Login System in VB.NET and SQL Server Tutorial and Source code
Problem
Create a Windows Form Application program in Visual Basic.Net that will allow users to create a login system with SQL Server.
Description
This tutorial will allow the user to create a Login System using VB.NET and SQL Server with 2 Text boxes and 2 command buttons.
Before the tutorial the following are required to start:
- Microsoft Visual Studio 2008 – Above
- Microsoft SQL Management Studio
The tutorial starts here:
- Using Microsoft SQL Management Studio, create a database with one table and 2 fields and name it Loginsystem.mdf
- Open Microsoft Visual Studio 2012
- Select a New Project on the File menu.
- Select Visual Basic, Windows Form Application then click OK.
- We need to design our form by the following controls:
- 2 Labels – labels for the Username Text box and Password text box
- 2 Text box – text boxes for the user name and password.
- 2 Command button – 1 button for Login and 1 button to cancel/close the form
- We will also name our form controls in this way:
- txtUsername is the name of the textbox for username
- txtPassword is the name of the textbox for Password
- cmdLogin is the name of the button for Login
- cmdCancel is the name of the button for Cancel
- This is how we design the form. (Feel free to layout your own)
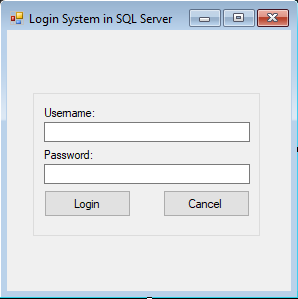
Figure 1. Design of the Form
- create a module and paste the following code:
Code here
Imports System.Data.SqlClient Module Connect_Database Public Conn As SqlConnection Public cmd As SqlCommand Public da As SqlDataAdapter Public dr As SqlDataReader Public dt As DataTable Public Sql As String Public Sub ConnecDatabase() Try Conn = New SqlConnection Conn.ConnectionString = "DATA SOURCE=(LOCAL);INITIAL CATALOG=LoginSystem;TRUSTED_CONNECTION=TRUE;INTEGRATED SECURITY=TRUE" Conn.Open() Catch ex As Exception End Try End Sub End Module
End Code
Code Explanation:
Codes for the connection between SQL Server and the system.
- Paste the code to import a service.
Code here
Imports System.Data.SqlClient
End Code
Code Explanation:
The code will connect the system to the SQL server as a client.
- Paste these codes to add a Sub for LoginSystem
Code here
Sub LoginSystem() If txtusername.Text = "" Then MsgBox("Username is Required!", MsgBoxStyle.Critical) txtusername.Focus() ElseIf txtpassword.Text = "" Then MsgBox("Password is Required!", MsgBoxStyle.Critical) txtpassword.Focus() Else Try ConnecDatabase() Sql = "Select * From tbl_accounts Where Username = @Username And Password = @Password;" cmd = New SqlCommand With cmd .Connection = Conn .CommandText = Sql .Parameters.Clear() .Parameters.AddWithValue("@Username", txtusername.Text) .Parameters.AddWithValue("@Password", txtpassword.Text) .ExecuteNonQuery() End With da = New SqlDataAdapter dt = New DataTable da.SelectCommand = cmd da.Fill(dt) Catch ex As SqlException MsgBox(ex.Message) Finally Conn.Close() da.Dispose() If dt.Rows.Count > 0 Then Dim Username, Password As String Username = dt.Rows(0).Item("Username") Password = dt.Rows(0).Item("Password") If txtusername.Text = Username And txtpassword.Text = Password Then MsgBox("Welcome " & txtusername.Text) txtusername.Text = "" txtpassword.Text = "" ElseIf txtusername.Text <> Username Then MsgBox("Username Doesn't Match!", MsgBoxStyle.Exclamation) txtusername.Text = "" txtusername.Focus() ElseIf txtpassword.Text <> Password Then MsgBox("Password Doesn't Match!", MsgBoxStyle.Exclamation) txtpassword.Text = "" txtpassword.Focus() Else MsgBox("Username or Password is Invalid!", MsgBoxStyle.Exclamation) txtpassword.Text = "" txtpassword.Focus() txtusername.Text = "" txtpassword.Text = "" End If End If End Try End If End Sub
End Code
Code Explanation:
The code will check the textboxes if there is a single character or not, once the textbox is blank, it will call the attention of the user, using a message box to fill the blank fields. Once the fields are filled, the system will go to the database then it will look for the username and password. If the username and password is matched, it will return a message box with a welcome with the username, else, it will return a return a message box that is either a the password or the username doesn’t match or the both username and password is invalid.
- Double click the login button then paste the following code.
Code here
LoginSystem()
End Code
Code Explanation:
This will call the sub LoginSystem mentioned earlier that will enable the user to login.
- Paste the following code to add a keydown event for the password text box.
Code here
Private Sub txtpassword_KeyDown(sender As Object, e As KeyEventArgs) Handles txtpassword.KeyDown If e.KeyCode = 13 Then LoginSystem() End If End Sub
End Code
Code Explanation:
The code will trigger if the user hits the Enter key on the Keyboard then it will login the user.
- Double click the cancel button then paste the following code:
Code here
Private Sub cmdcancel_Click(sender As Object, e As EventArgs) Handles cmdcancel.Click Me.Dispose() End Sub
End Code
Code Explanation:
The code will clear the textboxes and exits the form.
Conclusion:
Login is one of the basic security feature that must be included in any database driven information system. With this tutorial, you will be able to create a login form that connects to a database server and make your application secure.
Author:
Name: Charlie Devera
Email Address: charliedevera000@gmail.com
Free Download Source code (Login System in VB.NET and SQL Server)
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.