PHP" + finalPrice); Console.ReadKey(); } } }
Explanation of the Code:
- Basic Arithmetic Operations with Integers:
- We declare two integer variables num1 and num2 and demonstrate addition, subtraction, multiplication, division, and modulus.
- Each result is displayed using Console.WriteLine().
- Arithmetic Operations with Doubles:
- We demonstrate the same arithmetic operations, but this time with double values (num3 and num4), which allows for more precise results, especially in division.
- Real-World Example 1: Splitting a Bill:
- We calculate how much each person pays when splitting a bill evenly.
- Real-World Example 2: Calculating Total Cost with Discounts:
- This example demonstrates how to calculate the final price of an item after applying a discount.
This simple source code is designed to give beginners a clear understanding of how arithmetic operators work with integers and floating-point numbers, as well as how these operations are applied to solve real-world problems.
Output
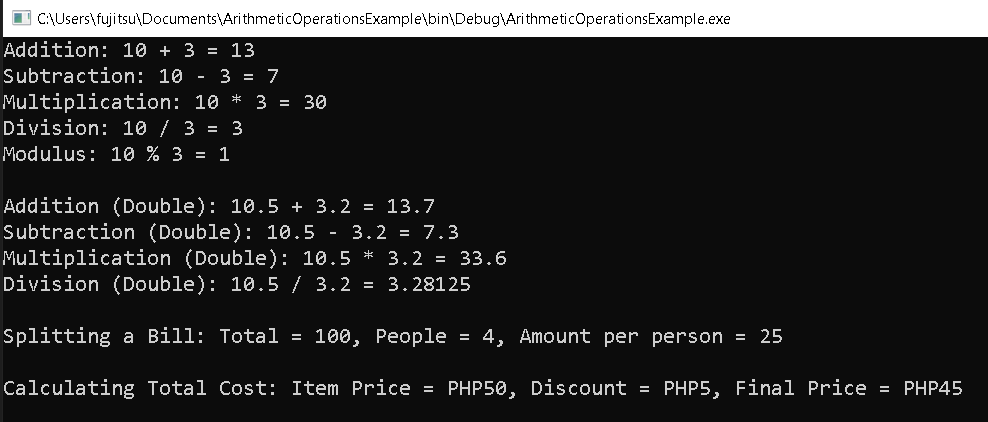
Conclusion
In this tutorial, we explored the basic arithmetic operators in C#—addition, subtraction, multiplication, division, and modulus. We demonstrated how these operators work with integers and floating-point numbers through simple examples and real-world scenarios like splitting bills and calculating discounts.
By mastering arithmetic operators, you’ll be able to perform essential calculations in your programs, whether it’s managing finances, analyzing data, or building interactive applications.
We encourage you to practice using these operators in real-world situations to strengthen your understanding. Try applying them to tasks like budgeting, calculating grades, or managing inventory. The more you practice, the more confident you’ll become in writing efficient and dynamic C# code!
Exercises
In order to reinforce your understanding of arithmetic operators in C#, it is essential to practice and apply these concepts through real-world examples. The following exercises, assessment, and lab exam will challenge you to enhance the source code, use arithmetic operations in different contexts, and solve practical problems. By completing these tasks, you will improve your ability to write efficient and dynamic C# programs that perform accurate calculations and data manipulations.
Exercise 1: Calculate Average Marks
- Write a program that takes the scores of 5 subjects as input from the user and calculates the total and average marks.
- Display the total and average on the console.
Exercise 2: Budget Tracker
- Write a program that tracks the user’s monthly budget. The user enters their monthly income and then enters the expenses for rent, groceries, transportation, and entertainment.
- Calculate and display the remaining balance after subtracting all expenses from the income.
Quiz
1. What is the symbol for the addition operator in C#?
A) –
B) *
C) +
D) /
2. What will be the output of the following C# code?
int a = 10;
int b = 4;
int result = a % b;
Console.WriteLine(result);
A) 2
B) 1
C) 4
D) 0
3. Which arithmetic operator would you use to calculate the total cost of two items in a shopping cart?
A) / (division)
B) * (multiplication)
C) + (addition)
D) % (modulus)
4. Given the code snippet below, which operator should be used to correctly calculate the average score of three students?
int score1 = 85;
int score2 = 90;
int score3 = 88;
int average = (score1 + score2 + score3) ___ 3;
A) %
B) /
C) *
D) +
5. Which statement correctly explains why using the modulus operator is useful when determining if a number is even or odd?
A) It divides two numbers and returns the quotient.
B) It adds two numbers together and checks if the result is even.
C) It divides two numbers and returns the remainder, which helps identify whether a number is divisible by 2.
D) It subtracts one number from another and checks for evenness.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.